Object Class In Java
Object class
The object class is like a root of the class hierarchy. In Java, it is the topmost class. By default, Object class is the parent class of all the classes which means that every class has an object as a parent class. All objects, including arrays, implement the methods of this class.
The Object class is useful, if we want to refer any object whose type we don't know.
Let's see an example, given below.
getObject() is a method that returns an object but it can be of any type like car, person etc. We can use Object class reference to refer that object.
For example
For example
- Object obj=getObject();
- //We doesn’t know what object would be returned from this method.
The Object class provides various general behaviors to all the objects such as object can be compared, cloned and notified etc.
Class declaration
class declaration for java.lang.Object class.


Class constructors
class constructor for java.lang.Object class.
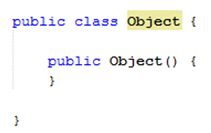
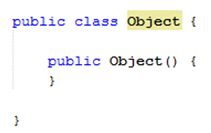
Methods of Object class
The object class provides various methods are,
public final ClassgetClass()
This method is mainly used to return the class object of this object.
public int hashCode()
This method is used to return the hashcode value for this object.
public boolean equals(Object obj)
This method is used to compare the specified object with this object.
protected Object clone() throws CloneNotSupportedException
This method is used to create and return the exact copy of this object.
public String toString()
This method is used to return the string representation of this object.
public final void notify()
This method is used to wakes up single thread and waiting on this object's monitor.
public final void notifyAll()
This method is used to wake up all the threads and waiting on this object's monitor.
public final void wait(long timeout)throws InterruptedException
This method is leads the current thread to wait for the specified milliseconds, until another thread notifies.
public final void wait(long timeout,int nanos)throws InterruptedException
This method leads to the current thread to wait for the specified miliseconds and nanoseconds, until another thread notifies.
public final void wait()throws InterruptedException
This method is used to causes the current thread to wait, until another thread notifies.
protected void finalize()throws Throwable
This method is used to invoke by the garbage collector before an object is being garbage collected.
Summary
Thus, we learnt, that an Object class is useful, if we want to refer any object whose type we don't know and also learnt its important methods.