ResourceBundle Class In Java
ResourceBundle Class
In Java, The ResourceBundle class is mainly used to internationalize the messages. We can say that it provides a mechanism to globalize the messages.
In terms of programming, the hardcoded message is not considered good because it’s different from one country to another. Thus, we use the ResourceBundle class to globalize the messages. This class loads the information’s from the properties file, which holds the messages.
The name of the properties file must be filename_languagecode_country code.
For example
Message_en_US.properties.
Methods of ResourceBundle class
public static ResourceBundle getBundle(String basename)
This method is used to return the object of the ResourceBundle class for the default locale.
public static ResourceBundle getBundle(String basename, Locale locale)
This method is used to return the object of the ResourceBundle class for the particular locale.
public String getString(String key)
This method is used to return the value for the corresponding key from the resource bundle.
Let's see an example of ResourceBundle class, given below.
MessageBundle_en_US.properties file holds the localize message for US country.
MessageBundle_in_ID.properties file holds the localize message for Indonesia country.
ResourceBundleExample.java file, which loads the properties file in a bundle and prints the messages.
MessageBundle_en_US.properties
- Message = how are you?
MessageBundle_in_ID.properties
- Message = apa kabar?
ResourceBundleExample.java
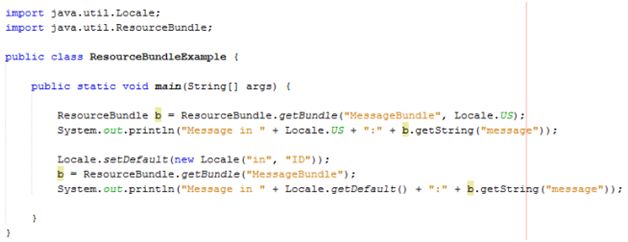
- import java.util.Locale;
- import java.util.ResourceBundle;
- public class ResourceBundleExample {
- public static void main(String[] args) {
- ResourceBundle b = ResourceBundle.getBundle("MessageBundle", Locale.US);
- System.out.println("Message in " + Locale.US + ":" + b.getString("message"));
- Locale.setDefault(new Locale("in", "ID"));
- b = ResourceBundle.getBundle("MessageBundle");
- System.out.println("Message in " + Locale.getDefault() + ":" + b.getString("message"));
- }
- }
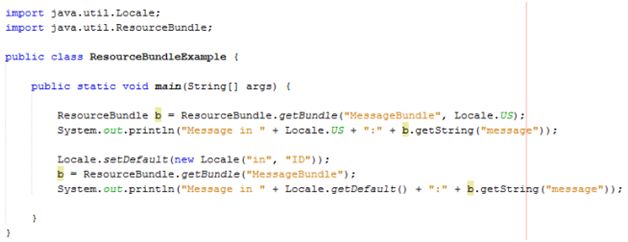
Summary
Thus, we learnt that the ResourceBundle class is mainly used to internationalize the messages and also learnt how to use it in Java.