Concatenation String In Java
Concatenation String
String concatenation simply forms a new string, which is the combination of multiple strings.
In Java two ways to concat string are given below.
- String concatenation by (+) operator.
- String concatenation by concat() method.
String Concatenation by (+) operator
With the use of (+) operator, we can add the two strings in Java, which are,
Let’s see an example, given below.
Code
- public class ConcatString {
- public static void main(String args[]) {
- String name = "Mia" + " Isabella";
- String address = "New" + "york";
- System.out.println(name);
- System.out.println(address);
- }
- }
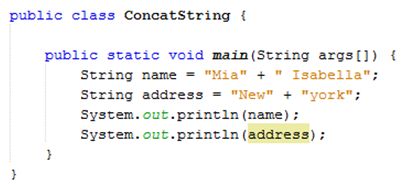
Output
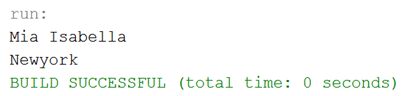
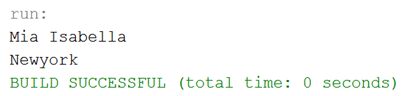
String Concatenation by concat() method
The string concat() method is used to concatenate the particular string to the end of the current string in Java.
Syntax
public String concat(String another)
Let's see an example, given below.
Code
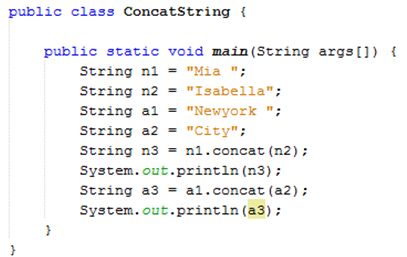
- public class ConcatString {
- public static void main(String args[]) {
- String n1 = "Mia ";
- String n2 = "Isabella";
- String a1 = "Newyork ";
- String a2 = "City";
- String n3 = n1.concat(n2);
- System.out.println(n3);
- String a3 = a1.concat(a2);
- System.out.println(a3);
- }
- }
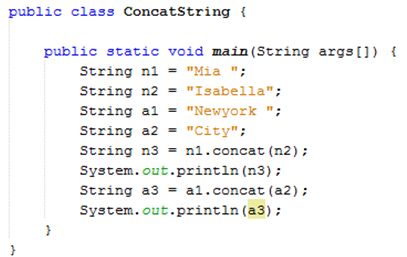
Output
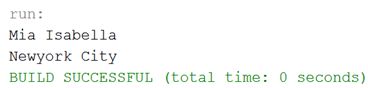
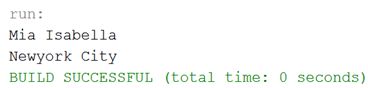
Summary
Thus, we learnt that the string concatenation means to form a new string, which is the combination of the multiple strings and also learnt their ways to concat the string in Java.