Comparator Interface In Java
Comparator Interface
In Java, comparator interface orders the objects of user-defined class. This interface is found in java.util package and it holds the two methods compare (Object obj1, Object obj2) and equals (Object element). It provides multiple sorting sequence i.e. we can sort the elements on the basis of any data member.
Method
public int compare(Object obj1,Object obj2)
This method is used to compare the first object with the second object.
Let’s see an example, given below.
Code
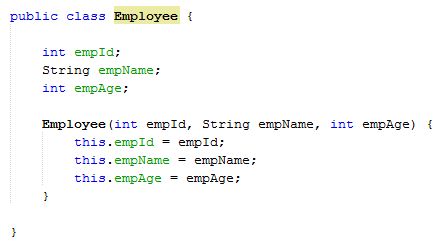
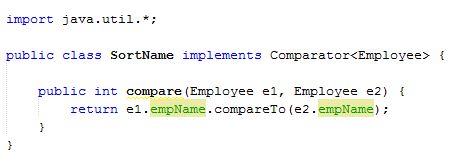
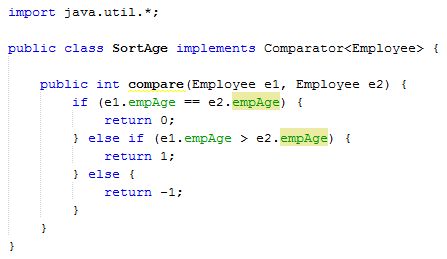
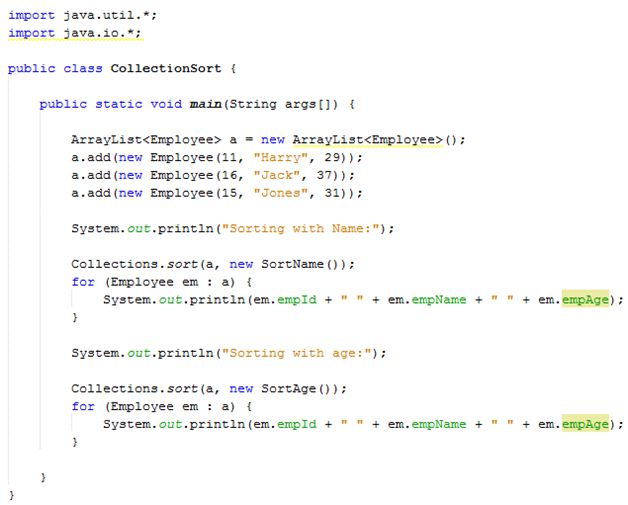
- public class Employee {
- int empId;
- String empName;
- int empAge;
- Employee(int empId, String empName, int empAge) {
- this.empId = empId;
- this.empName = empName;
- this.empAge = empAge;
- }
- }
- import java.util.*;
- public class SortName implements Comparator < Employee > {
- public int compare(Employee e1, Employee e2) {
- return e1.empName.compareTo(e2.empName);
- }
- }
- import java.util.*;
- public class SortAge implements Comparator < Employee > {
- public int compare(Employee e1, Employee e2) {
- if (e1.empAge == e2.empAge) {
- return 0;
- } else if (e1.empAge > e2.empAge) {
- return 1;
- } else {
- return -1;
- }
- }
- }
- import java.util.*;
- import java.io.*;
- public class CollectionSort {
- public static void main(String args[]) {
- ArrayList < Employee > a = new ArrayList < Employee > ();
- a.add(new Employee(11, "Harry", 29));
- a.add(new Employee(16, "Jack", 37));
- a.add(new Employee(15, "Jones", 31));
- System.out.println("Sorting with Name:");
- Collections.sort(a, new SortName());
- for (Employee em: a) {
- System.out.println(em.empId + " " + em.empName + " " + em.empAge);
- }
- System.out.println("Sorting with age:");
- Collections.sort(a, new SortAge());
- for (Employee em: a) {
- System.out.println(em.empId + " " + em.empName + " " + em.empAge);
- }
- }
- }
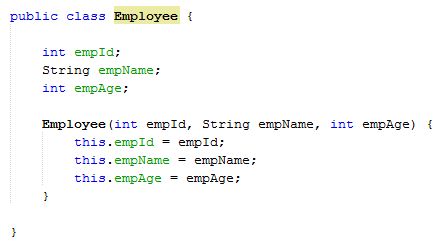
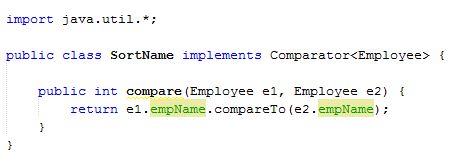
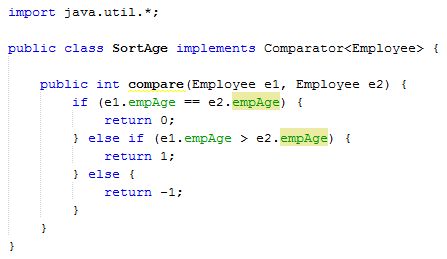
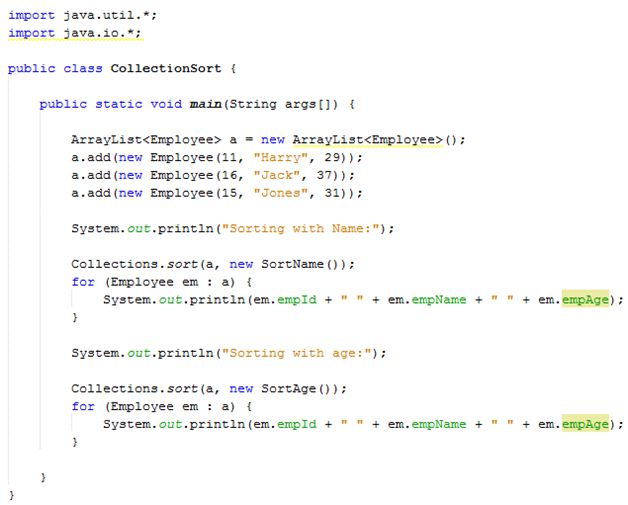
Output
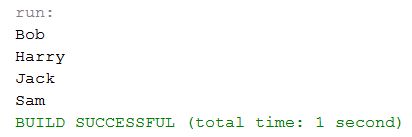
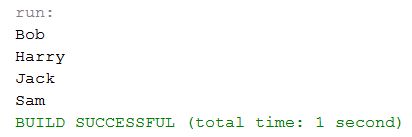
In the example, mentioned above, the classes provide the comparison logic, based on the name and age. In such case, we are using the compareTo() method of String class, which internally provides the comparison logic and then we are printing the objects values by sorting on the basis of the name and the age.
Summary
Thus, we learnt that Java comparator interface orders the objects of user-defined class. This interface is found in java.util package. It holds the two methods compare (Object obj1, Object obj2), equals (Object element) and also learnt how to create it in Java.