Object And Class In Java
In Java, the object and class is a basic technique of an object oriented programming language. In this, we create a program, using objects and classes.
Object
Object is like an entity, which has a state and behavior. For example- mobile, bike, pencil, cat, etc. As a car has states (its color, name and brand) and behavior (running), object is an instance of a class and an object can be tangible and intangible.
- Object represents identity, which uniquely identifies it. Like one car no. plate is different number of other cars so it’s a car’s identity which is unique.
- Object represents a value of an object like car name, brand and its color.
- Object represents the functionality of an object like running.
Creating an Object
In Java, the new keyword is used to create new object.
For example
Code
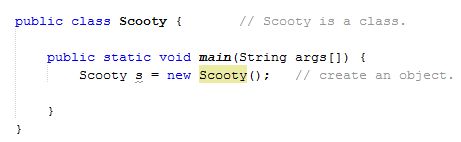
- public class Scooty { // Scooty is a class.
- public static void main(String args[]) {
- Scooty s = new Scooty(); // create an object.
- }
- }
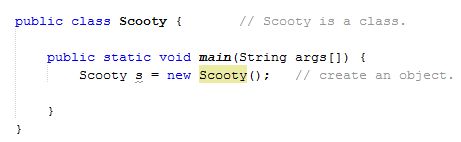
In Java, there are many other ways to create an object.
- By new Keyword
- By newInstance () method
- By clone () method
- By factory method
Class
Class is a template or we can say that it is a blueprint, where objects, data members and methods are defined.
Class can contain data member, method, constructor, block, class and an interface.
Syntax of class
class <class_name>{
data member;
method;
}
For example
Code
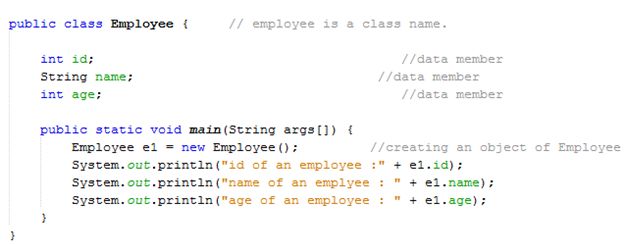
- public class Employee { //employee is a class name
- int id; //data member
- String name; //data member
- int age; //data member
- public static void main(String args[]) {
- Employee e1 = new Employee(); //creating an object of Employee
- System.out.println("id of an employee :" + e1.id);
- System.out.println("name of an employee :" + e1.name);
- System.out.println("age of an employee :" + e1.age);
- }
- }
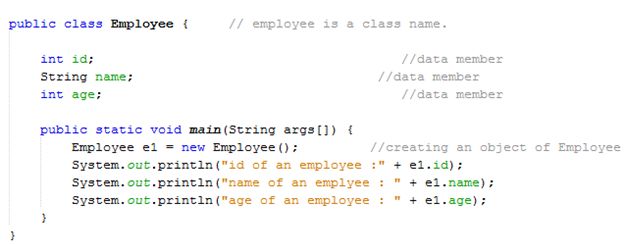
Output
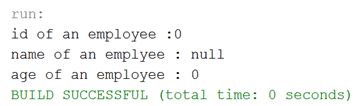
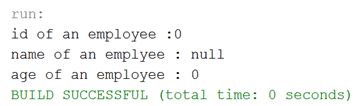
Instance variable in Java
Instance variable is declared inside the class but outside the method, constructor or block. It has the widest scope because it is globally visible to the whole class. It is created at the time of object creation. When an instance variable is created, it takes space in the heap memory and it is called through an object and default value is 0.
Method in Java
Method is like a function, which is used to represent a behavior of an object. It has many benefits as we can reuse the code and optimize the code.
For example
Code
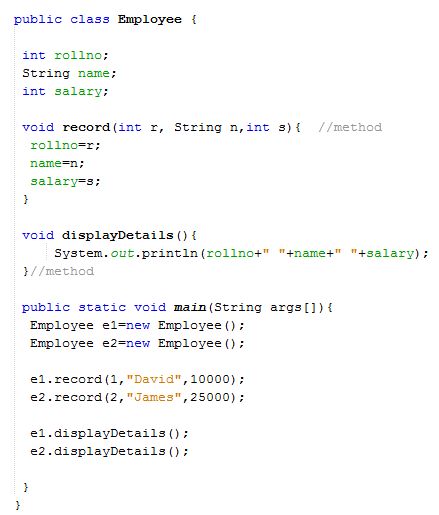
- public class Employee {
- int rollno;
- String name;
- int salary;
- void record(int r, String n, int s) { //method
- rollno = r;
- name = n;
- salary = s;
- }
- void displayDetails() {
- System.out.println(rollno + " " + name + " " + salary);
- } //method
- public static void main(String args[]) {
- Employee e1 = new Employee();
- Employee e2 = new Employee();
- e1.record(1, "David", 10000);
- e2.record(2, "James", 25000);
- e1.displayDetails();
- e2.displayDetails();
- }
- }
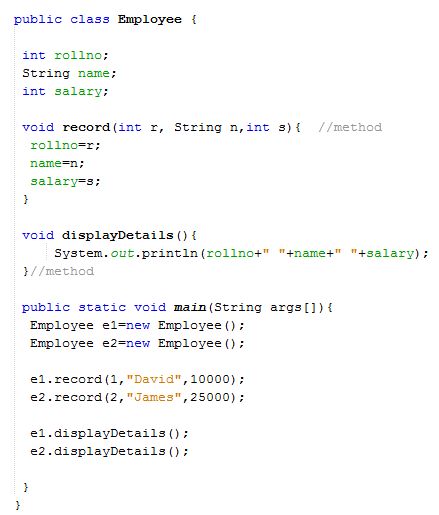
Output
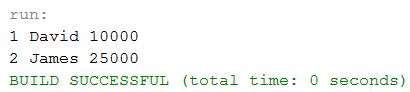
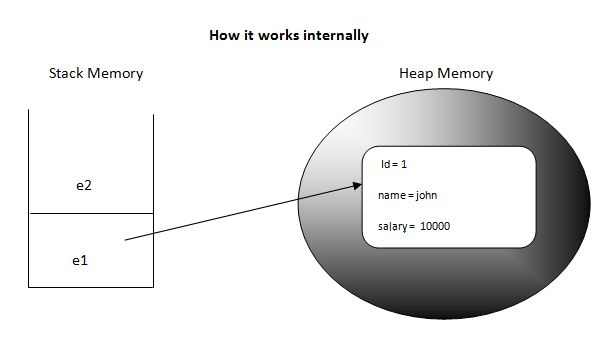
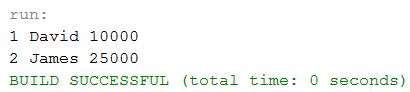
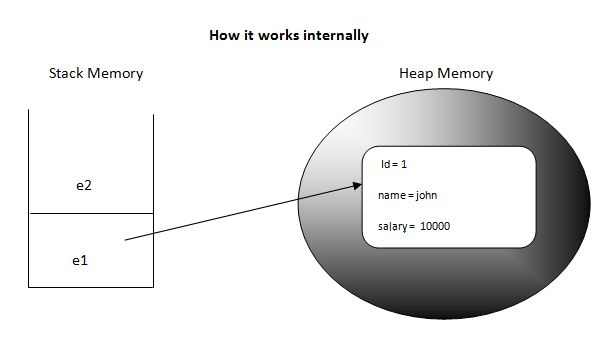
In the figure, mentioned above, an object takes the space in Heap memory area, e1 and e2 are the reference variables that refer to the object allocated in the memory and the reference variable refers to the allocated object in the heap memory area.
Summary
Thus, we learnt object and class are the basic techniques of an object oriented programming language and also learnt how to create a program, using object and class in Java.