Transaction Management In JDBC
Transaction Management
In JDBC, transaction means a single unit of work. A transaction is a group of operations used to perform one task, if all the operations in the group are success, the task is finished and the transaction is successfully completed. If anyone operation in the group is fails, the task fails and the transaction is fails.
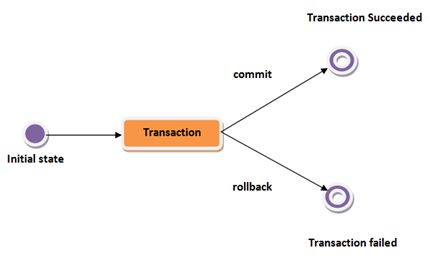
The ACID properties describes the transaction management well. ACID means Atomicity, Consistency, Isolation and Durability.
Atomicity denotes either all are successful or none.
Consistency makes sure to bring the database from one consistent state to the consistent state.
Isolation makes sure that the transaction is isolated from the other transaction.
Durability denotes once a transaction has been committed, it will remain thus, even in the event of errors, power loss etc.
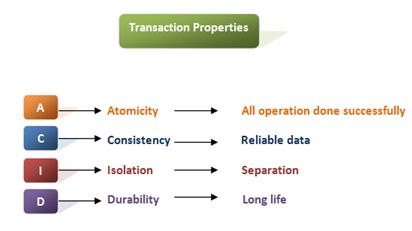
Advantage of Transaction Management
Fast performance: Because the database is hit at the time of commit and it makes the performance fast.
Connection interface provides some methods to manage transaction in JDBC which are,
void setAutoCommit(boolean status)
It is true by default, which means each transaction is committed by default.
void commit()
This method is used to commit the transaction.
void rollback()
This method is used to cancel the transaction.
Let's see an example of the transaction management, using Statement.
Code
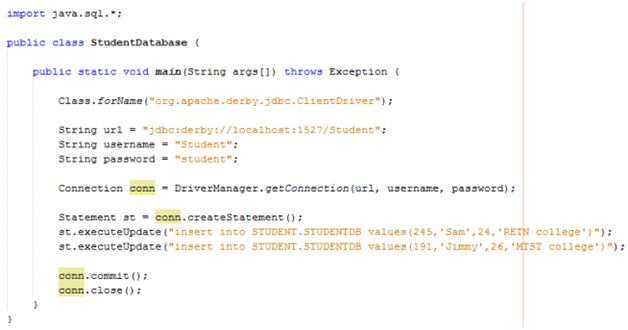
- import java.sql.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- Statement st = conn.createStatement();
- st.executeUpdate("insert into STUDENT.STUDENTDB values(245,'Sam',24,'RETN college')");
- st.executeUpdate("insert into STUDENT.STUDENTDB values(191,'Jimmy',26,'MTST college')");
- conn.commit();
- conn.close();
- }
- }
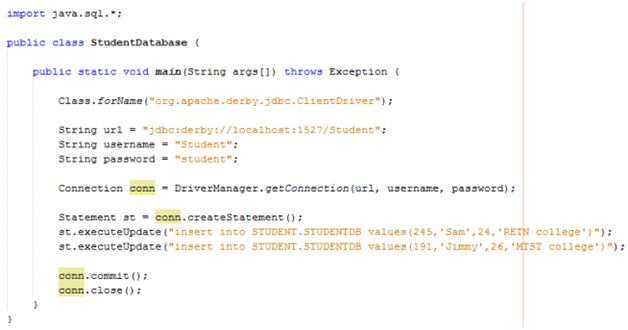
If we see the table Student, we will see that the two records have been added.
Let's see an example of the transaction management, using PreparedStatement.
Code
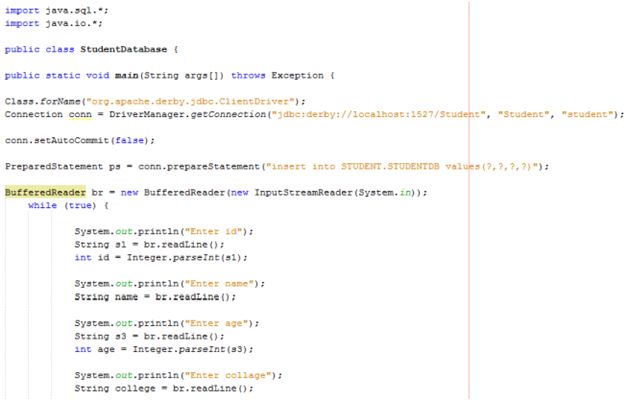
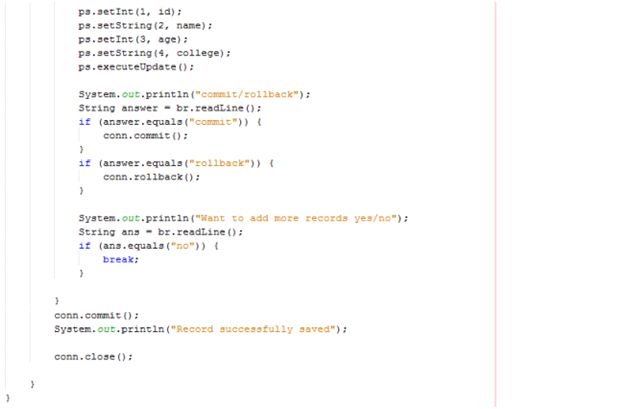
- import java.sql.*;
- import java.io.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- Connection conn = DriverManager.getConnection("jdbc:derby://localhost:1527/Student", "Student", "student");
- conn.setAutoCommit(false);
- PreparedStatement ps = conn.prepareStatement("insert into STUDENT.STUDENTDB values(?,?,?,?)");
- BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
- while (true) {
- System.out.println("Enter id");
- String s1 = br.readLine();
- int id = Integer.parseInt(s1);
- System.out.println("Enter name");
- String name = br.readLine();
- System.out.println("Enter age");
- String s3 = br.readLine();
- int age = Integer.parseInt(s3);
- System.out.println("Enter collage");
- String college = br.readLine();
- ps.setInt(1, id);
- ps.setString(2, name);
- ps.setInt(3, age);
- ps.setString(4, college);
- ps.executeUpdate();
- System.out.println("commit/rollback");
- String answer = br.readLine();
- if (answer.equals("commit")) {
- conn.commit();
- }
- if (answer.equals("rollback")) {
- conn.rollback();
- }
- System.out.println("Want to add more records yes/no");
- String ans = br.readLine();
- if (ans.equals("no")) {
- break;
- }
- }
- conn.commit();
- System.out.println("Record successfully saved");
- conn.close();
- }
- }
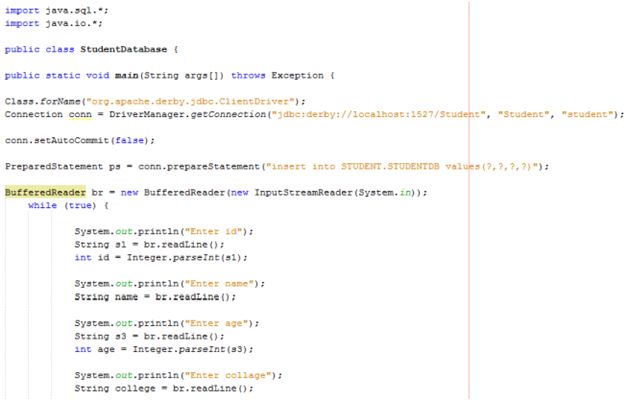
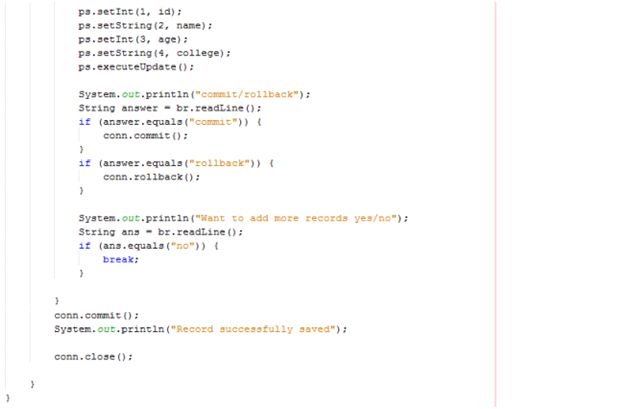
Output
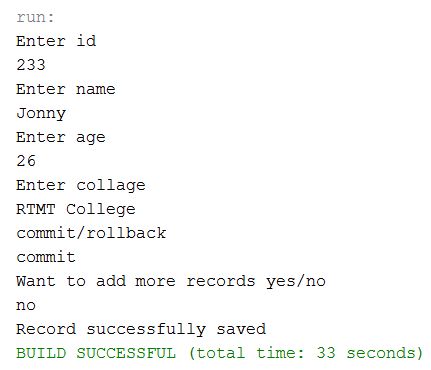
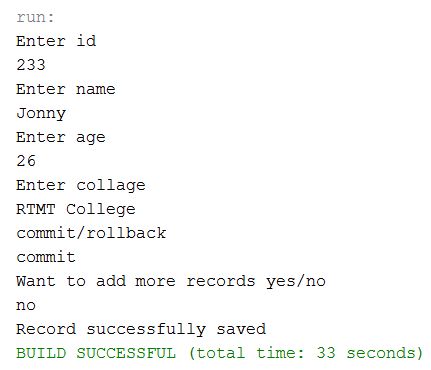
In the example mentioned above, it will ask to add more records until you press no. If we press no, the transaction is committed.
Summary
Thus, we learnt, JDBC transaction means a single unit of work and also learnt, how we can use it in Java through the examples.