Priority Of A Thread In Java
Thread Priority
Thread priorities are represented in number between 1 and 10. Thread scheduler schedules the threads , according to their priority, which are referred as a preemptive scheduling. There is no guarantee, because it fully depends on JVM specification that which scheduling, it chooses.
Each thread has a priority.
Three constants are defined in Thread class, which are,
public static int MIN_PRIORITY
public static int NORM_PRIORITY
public static int MAX_PRIORITY
By default thread priority is 5 (NORM_PRIORITY).
The value of MIN_PRIORITY is 1 and the value of MAX_PRIORITY is 10.
Let’s see an example, given below.
Code
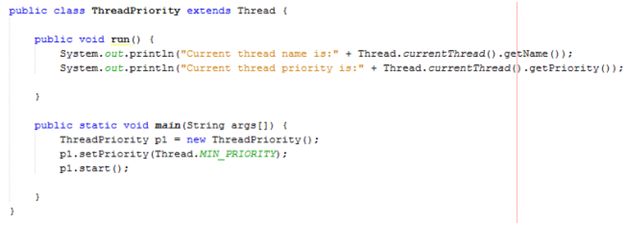
- public class ThreadPriority extends Thread {
- public void run() {
- System.out.println("Current thread name is:" + Thread.currentThread().getName());
- System.out.println("Current thread priority is:" + Thread.currentThread().getPriority());
- }
- public static void main(String args[]) {
- ThreadPriority p1 = new ThreadPriority();
- p1.setPriority(Thread.MIN_PRIORITY);
- p1.start();
- }
- }
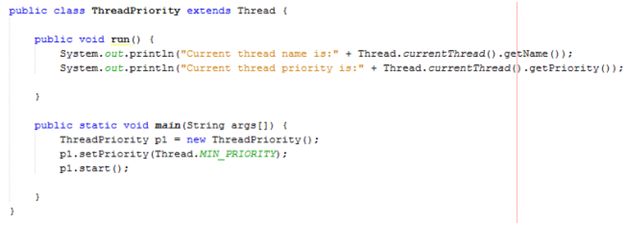
Output
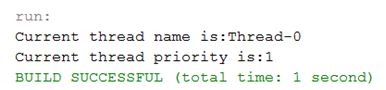
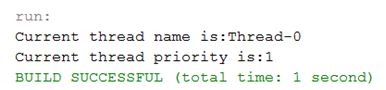
In the above example, we get the running thread name that is “Thread-0” and running thread minimum priority that is 1.
Summary
Thus, we learnt, by default, the thread priority is 5, MIN_PRIORITY is 1 and MAX_PRIORITY is 10 and also learnt, how we can use the thread priority in Java.