Autoboxing And Autounboxing In Java
Autoboxing and Autounboxing
In Java, autoboxing and autounboxing is the new feature of Java5. The automatic conversion of primitive data types into its equivalent wrapper type is called as autoboxing and the automatic conversion of wrapper class type into the corresponding primitive type means an opposite operation is called as autounboxing. Thus, programmer doesn't need to write the conversion code. There is no need of conversion between primitives and wrappers manually.
Let’s see an example of autoboxing, given below.
Code
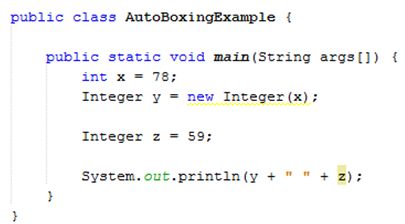
- public class AutoBoxingExample {
- public static void main(String args[]) {
- int x = 78;
- Integer y = new Integer(x);
- Integer z = 59;
- System.out.println(y + " " + z);
- }
- }
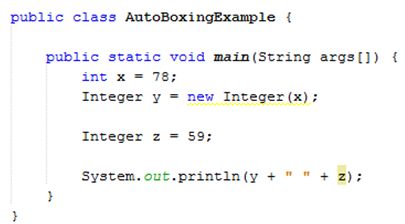
Output


Let’s see an example of autounboxing, given below.
Code
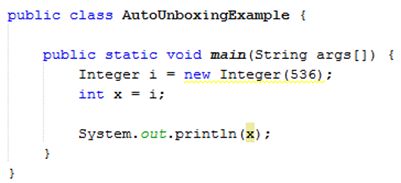
- public class AutoUnboxingExample {
- public static void main(String args[]) {
- Integer i = new Integer(536);
- int x = i;
- System.out.println(x);
- }
- }
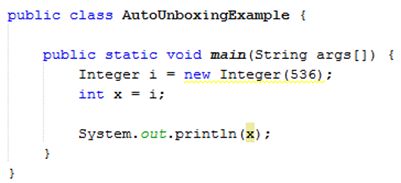
Output


Autoboxing and Autounboxing with comparison operators
Let’s see an example, given below.
Code
- public class AutoUnboxingExample {
- public static void main(String args[]) {
- Integer i = new Integer(53);
- if (i < 100) {
- System.out.println(i);
- }
- }
- }
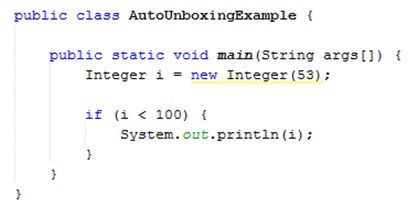
Output


Autoboxing and Autounboxing with method overloading
Autoboxing and autounboxing can be performed in method overloading but there are some rules for method overloading with autoboxing, which are,
- Widening beats boxing
- Widening beats varargs
- Boxing beats varargs
Let’s see an example of autoboxing, where widening beats boxing, given below.
Code
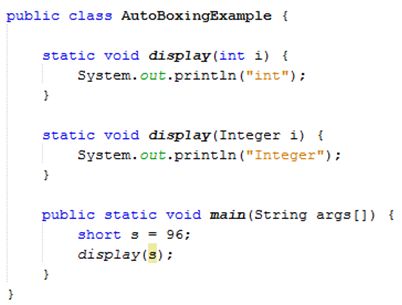
- public class AutoBoxingExample {
- static void display(int i) {
- System.out.println("int");
- }
- static void display(Integer i) {
- System.out.println("Integer");
- }
- public static void main(String args[]) {
- short s = 96;
- display(s);
- }
- }
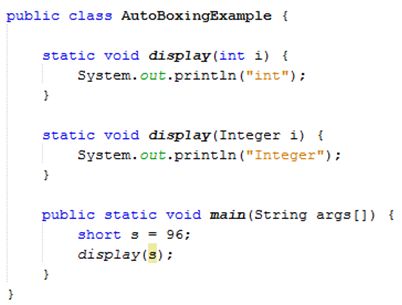
Output


Let’s see an example of autoboxing, where widening beats varargs are given below.
Code
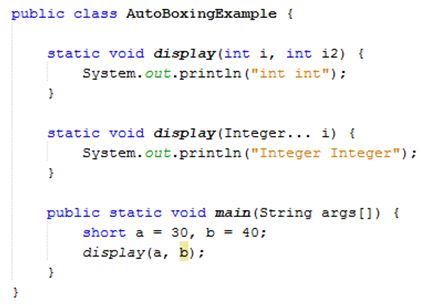
- public class AutoBoxingExample {
- static void display(int i, int i2) {
- System.out.println("int int");
- }
- static void display(Integer...i) {
- System.out.println("Integer Integer");
- }
- public static void main(String args[]) {
- short a = 30, b = 40;
- display(a, b);
- }
- }
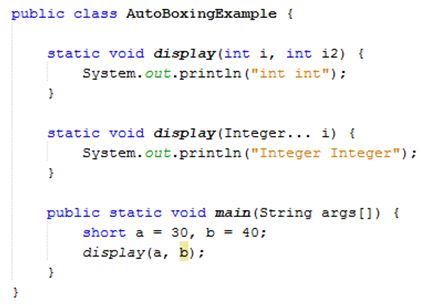
Output


Let’s see an example of autoboxing, where boxing beats varargs are given below.
Code
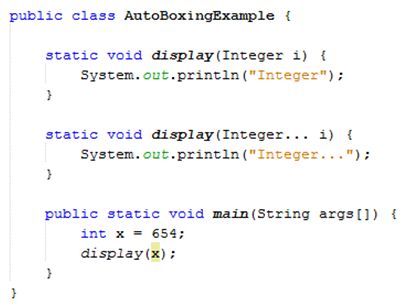
- public class AutoBoxingExample {
- static void display(Integer i) {
- System.out.println("Integer");
- }
- static void display(Integer...i) {
- System.out.println("Integer...");
- }
- public static void main(String args[]) {
- int x = 654;
- display(x);
- }
- }
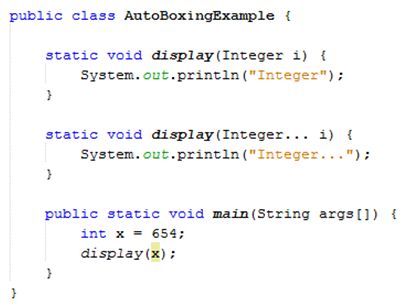
Output


Let’s see an example of method overloading with widening and boxing, given below.
Code
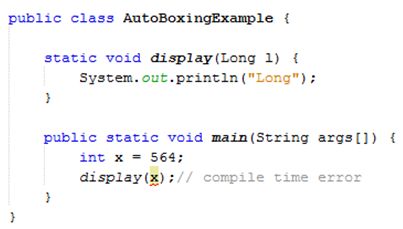
- public class AutoBoxingExample {
- static void display(Long l) {
- System.out.println("Long");
- }
- public static void main(String args[]) {
- int x = 564;
- display(x); // compile time error
- }
- }
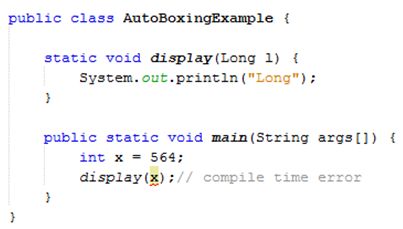
Summary
Thus, we learnt that the automatic conversion of the primitive data types into its equivalent wrapper type is called as autoboxing and opposite operation is called as autounboxing and also learnt how to use it in Java.