Nested Interface In Java
Nested Interface
When an interface is declared within another interface or a class, it is called as a nested interface.
The nested interface is used to group the related interfaces with the help of which, it becomes easy to maintain the code. The nested interface should be referred through the outer interface or class. It can't be accessed directly.
Nested interface should be public, if it is declared inside the interface but it can have any access modifier (public, private or protected), if declared within the class.
Nested interfaces are static by default.
Syntax
This is declared within the interface.
interface interface_name{
interface nested_interface_name{
}
}
Syntax
This is declared within the class.
class class_name{
interface nested_interface_name{
}
}
Let’s see an example of the nested interface, given below, which is declared within the interface.
Code
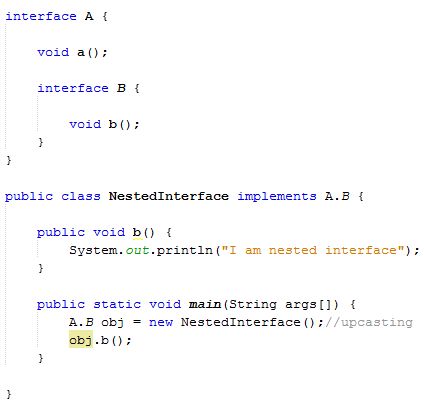
- interface A {
- void a();
- interface B {
- void b();
- }
- }
- public class NestedInterface implements A.B {
- public void b() {
- System.out.println("I am nested interface");
- }
- public static void main(String args[]) {
- A.B obj = new NestedInterface(); //upcasting
- obj.b();
- }
- }
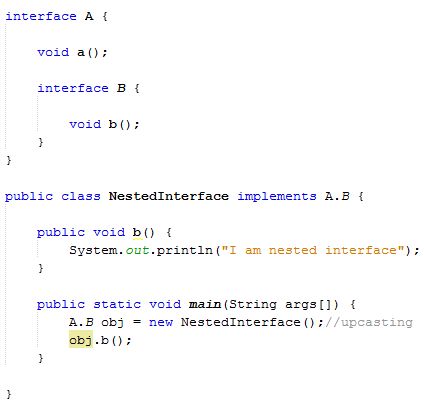
Output


In the example, mentioned above, we access the B interface by its outer interface A because it cannot be accessed directly.
Internal code generated by the compiler for the nested interface B.
The compiler internally creates public and static interface, as displayed below.
public static interface A$B
{
public abstract void b();
}
Let’s see an example of the nested interface, which is declared within the class.
Code
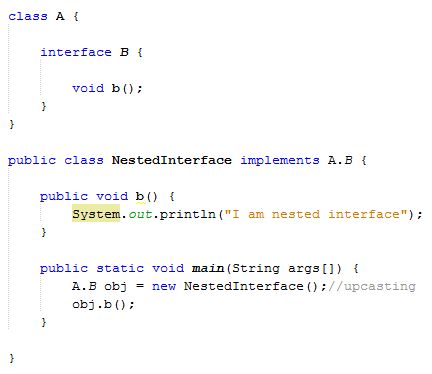
- class A {
- interface B {
- void b();
- }
- }
- public class NestedInterface implements A.B {
- public void b() {
- System.out.println("I am nested interface");
- }
- public static void main(String args[]) {
- A.B obj = new NestedInterface(); //upcasting
- obj.b();
- }
- }
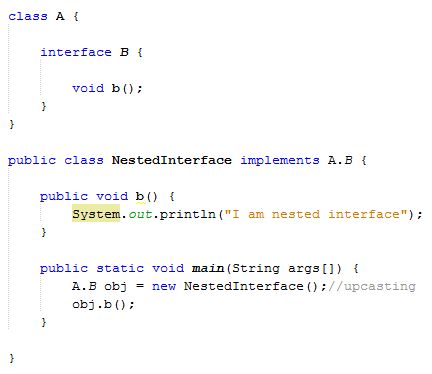
Output


We can define a class inside the interface
Yes, if we define a class inside any interface, Java compiler creates a static nested class.
How can we define a class within the interface? Let’s see,
interface Runable{
class A{}
}
Summary
Thus, we learnt that an interface declared within another interface or a class is called a nested interface in Java and also learnt how to create it.