if-else Statement In Java
if-else Statements
There are many types of if statement in Java, which are,
- if statement.
- if-else statement.
- if-else-if statement.
- Nested if statement.
if statement
If statement is very basic of all control flow statements. It is used to test the condition, if the condition is right .Hence, it returns the output TRUE. It returns only boolean value true or false.
Syntax
if(condition){
// statement.
}
Let’s understand with the flowchart, given below.
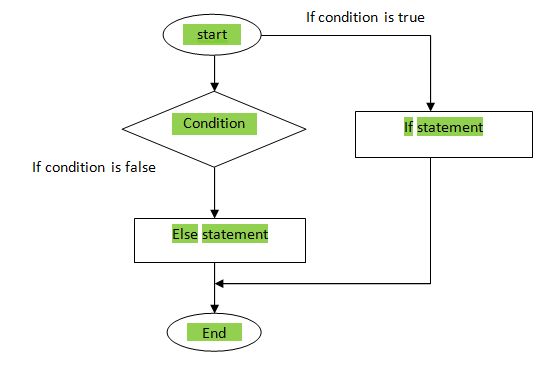
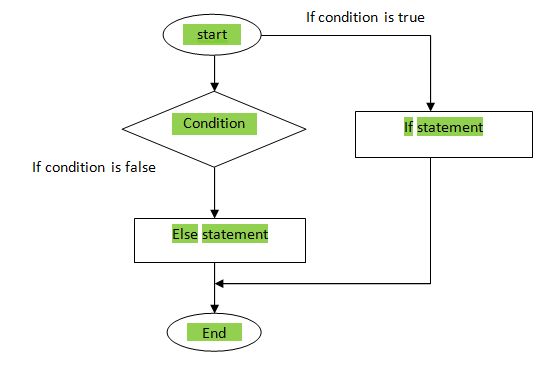
Let’s see an example.
Code
- public class IfStatement1 {
- public static void main(String[] args) {
- int no = 25;
- if (no > 18) {
- System.out.println("Number is greater than 18");
- }
- }
- }
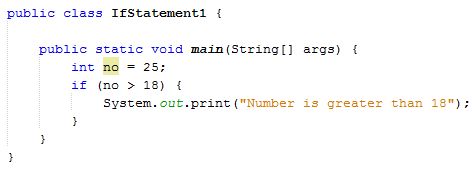
Output
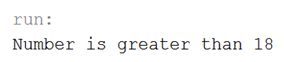
if-else statement
If – else statement is also used to test the condition but it provides a secondary path of the execution. It returns if statement when the condition is right and when the condition is wrong. Hence, it returns else statement.
Syntax
If (condition) {
// statement code.
} else {
//statement
}
}
Let’s understand with the flowchart, given below.
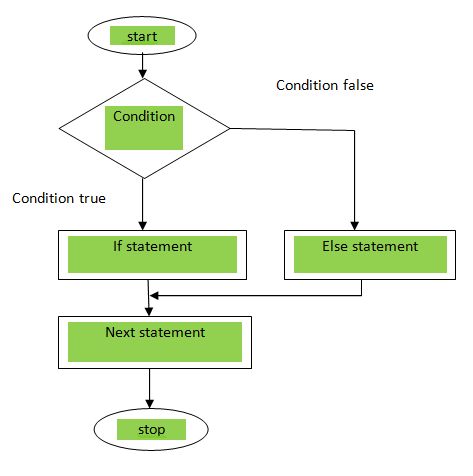
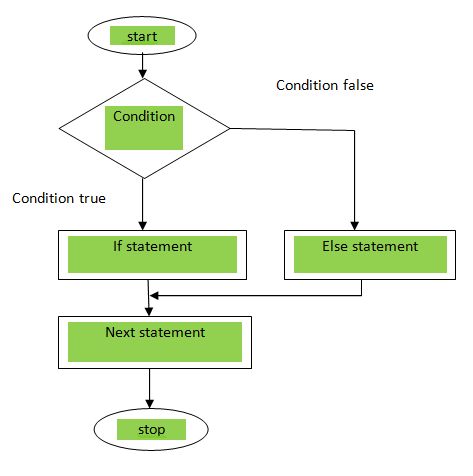
Let’s see an example.
Code
- public class IfelseStatement2 {
- public static void main(String[] args) {
- int num = 15;
- if (num % 2 == 0) {
- System.out.println("this number is even");
- } else {
- System.out.println("this number is odd");
- }
- }
- }
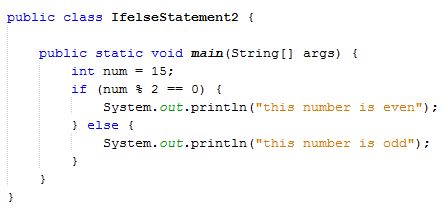
Output


if-else-if statement
if-else-if statement checks the one condition from the multiple statements. It is a different statement from others.
Syntax
if(condition1){
// code.
} else if (condition2) {
//code.
}
Else if (condition3){
//code.
}
else {
//code.
}
Let’s understand with the flowchart, given below.
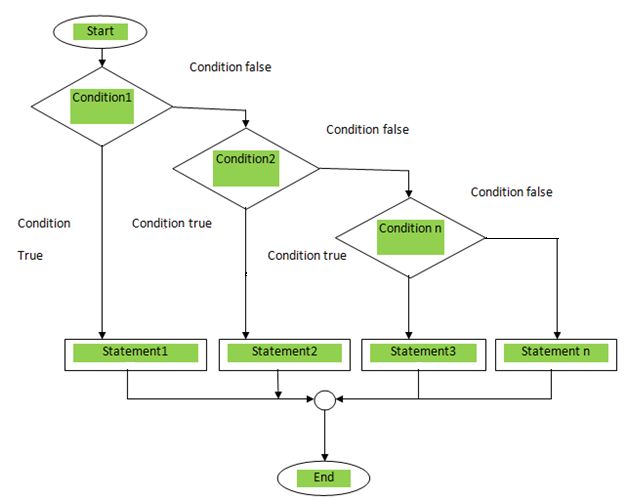
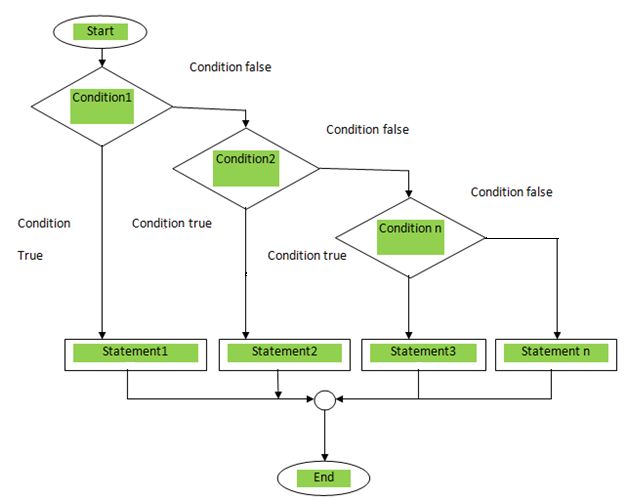
Let’s see an example, given below.
Code
- public class IfelseifStatement3 {
- public static void main(String[] args) {
- int marks = 80;
- if (marks < 50) {
- System.out.println("C grade");
- } else if (marks >= 50 && marks < 60) {
- System.out.println("C+ grade");
- } else if (marks >= 60 && marks < 70) {
- System.out.println("B grade");
- } else if (marks >= 70 && marks < 80) {
- System.out.println("B+ grade");
- } else if (marks >= 80 && marks < 90) {
- System.out.println("A grade");
- } else if (marks >= 90 && marks < 100) {
- System.out.println("A+ grade");
- } else {
- System.out.println("Unfounded");
- }
- }
- }
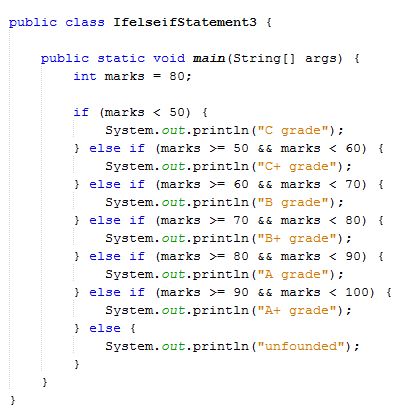
Output


Nested if Statement
In nested if statement, we can use one if statement or else if statement inside another if or else if statement. In other words, nested means one statement inside the other statement.
Syntax
if (condition1){
// code.
If (condition2) {
//code.
}
}
Let’s understand with the flowchart, given below.
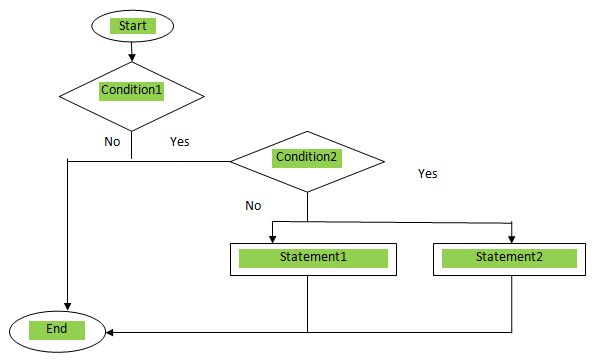
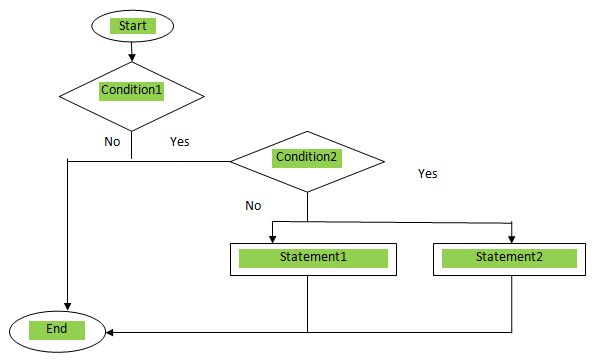
Let’s see an example, given below.
Code
- public class NestedifStatement4 {
- public static void main(String[] args) {
- int x = 20;
- int y = 10;
- if (x == 20) {
- if (y == 10) {
- System.out.println("X=20 and y=10");
- }
- }
- }
- }
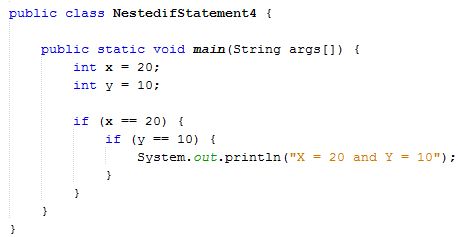
Output
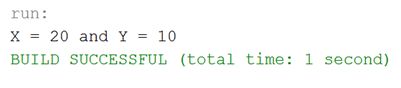
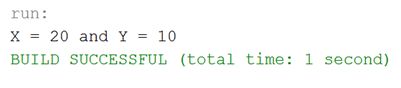
Summary
Thus, we learnt that if statement is very basic of all the control flow statements, it is used to test the condition and also learnt its four types in Java.