Final Keyword In Java
Final Keyword
In Java, “final” keyword is very important keyword, which is mainly used for restriction. We can use “final” keyword in many contexts such as variable, method and class.
Final variable
When we use “final” keyword with any variable, it is called as a final variable. Final variable can’t be changed. It will be constant.
Let’s see an example, given below.
Code
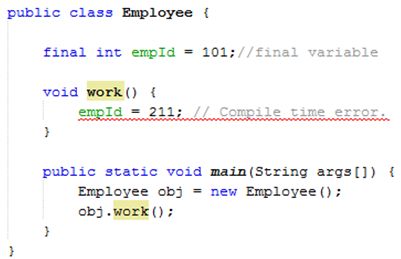
- public class Employee {
- final int empId = 101; //final variable
- void work() {
- empId = 211; // Compile time error
- }
- public static void main(String args[]) {
- Employee obj = new Employee();
- obj.work();
- }
- }
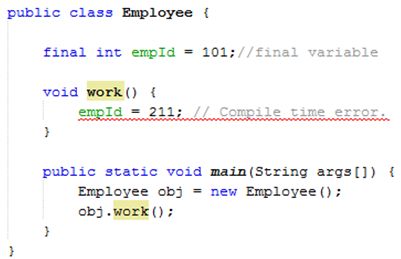
In the above example, we declare the final variable and initialize it at the time of declaration. Hence, we cannot initialize it again or we cannot change its value. It will be constant.
Blank or uninitialized final variable
In Java, blank or uninitialized final variable means a final variable, which is not initialized at the time of the declaration. We can initialize blank final variable only in constructor and if it is a static blank final variable so, it is initialized only in static block.
Let’s see an example of blank final variable, given below.
Code
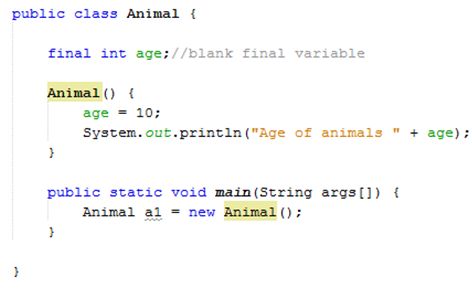
- public class Animal {
- final int age; //blank final variable
- Animal() {
- age = 10;
- System.out.println("Age of animal " + age);
- }
- public static void main(String args[]) {
- Animal a1 = new Animal();
- }
- }
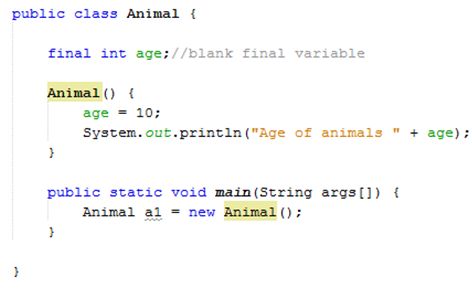
Output


Now, let’s see an example of static blank final variable, given below.
Code
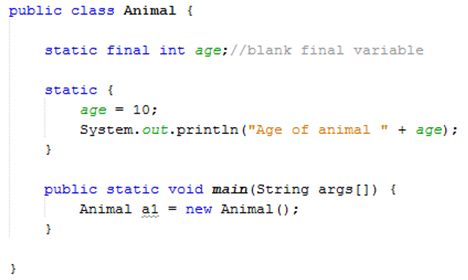
- public class Animal {
- static final int age; //blank final variable
- static {
- age = 10;
- System.out.println("Age of animal " + age);
- }
- public static void main(String args[]) {
- Animal a1 = new Animal();
- }
- }
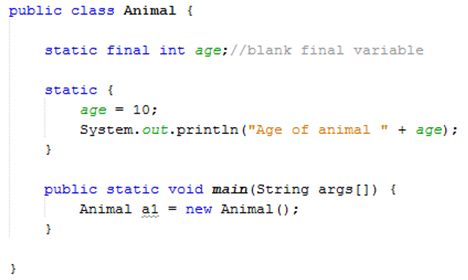
Output


Final method
When we use “final’ keyword with any method, it is called as a final method. Final method can’t be overridden.
Let’s see an example, given below.
Code
- class Employee {
- final void work() {
- System.out.println("Working");
- }
- }
- public class Manager extends Employee {
- void work() { // Compile time error
- System.out.println("working hard");
- }
- public static void main(String args[]) {
- Manager m1 = new Manager();
- m1.work();
- }
- }
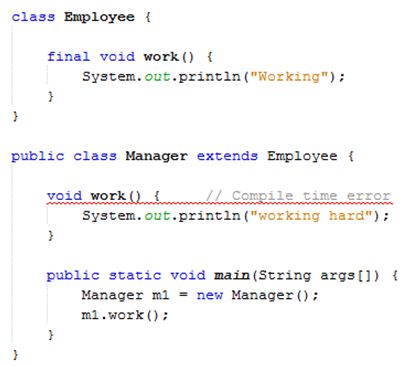
In the example, mentioned above, we declare the final method work (). If we declare any method as a final, we cannot override that method.
Final class
When we use “final” keyword with any class, it is called as a final class. Final class can’t be inheritted.
Let’s see an example, given below.
Code
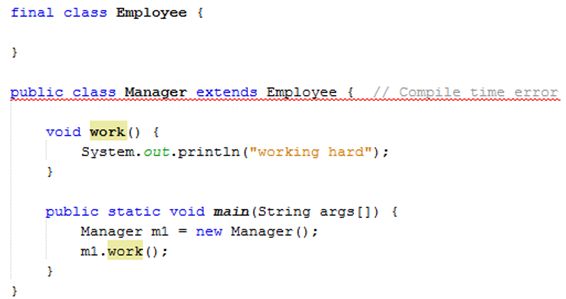
- final class Employee {}
- public class Manager extends Employee { // Compile time error
- void work() {
- System.out.println("working hard");
- }
- public static void main(String args[]) {
- Manager m1 = new Manager();
- m1.work();
- }
- }
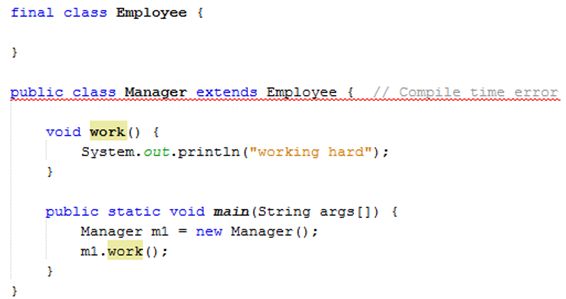
Can final method inherit?
Yes, we can inherit the final method but we cannot override it.
Let’ see an example, given below.
Code
- class Employee {
- final void work() {
- System.out.println("Working");
- }
- }
- public class Manager extends Employee {
- public static void main(String args[]) {
- Manager m1 = new Manager();
- m1.work();
- }
- }
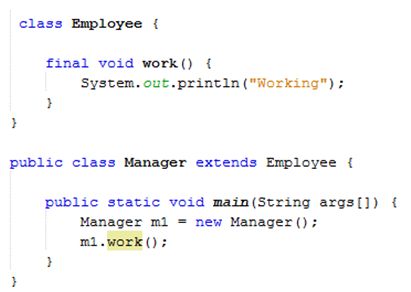
Output


We can’t declare a constructor final because constructor can’t be inherited.
Final parameter
If we declare any parameter as final, we can’t change the value of it.
Let’s see an example, given below.
Code
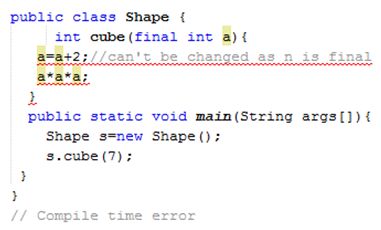
- public class Shape {
- int cube(final int a) {
- a = a + 2; //can't be changed as n is final
- a * a * a;
- }
- public static void main(String args[]) {
- Shape s = new Shape();
- s.cube(7);
- }
- }
- // Compile time error
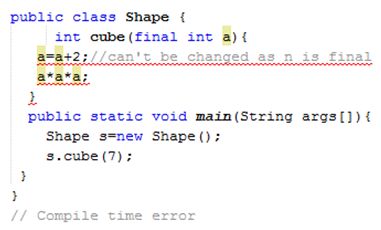
Summary
Thus, we learnt “final” keyword is very important keyword, which is mainly used for restriction and also learnt how to use it in Java.