Nested Try Block In Java
Nested Try Block
When we use one try block in another try block, it is called as nested try block in Java.
In other words, Java try catch blocks can be nested. One try-catch block can be present in another try block. Due to this, it is called as nesting of try catch blocks. Every time, a try block doesn’t have a catch handler for a particular exception. Thus, the stack is unwound and the next try block’s catch handlers are checked for a match. If no catch block matches, the JVM will handle the exception.
Why we use nested try block
Nested try block is used when some situation may arise, where a part of a block may cause one error and the other whole block itself may cause a different error. In this case, exception handlers have to be nested in Java.
Syntax
....
try // main try block
{
Statement 1;
Statement 2;
try //try-catch block inside another try block
{
Statement 3;
Statement 4;
}
catch(Exception e)
{
}
}
catch(Exception e1)
{
}
....
Let's see an example, given below.
Code
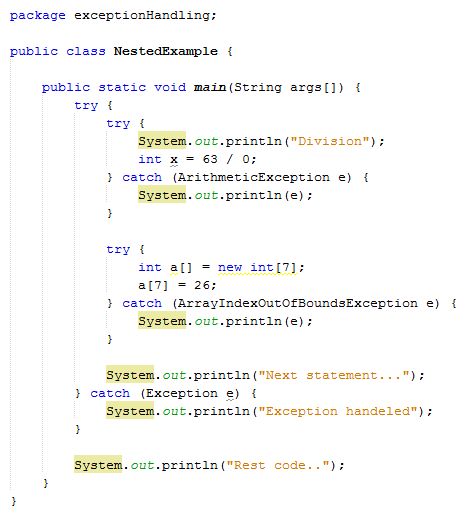
- package exceptionHandling;
- public class NestedExample {
- public static void main(String args[]) {
- try {
- try {
- System.out.println("Division");
- int x = 63 / 0;
- } catch (ArithmeticException e) {
- System.out.println(e);
- }
- try {
- int a[] = new int[7];
- a[7] = 26;
- } catch (ArrayIndexOutOfBoundsException e) {
- System.out.println(e);
- }
- System.out.println("Next statement...");
- } catch (Exception e) {
- System.out.println("Exception handeled");
- }
- System.out.println("Rest code..");
- }
- }
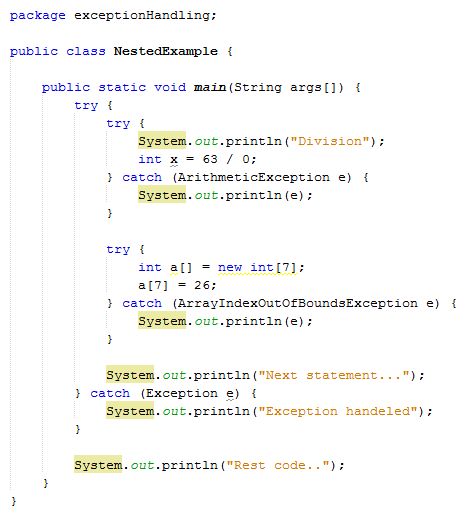
Output
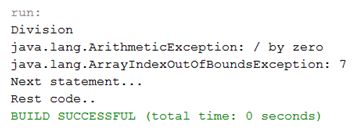
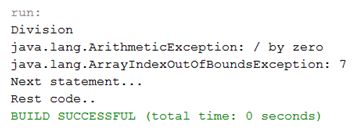
In the example shown above, nested try catch is used in Java.
The main point in this example is that when the inner try-catch blocks are not handling any exception, the flow of control comes back to the outer try-catch block and if the exception is not handled in the outer try-catch block also, the program will terminate immediately.
Summary
Thus, we learnt that if we use one try block in another try block, it is known as nested try block and also learnt why we need to use it in Java.