Aggregation In Java
Aggregation
In Java, aggregation represents “has-a” relationship between two classes and it is a special form of association. If a class has an entity reference, it is called as an aggregation. This helps to reduce duplication of the code and errors.
Aggregation is mainly used for the code reusability.
Let’s see an example, given below.
Code
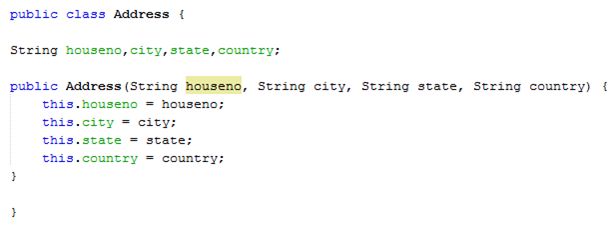
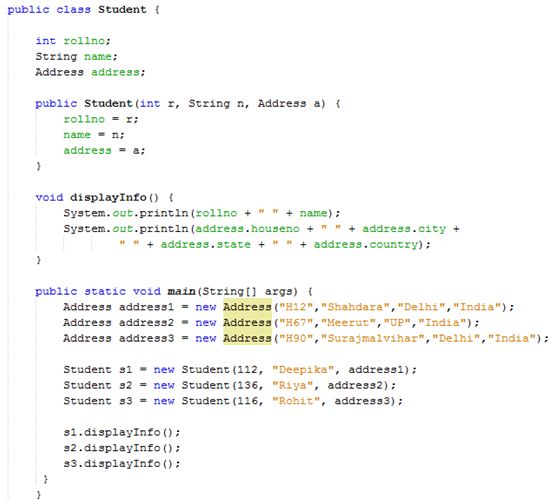
- public class Address {
- String houseno, city, state, country;
- public Address(String houseno, String city, String state, String country) {
- this.houseno = houseno;
- this.city = city;
- this.state = state;
- this.country = country;
- }
- }
- public class Student {
- int rollno;
- String name;
- Address address;
- public Student(int r, String n, Address a) {
- rollno = r;
- name = n;
- address = a;
- }
- void displayInfo() {
- System.out.println(rollno + " " + name);
- System.out.println(address.houseno + " " + address.city + " " + address.state + " " + address.country);
- }
- public static void main(String[] args) {
- Address address1 = new Address("H12", "Shahdara", "Delhi", "India");
- Address address2 = new Address("H67", "Meerut", "UP", "India");
- Address address3 = new Address("H90", "Surajmal vihar", "Delhi", "India");
- Student s1 = new Student(112, "Deepika", address1);
- Student s2 = new Student(136, "Riya", address2);
- Student s3 = new Student(116, "Rohit", address3);
- s1.displayInfo();
- s2.displayInfo();
- s3.displayInfo();
- }
- }
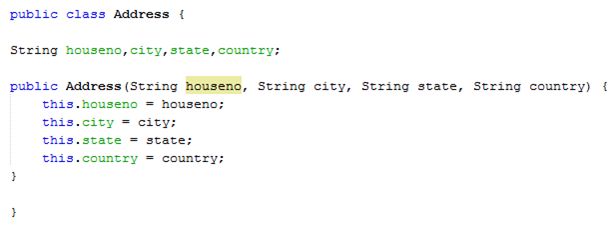
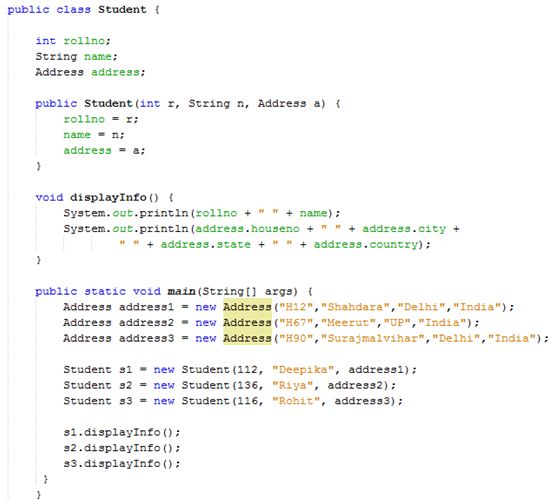
Output
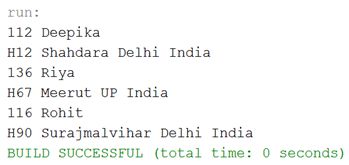
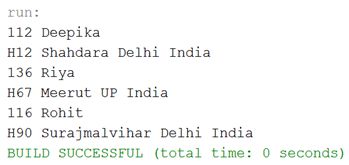
In the example, mentioned above, we create two classes Address class and Student class. Suppose, there are many students in college and each student must have a different address. Hence, the relationship between student and address is “Has-A” relationship that shows the aggregation between them.
Aggregation main advantage is code reusability and with the usage of aggregation, we can modify the complex code to a simple form and we can easily detect the errors in aggregation.
Why we use Aggregation in Java?
In Java, aggregation is used to maintain code in simple form. It is required in order to make and code reusable. We don’t need to write same code again and again, which is already explained in the example, mentioned above. In order to maintain Student’s address, we just have to use the reference of Address class as defining each of these classes. Hence, we can improve the code reusability, using aggregation relationship. Aggregation is a best choice, when there is no IS-A relationship.
Summary
Thus, we learnt that aggregation represents “has-a” relationship between two classes. It is a special form of association and we also learnt its usage in Java.