Wrapper Class In Java
Wrapper Class
In Java, Wrapper class provides the concept to convert primitive into an object and an object into primitive. They "wrap" the primitive data type into an object of the class. Wrapper classes are a part of the java.lang package, which is imported by default into all Java programs.
Since Java 5, add new features of Java autoboxing and unboxing. These features convert automatically primitive into an object and object into primitive. The automatic conversion of primitive into an object is known as autoboxing and unboxing.
Use of Wrapper class in Java
- To wrap primitive into an object. According to it, primitives can perform the activities reserved for the objects collection.
- To provide a collection of utility functions for the primitives like converting primitive types to and from the string objects, converting to the various bases and comparing various objects.
In Java, eight classes of java.lang package are called as wrapper class. The list of eight wrapper classes is given below.
Primitive Type | Wrapper class |
boolean | Boolean |
Char | Character |
Byte | Byte |
Short | Short |
Int | Integer |
Long | Long |
Float | Float |
double | Double |
Wrapper class hierarchy as per Java API
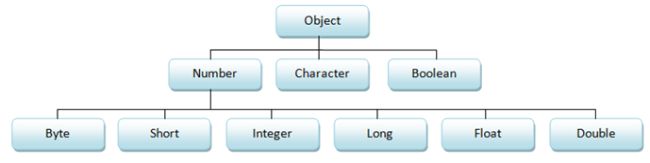
Let’s see an example of primitive to wrapper class, given below.
Code
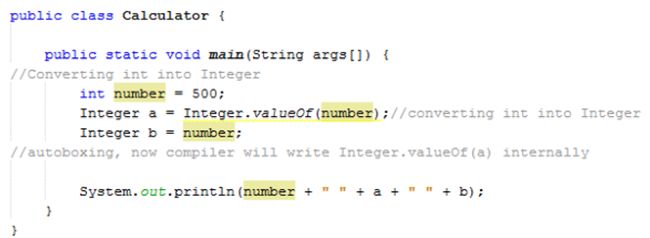
- public class Calculator {
- public static void main(String args[]) {
- //Converting int into Integer
- int number = 500;
- Integer a = Integer.valueOf(number); //converting int into Integer
- Integer b = number; //autoboxing, now compiler will write Integer.valueOf(a) internally
- System.out.println(number + " " + a + " " + b);
- }
- }
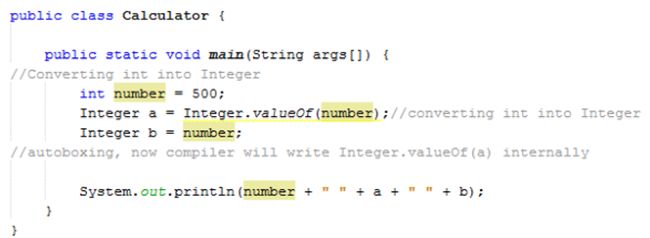
Output


Let’s see an example of Wrapper class to primitive.
Code
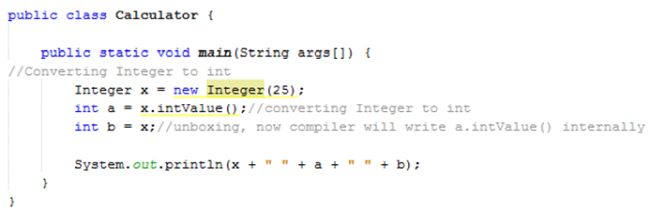
- public class Calculator {
- public static void main(String args[]) {
- //Converting Integer to int
- Integer x = new Integer(25);
- int a = x.intValue(); //converting Integer to int
- int b = x; //unboxing, now compiler will write a.intValue() internally
- System.out.println(x + " " + a + " " + b);
- }
- }
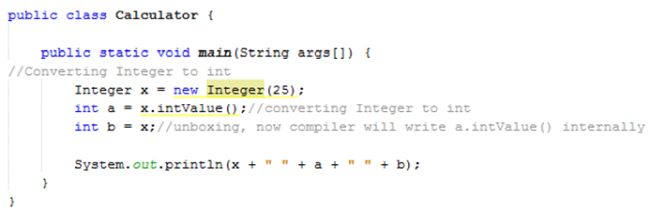
Output


Summary
Thus, we learnt that Wrapper class provides the concept to convert primitive into object and object into primitive. We also learnt use of it in Java.