ExceptionHandling With MethodOverriding In Java
Exception handling with Methodoverriding
Key points of exception handling with Methodoverriding are,
- In Java, if the parent class method does not declare an exception, the child class overridden method cannot declare the checked exception but child class overridden method can declare an unchecked exception.
- In Java, if the parent class method declares an exception, the child class overridden method can declare same, child class exception or no exception but cannot declare a parent class exception.
When the parent class method does not declare an exception
Let’s see an example, given below.
Code
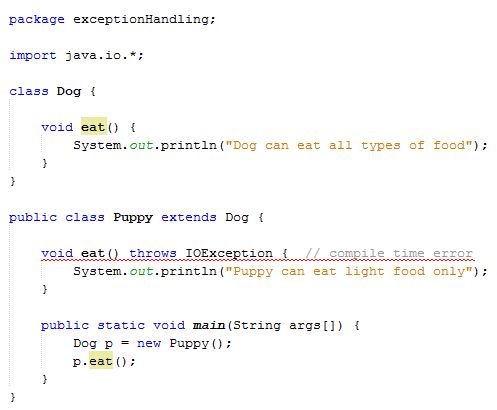
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() throws IOException { // compile time error
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- p.eat();
- }
- }
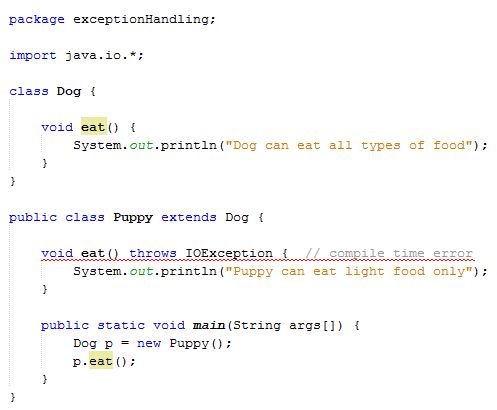
In the example, shown above, Dog (parent) class method does not declare an exception but Puppy (child) class overridden method cannot declare the checked exception in Java.
Let’s see another example, given below.
Code
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() throws ArithmeticException {
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- p.eat();
- }
- }
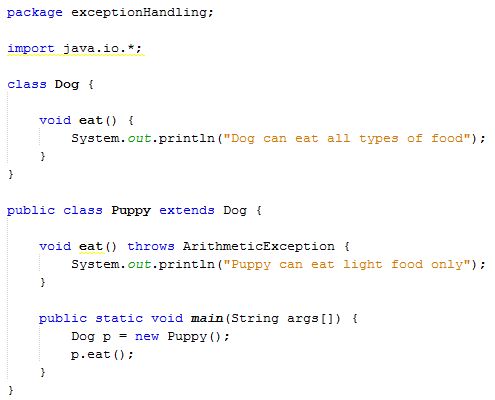
Output


In the above example, Dog (parent) class method does not declare an exception but Puppy (child) class overridden method can declare unchecked exception in Java.
When the parent class method declares an exception
Let’s see an example, given below.
Code
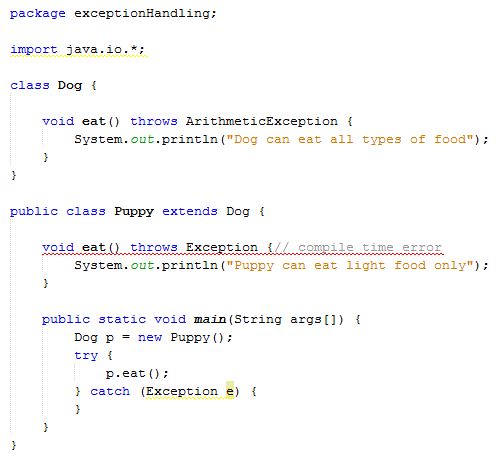
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() throws ArithmeticException {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() throws Exception { // compile time error
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- try {
- p.eat();
- } catch (Exception e) {}
- }
- }
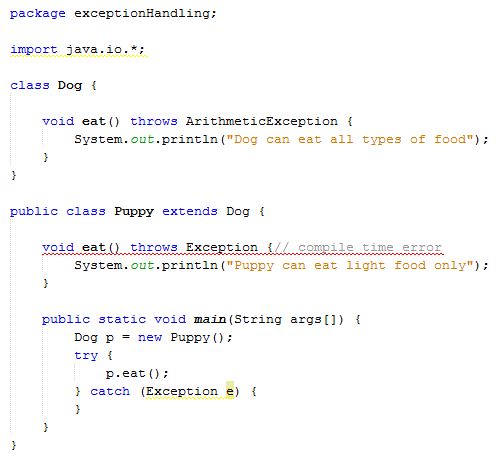
In the example, shown above, Dog (parent) class method declares an exception but Puppy (child) class overridden method can declare same, child class exception or no exception but cannot declare parent class exception. Thus, it gives a compile time error because puppy class declares Dog class exception.
Let’s see another example, given below.
Code
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() throws Exception {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() throws Exception {
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- try {
- p.eat();
- } catch (Exception e) {}
- }
- }
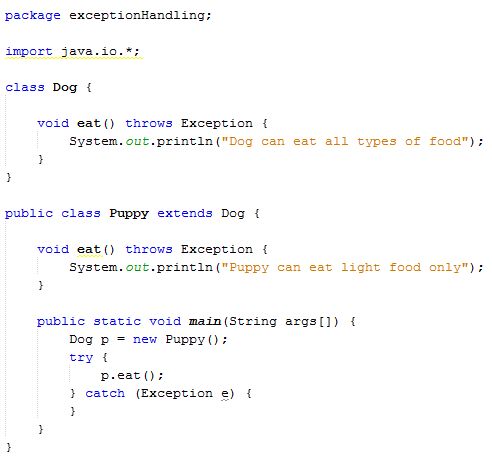
Output


In the example, shown above, Dog (parent) class method declares an exception but Puppy (child) class overridden method can declare the same exception.
Let’s see another example, given below.
Code
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() throws Exception {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() throws ArithmeticException {
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- try {
- p.eat();
- } catch (Exception e) {}
- }
- }
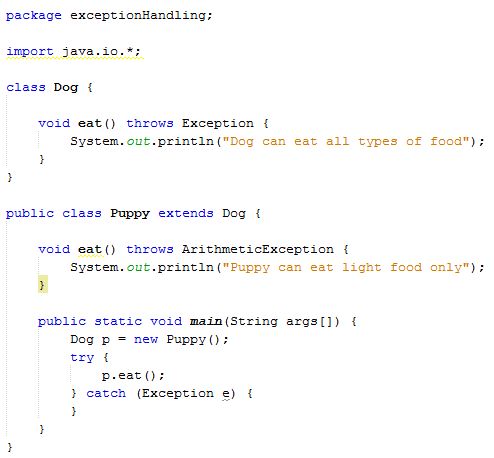
Output


In the example, shown above, Dog (parent) class method declares an exception but Puppy (child) class overridden method can declare the child class exception.
Let’s see another example, given below.
Code
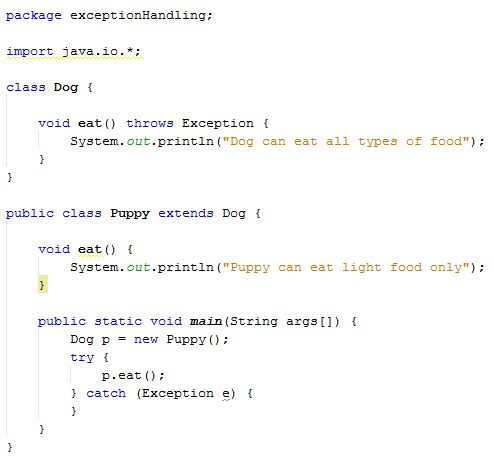
- package exceptionHandling;
- import java.io.*;
- class Dog {
- void eat() throws Exception {
- System.out.println("Dog can eat all types of food");
- }
- }
- public class Puppy extends Dog {
- void eat() {
- System.out.println("Puppy can eat light food only");
- }
- public static void main(String args[]) {
- Dog p = new Puppy();
- try {
- p.eat();
- } catch (Exception e) {}
- }
- }
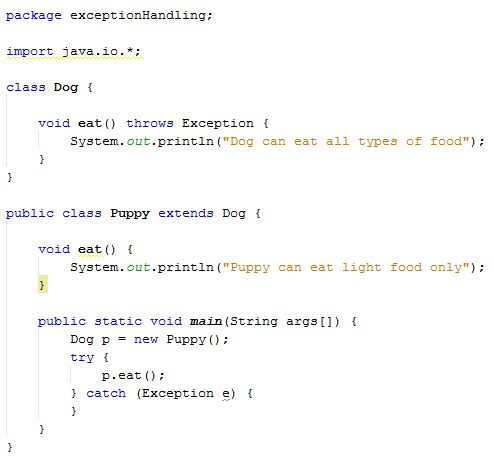
Output


In the example, shown above, Dog (parent) class method declares an exception but Puppy (child) class overridden method declares no exception.
Summary
Thus, we learnt the usage of ExceptionHandling with MethodOverriding in Java with the examples and also learnt its rules.