toString() Method In Java
toString()
The toString() method is used to return the string representation of the object.
When we want to print any object, Java compiler internally invokes the toString() method on the object. Hence, the toString() method overrides and returns the desired output. It can be the state of an object and it depends on our implementation.
Advantages of toString() method in Java
With overriding, the toString() method of the Object class, we can return the values of the object and we don't need to write much code. It saves time also.
Let’s see the problem without using toString() method in Java.
Code
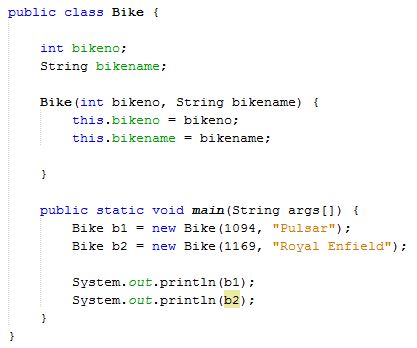
- public class Bike {
- int bikeno;
- String bikename;
- Bike(int bikeno, String bikename) {
- this.bikeno = bikeno;
- this.bikename = bikename;
- }
- public static void main(String args[]) {
- Bike b1 = new Bike(1094, "Pulsar");
- Bike b2 = new Bike(1169, "Royal Enfield");
- System.out.println(b1);
- System.out.println(b2);
- }
- }
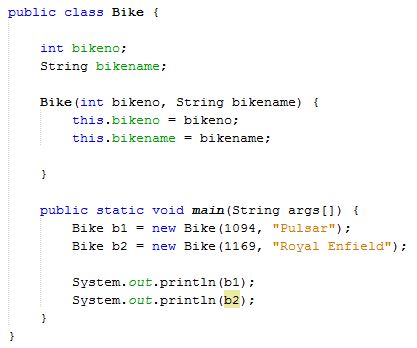
Output
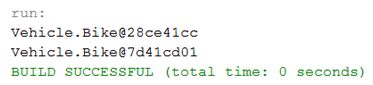
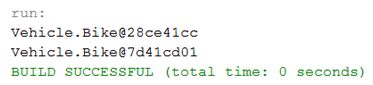
In the example, shown above, we can see that b1 and b2 prints the hashcode values of the objects but we want to print the values of these objects. Java compiler internally invokes toString() method and overriding this method will return the particular values of the program.
Now, let's see the example of toString() method.
Code
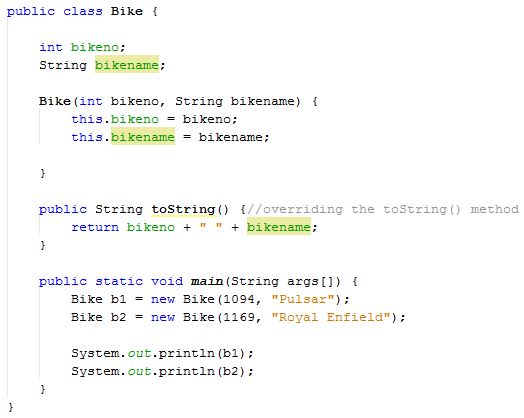
- public class Bike {
- int bikeno;
- String bikename;
- Bike(int bikeno, String bikename) {
- this.bikeno = bikeno;
- this.bikename = bikename;
- }
- public String toString() { //overriding the toString() method
- return bikeno + " " + bikename;
- }
- public static void main(String args[]) {
- Bike b1 = new Bike(1094, "Pulsar");
- Bike b2 = new Bike(1169, "Royal Enfield");
- System.out.println(b1);
- System.out.println(b2);
- }
- }
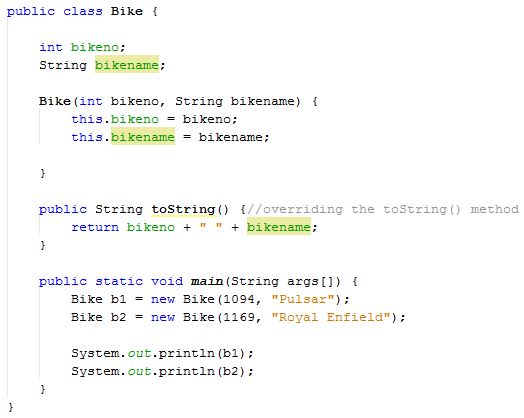
Output
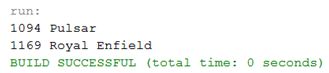
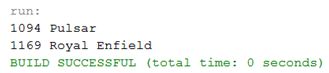
In the example, shown above, we can see that with overriding, the toString() method, we can get the desired output of the code due to which we use toString() method in Java.
Summary
Thus, we learnt that toString() method is used to return the string representation of the object in Java and also learnt their main advantages in Java.