Introduction Of Swing In Java
Swing
In Java, Swing is a part of Java Foundation Classes (JFC), which is used to create Window-based Applications. It is built on the top of AWT API and fully written in Java. Swing provides the platform-independent and lightweight components.
The javax.swing package gives many classes for swing API such as JButton, JTextField, JRadioButton, JCheckbox, JColorChooser etc.
Difference between AWT and Swing
AWT | Swing |
AWT components are platform-dependent. | Swing components are platform-independent. |
Its components are heavyweight. | Its components are lightweight. |
AWT doesn't support pluggable look and feel. | Swing supports pluggable look and feel. |
AWT gives less components than Swing. | Swing gives more powerful components than AWT. |
AWT doesn't follow MVC (Model View Controller). | Swing follows MVC (Model View Controller). |
Java Foundation Classes (JFC) is a set of GUI components, which makes it simple for the development of desktop Applications.
Hierarchy of Swing classes
The hierarchy of Swing API is given below.
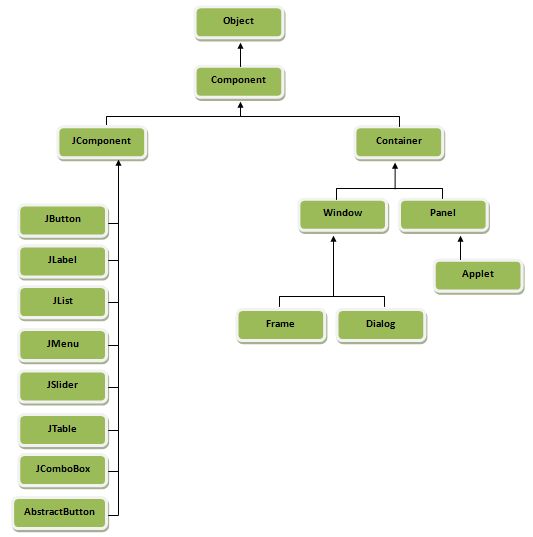
Methods of Component class
The methods of Component class are generally used in Swing, which are,
public void add(Component c)
This method is used to add a component on another component.
public void setSize(int width,int height)
This method is used to set the size of the component.
public void setLayout(LayoutManager m)
This method is used to set the layout manager for the component.
public void setVisible(boolean b)
This method is used to set the visibility of the component. It is by default false.
In Swing, there are 2 ways to create a frame, which are,
- By creating the object of Frame class (association)
- By extending Frame class (inheritance)
In Swing, we can write the code inside the main(), constructor or any other method.
Let’s see an example of Swing, given below.
Code
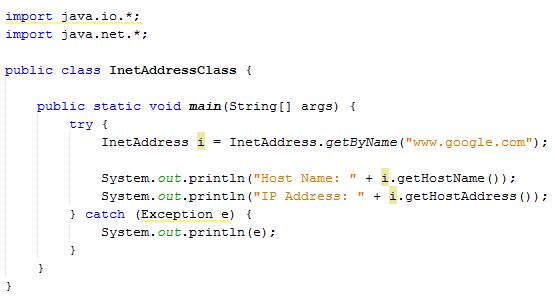
Output
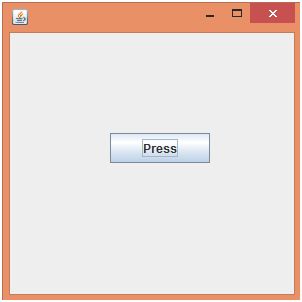
- import javax.swing.*;
- public class SwingExample {
- public static void main(String[] args) {
- JFrame jf = new JFrame(); //create object of JFrame
- JButton jb = new JButton("Press"); //create object of JButton
- jb.setBounds(100, 100, 100, 30);
- jf.add(jb); //add button in JFrame
- jf.setSize(300, 300); // width and height
- jf.setLayout(null);
- jf.setVisible(true);
- }
- }
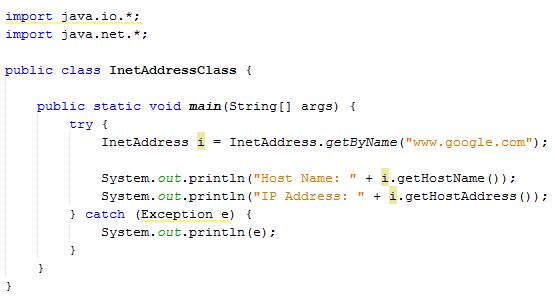
Output
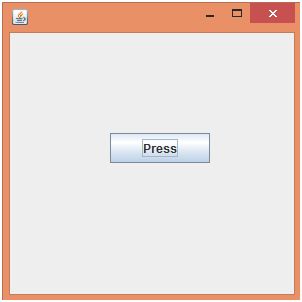
In the example, shown above, we create one button and add it on the JFrame object inside the main() method.
Let’s see an example of Swing through Association inside the constructor.
Code
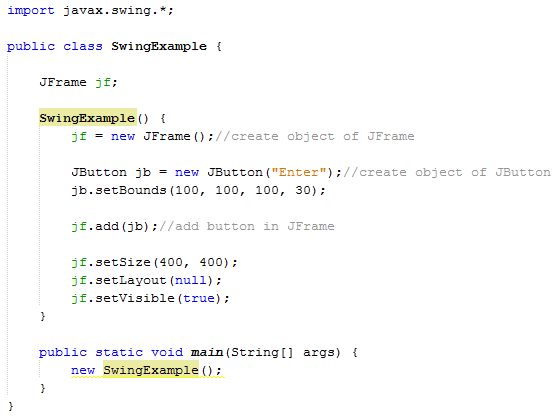
- import javax.swing.*;
- public class SwingExample {
- JFrame jf;
- SwingExample() {
- jf = new JFrame(); //create object of JFrame
- JButton jb = new JButton("Enter"); //create object of JButton
- jb.setBounds(100, 100, 100, 30);
- jf.add(jb); //add button in JFrame
- jf.setSize(400, 400);
- jf.setLayout(null);
- jf.setVisible(true);
- }
- public static void main(String[] args) {
- new SwingExample();
- }
- }
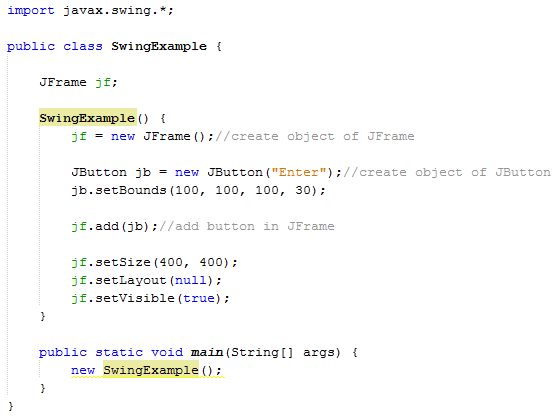
Output
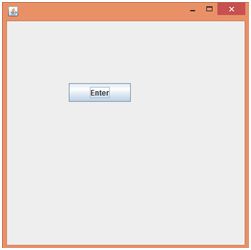
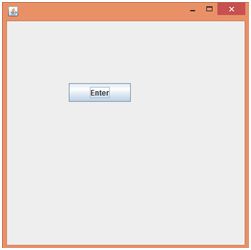
In the example, shown above, the setBounds(int xaxis, int yaxis, int width, int height) are used to set the position of the button. We can also write all the codes to create JFrame, JButton and the method is called inside Java constructor.
Let’s see an example of Swing through an inheritance.
Code
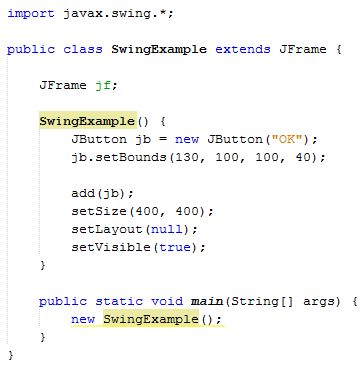
- import javax.swing.*;
- public class SwingExample extends JFrame {
- JFrame jf;
- SwingExample() {
- JButton jb = new JButton("OK");
- jb.setBounds(130, 100, 100, 40);
- add(jb);
- setSize(400, 400);
- setLayout(null);
- setVisible(true);
- }
- public static void main(String[] args) {
- new SwingExample();
- }
- }
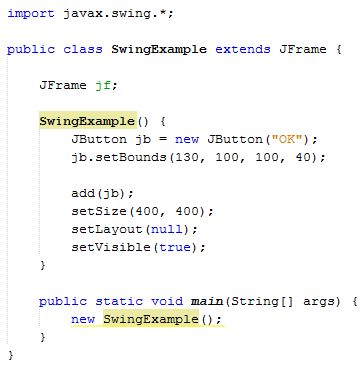
Output
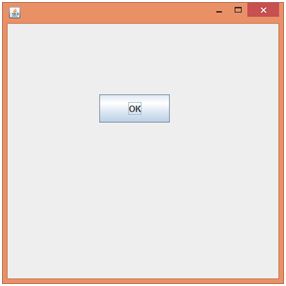
We can also inherit JFrame class. Thus, there is no need to create the object of JFrame class explicitly.
Summary
Thus, we learnt that Java Swing is a part of Java Foundation Classes (JFC), which is used to create Window-based Applications and also learnt how we can use it in Java.