Dynamic And Static Binding In Java
Binding
In Java, binding means connection of the method definition to the method call.
In other words, related method calls the method body, which is called as a binding.
There are two types of binding in Java, which are,
- Static binding
- Dynamic binding
First, we discuss few terms, which is important to understand the concepts of binding.
Types of instance are,
- Variables type
Every variable has a type primitive and non-primitive. Like- Int n = 10; // n variable is a type of int.
- References type
A reference type is a data type which based on a class rather than on one of the primitive types that are built in Java.- class Student {
- public static void main(String args[]) {
- Student s; // s is a type of student class.
- Object type
An object is an instance of particular Java class and it is also an instance of its parent class.- class Car {}
- class Nano extends Car {
- public static void main(String args[]) {
- Nano n1 = new Nano(); // n1 is an instance of Nano class and also an instance of Car class.
Static binding
In Java, when type of the object can be resolved at the compile time by the compiler, it is known as static or early binding.
If a class has any private, final or static method, there is static binding.
Let’s see an example, given below,
Code
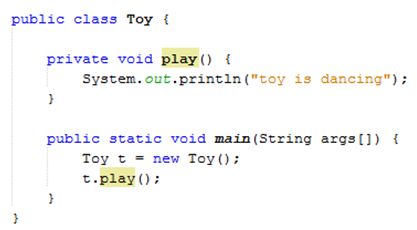
- public class Toy {
- private void play() {
- System.out.println("toy is dancing");
- }
- public static void main(String args[]) {
- Toy t = new Toy();
- t.play();
- }
- }
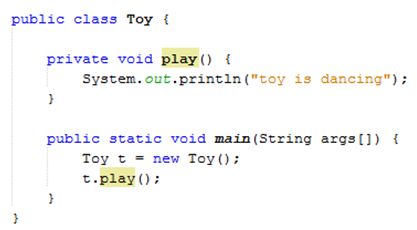
Output


Dynamic binding
In Java, when type of the object can be resolved at runtime, it is known as a dynamic or late binding.
Let’s see an example, given below.
Code
- class Toy {
- void play() {
- System.out.println("Toy is dancing");
- }
- }
- public class Doll extends Toy {
- void play() {
- System.out.println("Doll is dancing and singing");
- }
- public static void main(String args[]) {
- Toy t = new Doll();
- t.play();
- }
- }
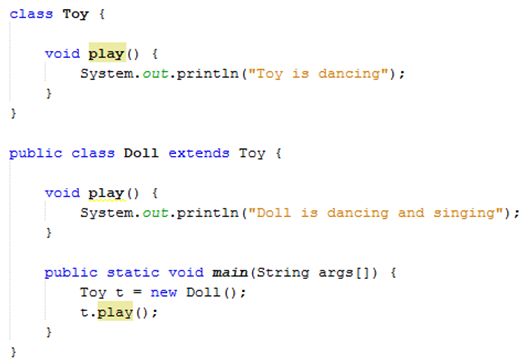
Output


In the example, mentioned above, an object type can’t be resolved at a compile time by the compiler because instance of doll is also an instance of toy. Therefore, the compiler doesn’t know its type at the compile time. It knows only its base type.
Summary
Thus, we learnt that the related method calls the method body is called a binding in Java and also learnt its types of dynamic binding and static binding.