FileWriter And FileReader Class In Java
FileWriter And FileReader Class
In Java, character streams are used to perform input and output for 16-bit unicode. While there are many classes related to character streams but commonly used classes are FileReader and FileWriter. Internally, FileReader uses FileInputStream and FileWriter uses FileOutputStream but now major difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
When we want to write and read the data from the text files, FileWriter and FileReader classes are used. These are character-oriented classes, which are used for file handling in Java.
If we have to read and write the textual information, do not use the FileInputStream and FileOutputStream classes in Java.
FileWriter class
If we want to write character-oriented data, FileWriter class is used by the file in Java. This class inherits from the OutputStreamWriter class.
Constructors of FileWriter class
FileWriter(String file)
It is used to create a new file. It gets file name in the string.
FileWriter(File file)
It is used to create a new file. It gets the file name in the file object.
Methods of FileWriter class
public void write(String text)
This method is used to write the string into FileWriter.
public void write(char c)
This method is used to write the char into FileWriter.
public void write(char[] c)
This method is used to write char array into FileWriter.
public void flush()
This method is used to flush the data of FileWriter.
public void close()
This method is used to close FileWriter.
Let’s see an example, given below.
Code
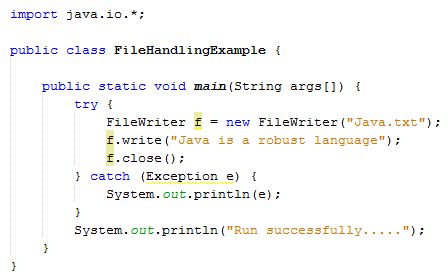
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) {
- try {
- FileWriter f = new FileWriter("Java.txt");
- f.write("Java is a robust language");
- f.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Run successfully.....");
- }
- }
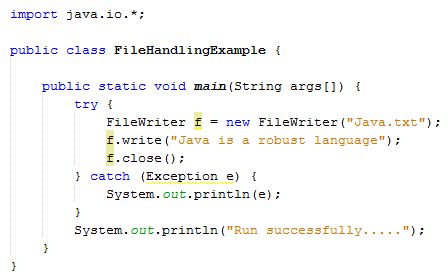
Output


In the above example, we write the data in the Java.txt file.
FileReader class
If we want to read the data from the file, FileReader class is used. It returns the data in byte format , similar to FileInputStream class. This class inherits from the InputStreamReader class.
Constructors of FileReader class
FileReader(String file)
It is used to get the filename in the string. It opens the given file in the read mode. If file doesn't exist, it throws FileNotFoundException.
FileReader(File file)
It is used to get the filename in file instance. It opens the given file in the read mode. If the file doesn't exist, it throws FileNotFoundException.
Methods of FileReader class
public int read()
This method is used to return a character in ASCII form. It returns -1 at the end of file.
public void close()
This method is used to close FileReader.
Let’s see an example, given below.
Code
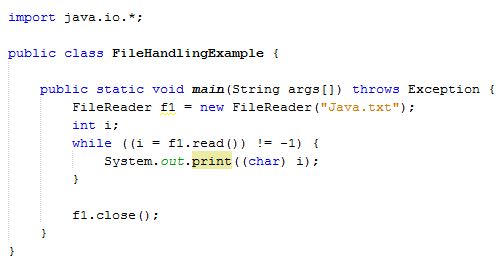
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- FileReader f1 = new FileReader("Java.txt");
- int i;
- while ((i = f1.read()) != -1) {
- System.out.print((char) i);
- }
- f1.close();
- }
- }
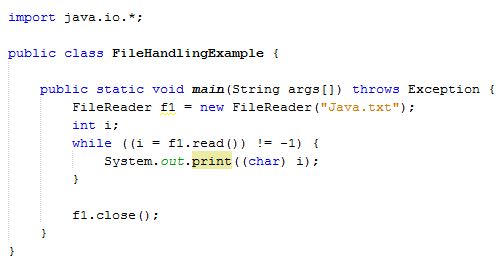
Output


In the example, mentioned above, we read the data from Java.txt file.
Summary
Thus, we learnt that when we want to write and read the data from the text files, FileWriter and FileReader classes are used in Java and also learnt its constructor and methods.