Immutable String In Java
Immutable String
String objects are immutable in Java. Immutable simply means unchangeable, which means that the value stored in the object cannot be changed and when we perform any operations such as concat or replace, internally a new object is created to hold the result.
In other words, once the string object is created in Java, its data or state can't be changed but a new string object is created.
Let's see a simple example, given below.
Code
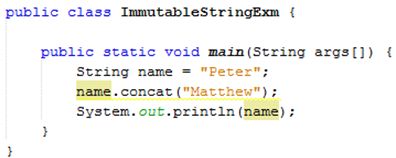
- public class ImmutableStringExm {
- public static void main(String args[]) {
- String name = "Peter";
- name.concat("Matthew");
- System.out.println(name);
- }
- }
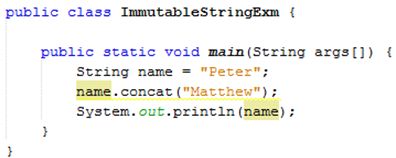
Output


Let’s understand with fig.
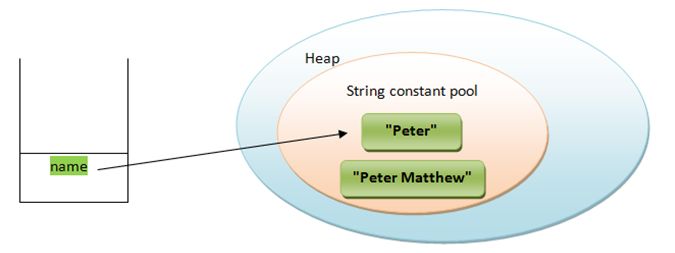
In the figure, shown above, two objects are created but the name reference variable still refers to "Peter" and not to "Peter Matthew". Since Peter is not changed but a new object is created with Peter Matthew and due to this, the string is known as immutable.
When we explicitly assign it to the reference variable, it will refer to “Peter Matthew" object.
Let’s see an example, given below.
Code
- public class ImmutableStringExm {
- public static void main(String args[]) {
- String name = "Peter";
- name = name.concat(" Matthew");
- System.out.println(name);
- }
- }
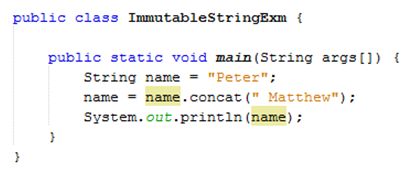
Output


In the example, shown above, name points to the “Peter Matthew” and still Peter object is not modified.
Let’s see another example, given below.
Code
- public class ImmutableStringExm {
- public static void main(String args[]) {
- String s1 = "Let’s test";
- s1.concat(" String is IMMUTABLE");
- System.out.println(s1);
- s1 = s1.concat(" String is IMMUTABLE");
- System.out.println(s1);
- }
- }
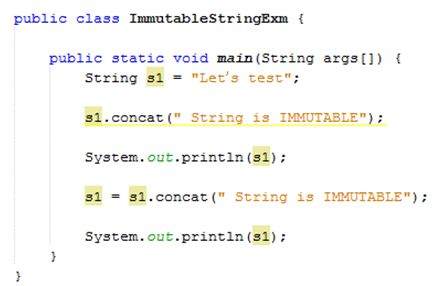
Output
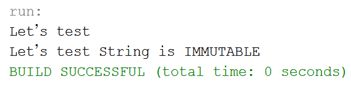
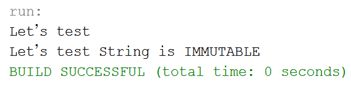
String object is immutable in Java because Java uses the concept of string literal. Suppose, there are six or seven reference variables and all reference variables refers to one object "Peter". If one reference variable changes the value of the object then it will be affected to all the reference variables. That’s why string objects are immutable in Java.
Summary
Thus, we learnt that immutable string simply means that the value stored in the object cannot be changed in Java and also learnt how to create immutable string in Java.