JColorChooser Class In Java
JColorChooser Class
In Java, JColorChooser class creates a color chooser dialog box. Thus, the user can select any color.
Constructors of JColorChooser class
JColorChooser()
This constructor is used to create a color chooser pane with White color initially.
JColorChooser(Color initialColor)
This constructor is used to create a color chooser pane with the particular color initially.
Methods of JColorChooser class
public static Color showDialog(Component c, String title, Color initialColor)
This method is used to show the color-chooser dialog box.
Let’s see an example of JColorChooser class, given below.
Code
- import java.awt.event.*;
- import java.awt.*;
- import javax.swing.*;
- public class JColorChooserExample extends JFrame implements ActionListener {
- JButton jb;
- Container c;
- JColorChooserExample() {
- c = getContentPane();
- c.setLayout(new FlowLayout());
- jb = new JButton("Color");
- jb.addActionListener(this);
- c.add(jb);
- }
- public void actionPerformed(ActionEvent e) {
- Color initialcolor = Color.GREEN;
- Color col = JColorChooser.showDialog(this, "Choose a color", initialcolor);
- c.setBackground(col);
- }
- public static void main(String[] args) {
- JColorChooserExample jch = new JColorChooserExample();
- jch.setSize(400, 400);
- jch.setVisible(true);
- jch.setDefaultCloseOperation(EXIT_ON_CLOSE);
- }
- }
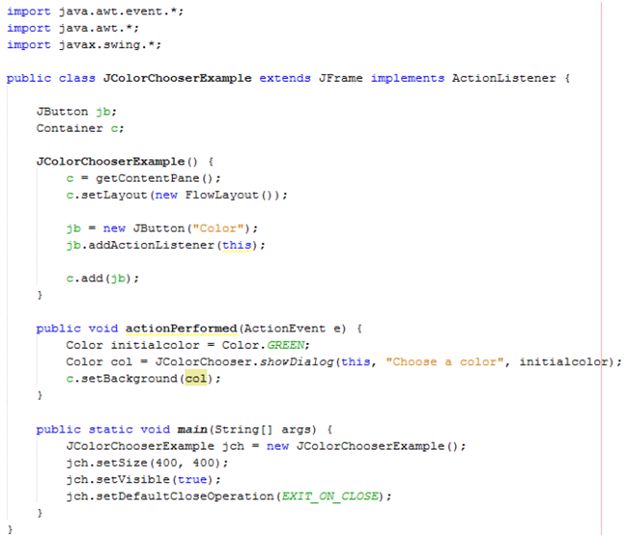
Output
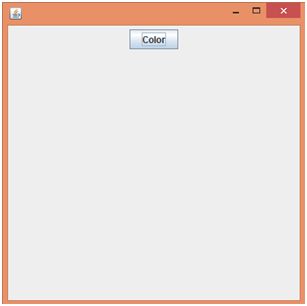
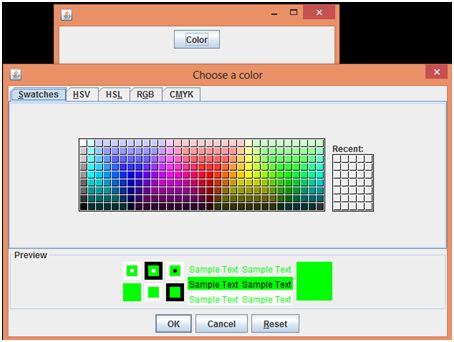
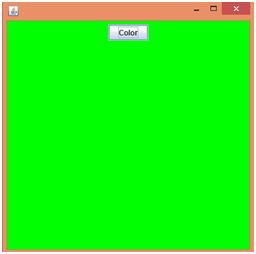
Summary
Thus, we learnt that the JColorChooser class creates a color chooser dialog box. Thus, the user can select any color and we also learnt how to use it in Java.