SequenceInputStream Class In Java
SequenceInputStream Class
To read data from the multiple streams, SequenceInputStream class is used and the data is read by it one by one.
Constructors of SequenceInputStream class
SequenceInputStream(InputStream s1, InputStream s2)
It is used to create a new input stream by reading the data of two input stream in order, first s1 and then s2.
SequenceInputStream(Enumeration e)
It is used to create a new input stream by reading the data of an enumeration whose type is InputStream.
Let’s see an example, given below.
Code
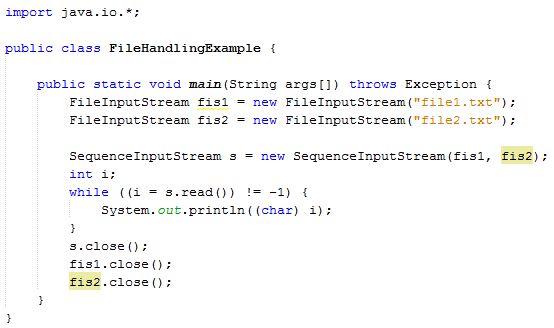
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- FileInputStream fis1 = new FileInputStream("file1.txt");
- FileInputStream fis2 = new FileInputStream("file2.txt");
- SequenceInputStream s = new SequenceInputStream(fis1, fis2);
- int i;
- while ((i = s.read()) != -1) {
- System.out.println((char) i);
- }
- s.close();
- fis1.close();
- fis2.close();
- }
- }
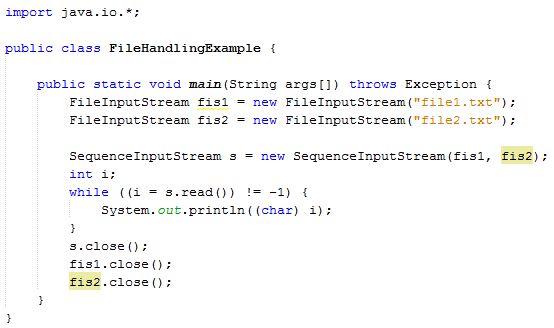
In the example, mentioned above, first read the files one by one and then print the data of both the files file1.txt and file2.txt.
Let’s see another example, given below.
Code
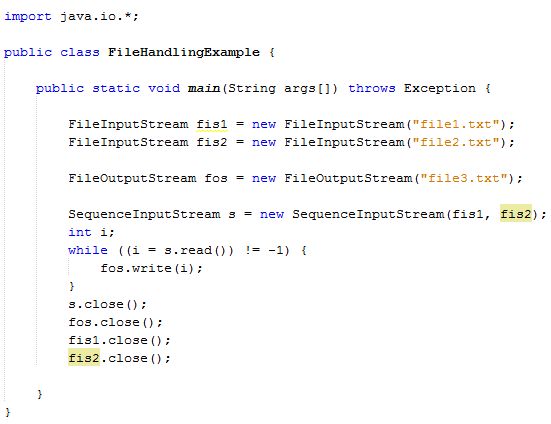
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- FileInputStream fis1 = new FileInputStream("file1.txt");
- FileInputStream fis2 = new FileInputStream("file2.txt");
- FileOutputStream fos = new FileOutputStream("file3.txt");
- SequenceInputStream s = new SequenceInputStream(fis1, fis2);
- int i;
- while ((i = s.read()) != -1) {
- fos.write(i);
- }
- s.close();
- fos.close();
- fis1.close();
- fis2.close();
- }
- }
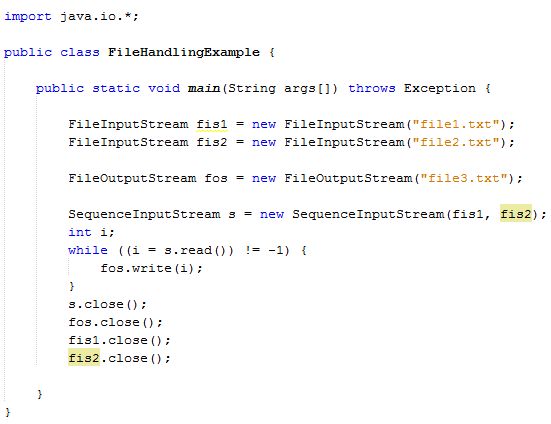
In the example, mentioned above, we are writing the data of the two files file1.txt and file2.txt into another file named file3.txt.
Summary
Thus, we learnt to read the data from the multiple streams, SequenceInputStream class is used in Java and also learnt how we can create it.