ThreadGroup Class In Java
ThreadGroup Class
A group of multiple threads in a single object is called a ThreadGroup in Java.
With the help of ThreadGroup class, we can suspend, resume or interrupt group of threads with a single method call.
Suspend () method, resume () method and stop () method are deprecated now.
ThreadGroup is implemented by java.lang.ThreadGroup class.
Constructors of ThreadGroup class
ThreadGroup(String name)
It is used to create a thread group with the given name.
ThreadGroup(ThreadGroup parent, String name)
It is used to create a thread group with the given parent group and name.
Methods of ThreadGroup class
int activeCount()
This method is used to return no. of threads running in current group.
int activeGroupCount()
This method is used to return a no. of active group in this thread group.
void destroy()
This method is used to destroy this thread group and all its sub groups.
String getName()
This method is used to return the name of this group.
ThreadGroup getParent()
This method is used to return the parent of this group.
void interrupt()
This method is used to interrupt all the threads of the group.
void list()
This method is used to print the information of the group to standard console.
Let’s see an example of ThreadGroup, given below.
Code
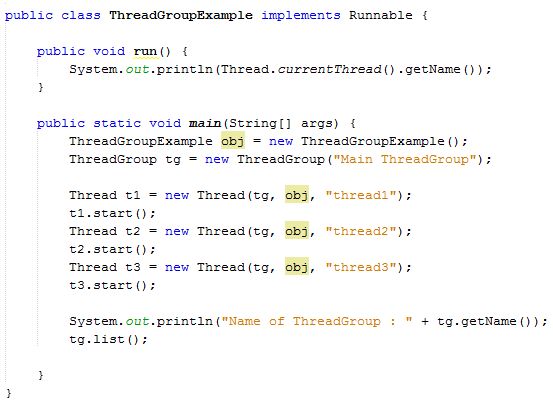
- public class ThreadGroupExample implements Runnable {
- public void run() {
- System.out.println(Thread.currentThread().getName());
- }
- public static void main(String[] args) {
- ThreadGroupExample obj = new ThreadGroupExample();
- ThreadGroup tg = new ThreadGroup("Main ThreadGroup");
- Thread t1 = new Thread(tg, obj, "thread1");
- t1.start();
- Thread t2 = new Thread(tg, obj, "thread2");
- t2.start();
- Thread t3 = new Thread(tg, obj, "thread3");
- t3.start();
- System.out.println("Name of ThreadGroup : " + tg.getName());
- tg.list();
- }
- }
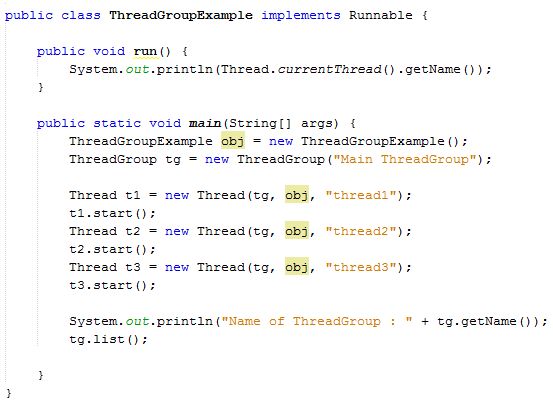
Output
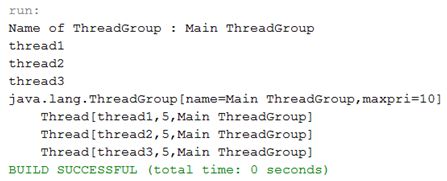
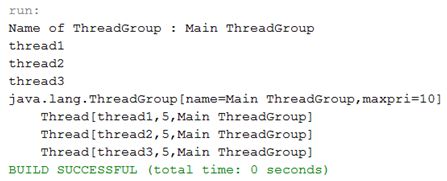
In the example, mentioned above, we created three threads, which belong to one group. Here, tg is the thread group name, the ThreadGroupExample class implements Runnable interface and "thread1", "thread2", "thread3" are the thread names and at the present, we can interrupt all the threads easily.
Let’s see an example, given below.

Let’s see an example, given below.

Summary
Thus, we learnt a group of multiple threads in a single object is called as a ThreadGroup and also learnt how to create it in Java.