How To Sort In Collection
Sort the element
Collections class allows the static methods to sort the elements of the collection. If the collection elements are of Set type, we can use TreeSet but cannot sort the list elements. Collections class provides the methods to sort the elements of List type elements. We can sort the elements of the string objects, Wrapper class objects and User-defined class objects.
In Java, String class and Wrapper classes implements the comparable interface. Thus, if we store the objects of string or wrapper classes, they will be Comparable.
Method of Collections class to arrange List elements
public void sort(List list)
This method is used to sort the elements of the list but the list elements must be of comparable type.
Let’s see an example, given below.
Code
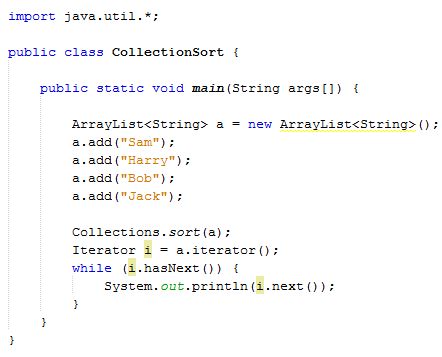
- import java.util.*;
- public class CollectionSort {
- public static void main(String args[]) {
- ArrayList < String > a = new ArrayList < String > ();
- a.add("Sam");
- a.add("Harry");
- a.add("Bob");
- a.add("Jack");
- Collections.sort(a);
- Iterator i = a.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- }
- }
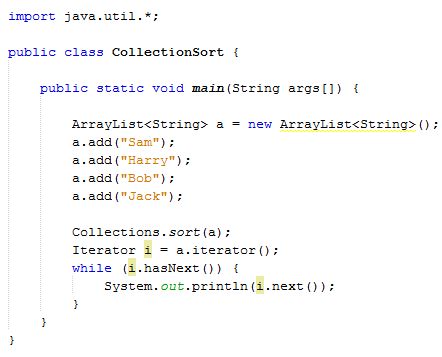
Output
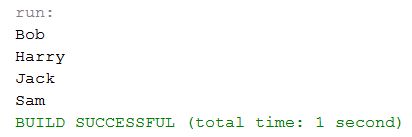
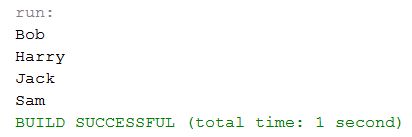
In the example, mentioned above, sorting the elements of the list contains the string objects.
Let’s see another example, given below.
Code
- import java.util.*;
- public class CollectionSort {
- public static void main(String args[]) {
- ArrayList a = new ArrayList();
- a.add(Integer.valueOf(45));
- a.add(Integer.valueOf(161));
- a.add(598);
- Collections.sort(a);
- Iterator i = a.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- }
- }
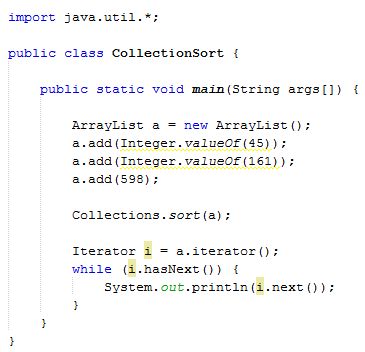
Output
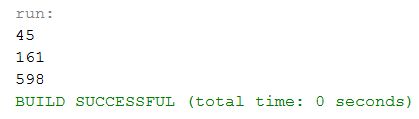
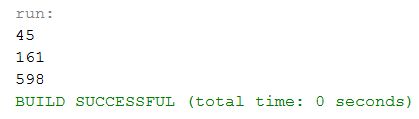
In the example, mentioned above, sorting the elements of the list, which contains Wrapper class objects.
Summary
Thus, we learnt that collections class provides the methods to sort the elements of the list type elements. We can sort the elements of the string objects, Wrapper class objects and User-defined class objects. We also learnt how to create it in Java.