JRadioButton Class In Java
JRadioButton Class
In Java, JRadioButton class creates a radio button and it is used to choose one option from the multiple options. It is commonly used in the exam systems or the quiz. It must be added in ButtonGroup to select one radio button only.
The JRadioButton class extends the JToggleButton class, which extends AbstractButton class.
Constructors of JRadioButton class
JRadioButton()
This constructor creates an unselected radio button with no text.
JRadioButton(String s)
This constructor creates a unselected radio button with the particular text.
JRadioButton(String s, boolean selected)
This constructor creates a radio button with the particular text and the selected status.
Methods of AbstractButton class
public void setText(String s)
This method is used to set the particular text on the button.
public String getText()
This method is used to return the text of the button.
public void setEnabled(boolean b)
This method is used to enable or disable the button.
public void setIcon(Icon b)
This method is used to set the particular Icon on the button.
public Icon getIcon()
This method is used to get the Icon of the button.
public void setMnemonic(int a)
This method is used to set the mnemonic on the button.
public void addActionListener(ActionListener a)
This method is used to add the action listener to the object.
Let’s see an example of JRadioButton class, given below.
Code
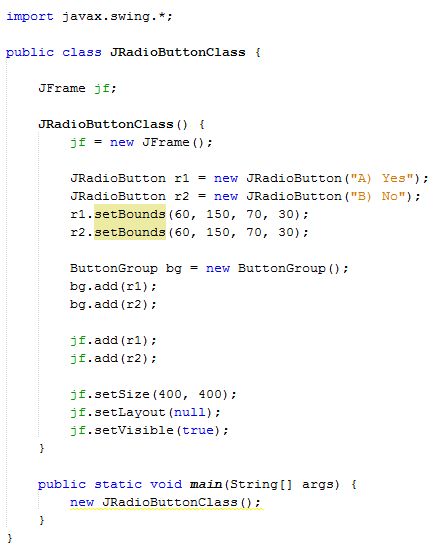
- import javax.swing.*;
- public class JRadioButtonClass {
- JFrame jf;
- JRadioButtonClass() {
- jf = new JFrame();
- JRadioButton r1 = new JRadioButton("A) Yes");
- JRadioButton r2 = new JRadioButton("B) No");
- r1.setBounds(60, 150, 70, 30);
- r2.setBounds(60, 150, 70, 30);
- ButtonGroup bg = new ButtonGroup();
- bg.add(r1);
- bg.add(r2);
- jf.add(r1);
- jf.add(r2);
- jf.setSize(400, 400);
- jf.setLayout(null);
- jf.setVisible(true);
- }
- public static void main(String[] args) {
- new JRadioButtonClass();
- }
- }
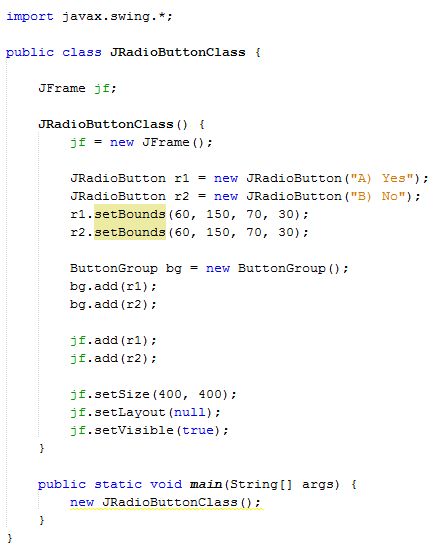
ButtonGroup class
ButtonGroup class is used to group multiple buttons. Thus, at a time, only one button can be selected.
Let’s see an example, given below, JRadioButton class with the event handling.
Code
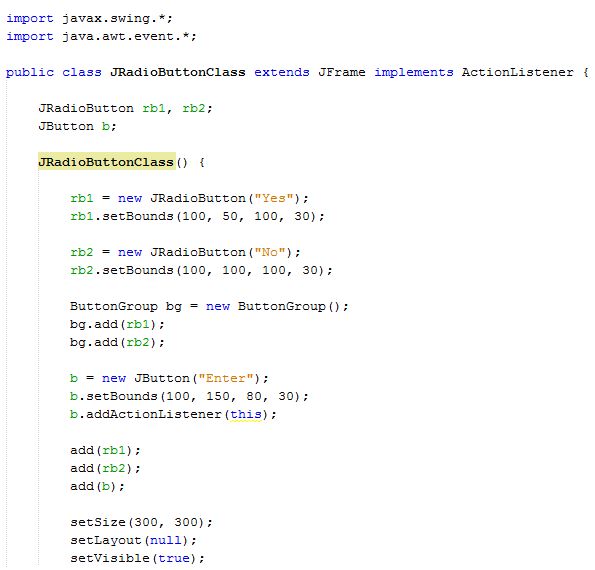
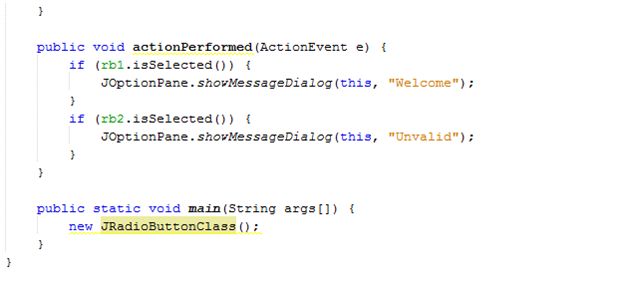
Output
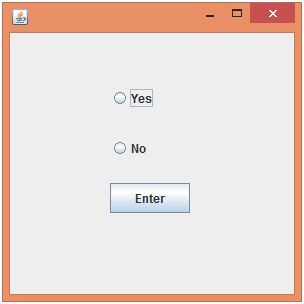
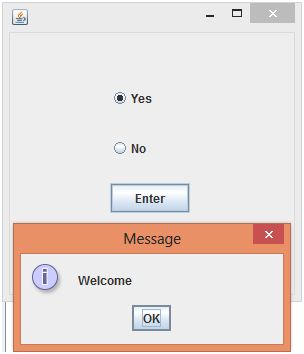
- import javax.swing.*;
- import java.awt.event.*;
- public class JRadioButtonClass extends JFrame implements ActionListener {
- JRadioButton rb1, rb2;
- JButton b;
- JRadioButtonClass() {
- rb1 = new JRadioButton("Yes");
- rb1.setBounds(100, 50, 100, 30);
- rb2 = new JRadioButton("No");
- rb2.setBounds(100, 100, 100, 30);
- ButtonGroup bg = new ButtonGroup();
- bg.add(rb1);
- bg.add(rb2);
- b = new JButton("Enter");
- b.setBounds(100, 150, 80, 30);
- b.addActionListener(this);
- add(rb1);
- add(rb2);
- add(b);
- setSize(300, 300);
- setLayout(null);
- setVisible(true);
- }
- public void actionPerformed(ActionEvent e) {
- if (rb1.isSelected()) {
- JOptionPane.showMessageDialog(this, "Welcome");
- }
- if (rb2.isSelected()) {
- JOptionPane.showMessageDialog(this, "Unvalid");
- }
- }
- public static void main(String args[]) {
- new JRadioButtonClass();
- }
- }
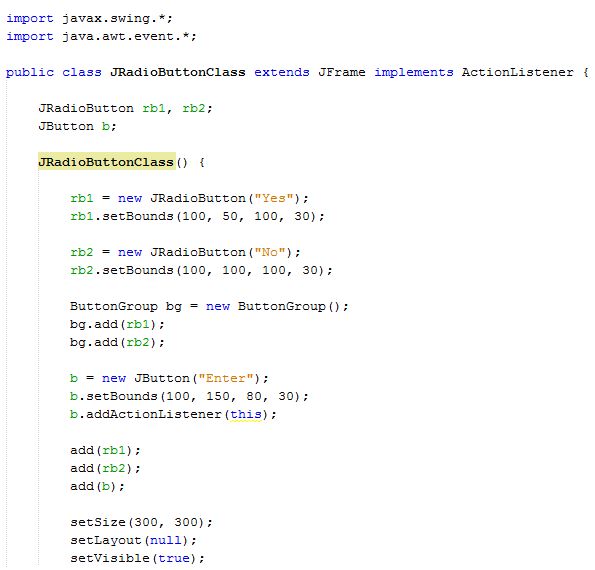
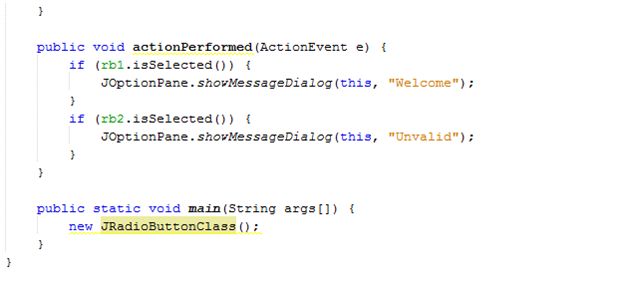
Output
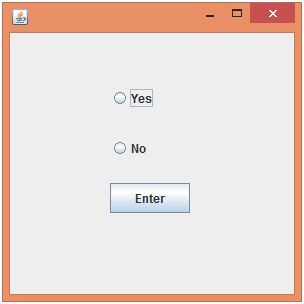
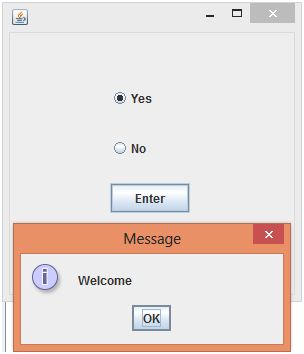
Summary
Thus, we learnt that JRadioButton class creates a radio button and it is used to choose one option from multiple options and also learnt how to use it in Java.