Thread Pool In Java
Thread Pool
Thread pool is a group of worker threads, which are waiting for the job and it can be reused many times.
A group of fixed size threads are created in a thread pool. A thread from the thread pool is selected and is assigned a job by the Service provider.After completion his job, thread is contained in the thread pool again.
Advantage of Thread Pool
Its main advantage is it saves time and better performance because there is no need to create new thread.
Usage of Thread Pool
It is mainly used in Servlet and JSP in which Web or Servlet container creates a thread pool to process the request.
Let’s see an example, given below.
Code
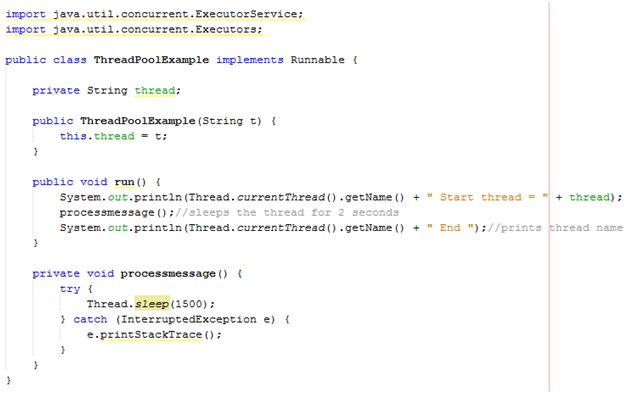
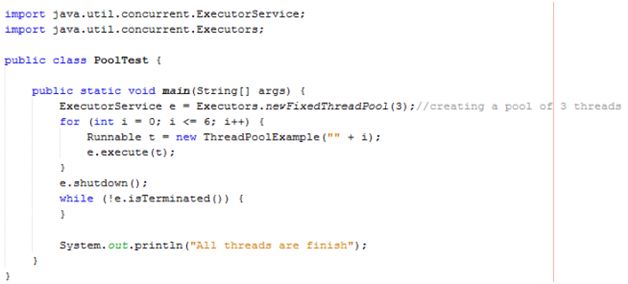
- import java.util.concurrent.ExecutorService;
- import java.util.concurrent.Executors;
- public class ThreadPoolExample implements Runnable {
- private String thread;
- public ThreadPoolExample(String t) {
- this.thread = t;
- }
- public void run() {
- System.out.println(Thread.currentThread().getName() + " Start thread = " + thread);
- processmessage(); //sleeps the thread for 2 seconds
- System.out.println(Thread.currentThread().getName() + " End "); //prints thread name
- }
- private void processmessage() {
- try {
- Thread.sleep(1500);
- } catch (InterruptedException e) {
- e.printStackTrace();
- }
- }
- }
- import java.util.concurrent.ExecutorService;
- import java.util.concurrent.Executors;
- public class PoolTest {
- public static void main(String[] args) {
- ExecutorService e = Executors.newFixedThreadPool(3); //creating a pool of 3 threads
- for (int i = 0; i <= 6; i++) {
- Runnable t = new ThreadPoolExample("" + i);
- e.execute(t);
- }
- e.shutdown();
- while (!e.isTerminated()) {}
- System.out.println("All threads are finish");
- }
- }
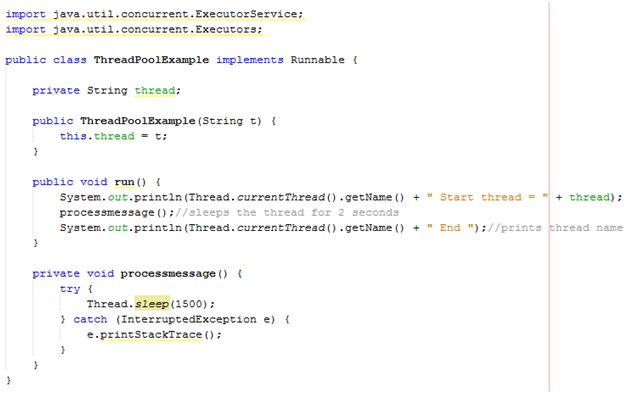
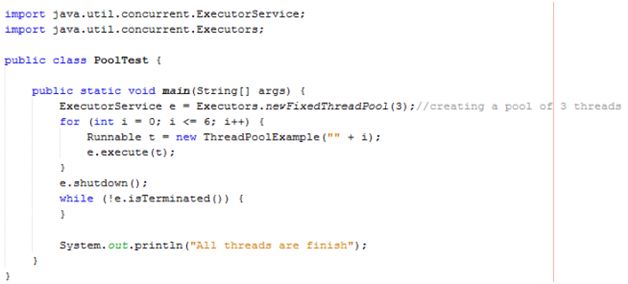
Output
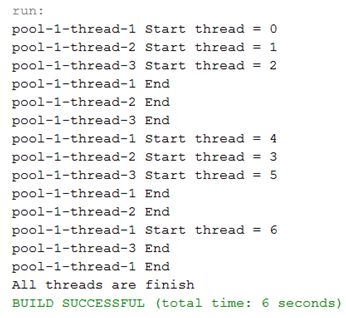
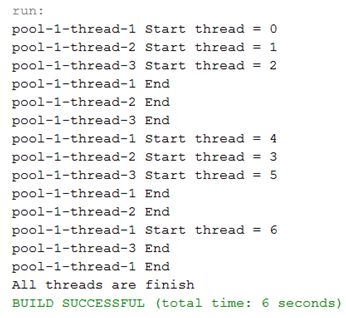
Summary
Thus, we learnt, thread pool is a group of worker threads, which are waiting for the job and it can be reused many times and also learnt, how to create it in Java.