Encapsulation In Java
Encapsulation
In Java, encapsulation is a concept of OOPS. It is a procedure of wrapping the code and data together into a single unit, for example tea is a mixture of several items.
Encapsulation is a process to bind related methods and variables in a protective wrapper (Class) with necessary access modifiers .With the use of encapsulation, the code can be secured from an unauthorized access by others and is easy to maintain.
We can create a fully encapsulated class by creating all the data members of the class as private. Java provides setter and getter methods to set and get the data in it. Example of fully encapsulated class is a Java bean class.
Advantages of Encapsulation are,
- It provides the control over the data. We can write the logic inside the setter method.
- We can make our class read only or write only with the use of setter and getter method.
- It makes the code more secure, flexible and maintainable.
- In encapsulation, we can change one part of the code easily without affecting the other part of the code.
Let’s see an example, given below.
Code
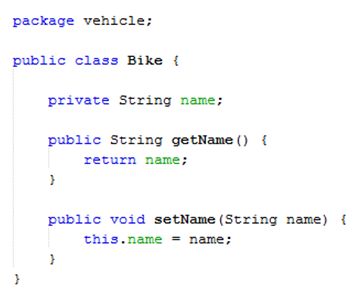
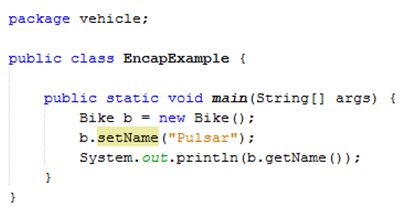
- package vehicle;
- public class Bike {
- private String name;
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- }
- package vehicle;
- public class EncapExample {
- public static void main(String[] args) {
- Bike b = new Bike();
- b.setName("Pulsar");
- System.out.println(b.getName());
- }
- }
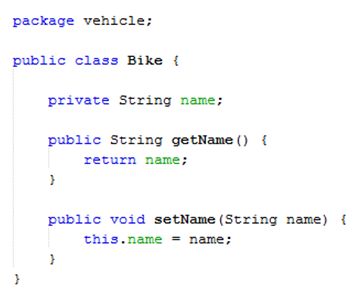
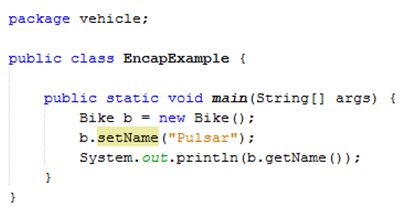
Output


In the example of encapsulation program, shown above, has only one data member with its setter and getter methods.
Summary
Thus, we learnt that encapsulation is a process to bind the related methods and variables in a protective wrapper (Class) with the necessary access modifiers and also learnt its advantages in Java.