Annotations In Java
Annotations
In Java, annotation is a tag, which shows the metadata that is attached with the class, interface and methods to indicate some additional information, which can be used by Java compiler and JVM.
Annotations are mainly used to provide the additional information. Thus, it is an alternative option for XML and Java marker interfaces.
Built-In Java Annotations
In Java, there are many built-in annotations. Some annotations are useful for Java code and few for other annotations.
Built-In Java annotations, which are used in Java code are,
- @Override
- @SuppressWarnings
- @Deprecated
Built-In Java Annotations which used in other annotations are,
- @Target
- @Retention
- @Inherited
- @Documented
Built-In Annotations in Java
@Override
@Override annotation checks that the child class method is overriding the parent class method. If it does not, the compile time error occurs. To mark @Override annotation is better because it provides assurance that the method is overridden.
Let’s see an example of @Override annotation, given below.
Code
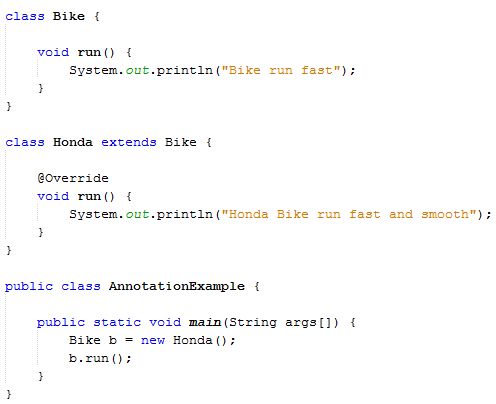
- class Bike {
- void run() {
- System.out.println("Bike run fast");
- }
- }
- class Honda extends Bike {
- @Override
- void run() {
- System.out.println("Honda Bike run fast and smooth");
- }
- }
- public class AnnotationExample {
- public static void main(String args[]) {
- Bike b = new Honda();
- b.run();
- }
- }
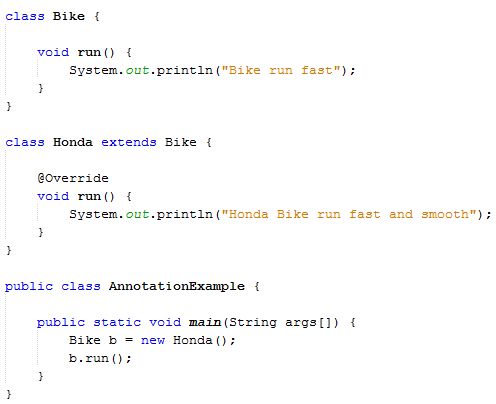
Output


@SuppressWarnings
@SuppressWarnings annotation suppresses the warnings issued by the compiler.
Let’s see an example of @SuppressWarnings annotation.
Code
- import java.util.*;
- public class AnnotationExample {
- @SuppressWarnings("Unchecked")
- public static void main(String args[]) {
- ArrayList a = new ArrayList();
- a.add("Bob");
- a.add("Jack");
- a.add("Mia");
- for (Object obj: a) {
- System.out.println(obj);
- }
- }
- }
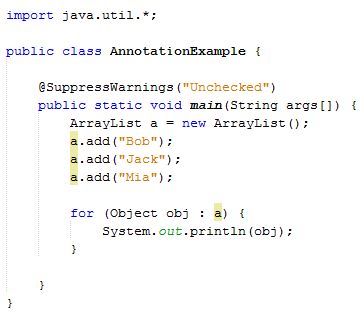
Output
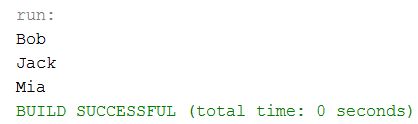
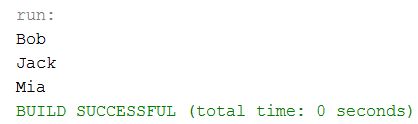
In the example, mentioned above, there is no warning at the compile time and If we remove the @SuppressWarnings("unchecked") annotation, it will show the warning at the compile time because we are using non-generic collection.
@Deprecated
@Deprecated annotation marks that this method is deprecated. Thus, the compiler prints the warning and it informs the user that it may be removed in the future versions. Hence, it is better not to use such methods.
Let’s see an example of @Deprecated annotation.
Code
- class Simple {
- void a() {
- System.out.println("a() method is calling...");
- }
- @Deprecated
- void b() {
- System.out.println("b() method is calling...");
- }
- }
- public class AnnotationExample {
- public static void main(String args[]) {
- Simple s = new Simple();
- s.b();
- }
- }
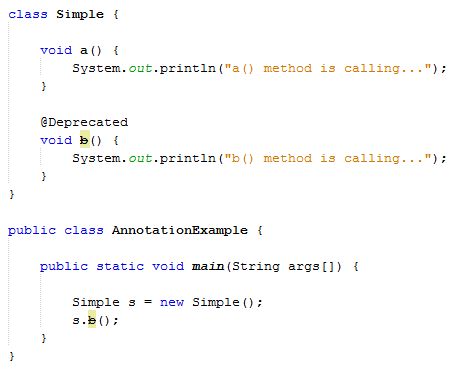
Output


In the example, mentioned above, program runs normally at the runtime but at the compile time, annotation. Example.java uses or overrides a deprecated API and is recompiled by –Xlint:deprecation for the details.
Summary
Thus, we learnt that Java annotations are mainly used to provide the additional information. Thus, it is an alternative option for XML and Java marker interfaces. We also learnt how we can use it in Java.