Call run() Method Directly Instead Of start() Method
If we call run() method directly instead of start() method
Every thread starts in a separate call stack.
Calling the run() method from the main thread, followed by the run() method goes on the present call stack rather than at the beginning of a new call stack.
Let’s understand with the example, given below.
Code
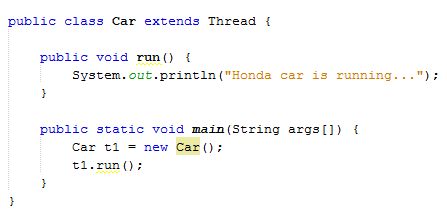
- public class Car extends Thread {
- public void run() {
- System.out.println("Honda car is running...");
- }
- public static void main(String args[]) {
- Car t1 = new Car();
- t1.run();
- }
- }
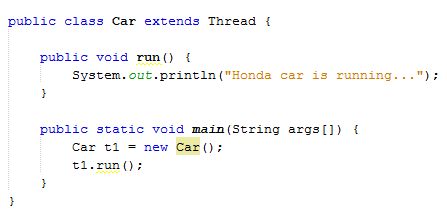
Output


In the above example, we observe that program run normally but it does not start a separate call stack.
Let’s see another example, given below, if we directly call run() method.
Code
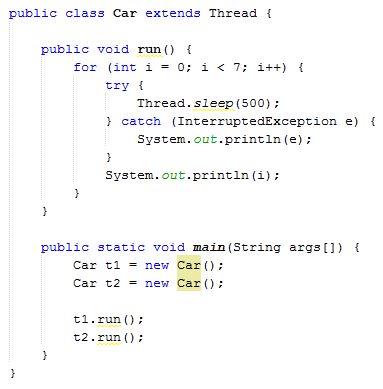
- public class Car extends Thread {
- public void run() {
- for (int i = 0; i < 7; i++) {
- try {
- Thread.sleep(500);
- } catch (InterruptedException e) {
- System.out.println(e);
- }
- System.out.println(i);
- }
- }
- public static void main(String args[]) {
- Car t1 = new Car();
- Car t2 = new Car();
- t1.run();
- t2.run();
- }
- }
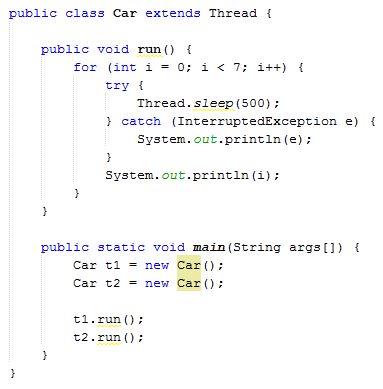
Output
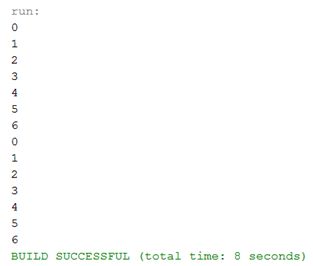
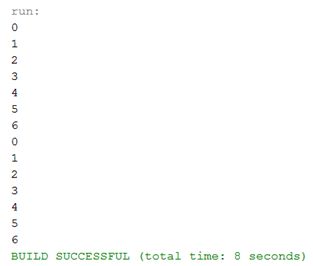
In the example, mentioned above, we can see, there is no context-switching because here t1 and t2 will treat as a normal object and not a thread object.
Summary
Thus, we learnt, if we call run() method directly instead of start() method, object treats it as a normal object and not a thread object in Java multithreading.