Statement Interface In Java
Statement Interface
In JDBC, the Statement interface provides methods to execute queries with the database. This interface is a factory of ResultSet and it provides factory method to get the object of ResultSet.
Methods of Statement interface
public ResultSet executeQuery(String sql)
This method is used to execute SELECT query. It returns the object of ResultSet.
public int executeUpdate(String sql)
This method is used to execute particular query, it may be create, drop, insert, update, delete etc.
public boolean execute(String sql)
This method is used to execute queries that may return multiple results.
public int[] executeBatch()
This method is used to execute batch of commands.
Let’s see an example1, given below.
Code
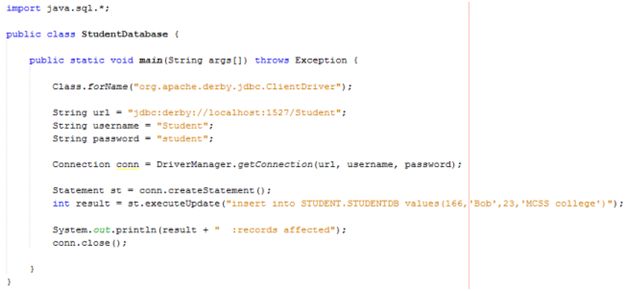
- import java.sql.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- Statement st = conn.createStatement();
- int result = st.executeUpdate("insert into STUDENT.STUDENTDB values(166,'Bob',23,'MCSS college')");
- System.out.println(result + " :records affected");
- conn.close();
- }
- }
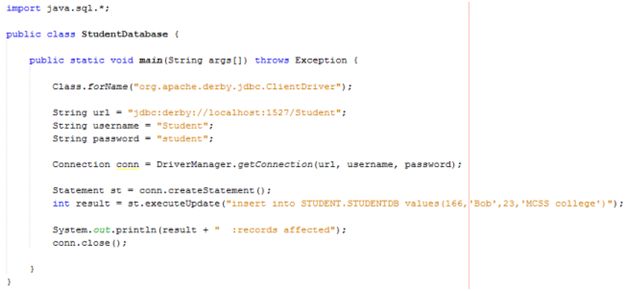
Output


Let’s see an example2, given below.
Code
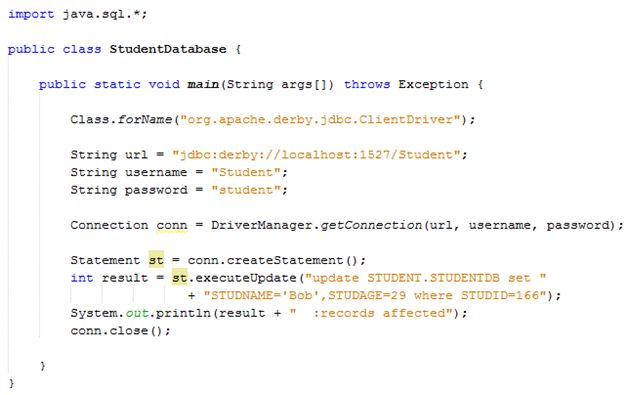
- import java.sql.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- Statement st = conn.createStatement();
- int result = st.executeUpdate("update STUDENT.STUDENTDB set STUDNAME='Bob',STUDAGE=29 where STUDID=166");
- System.out.println(result + " :records affected");
- conn.close();
- }
- }
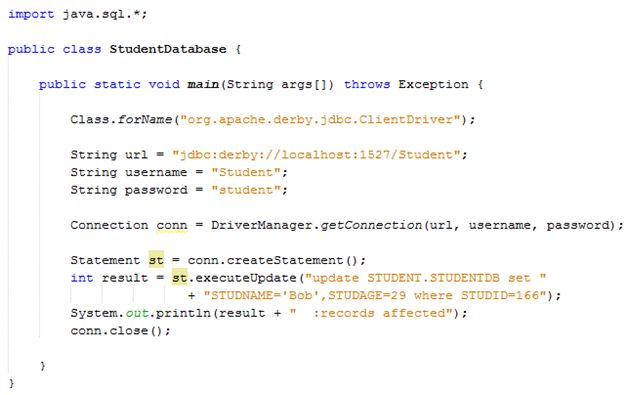
Output


Let’s see an example3, given below.
Code
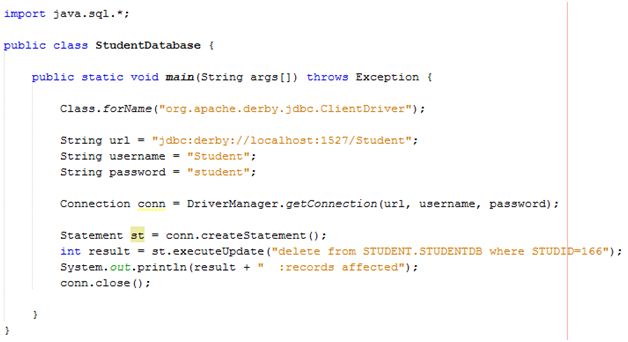
- import java.sql.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- Statement st = conn.createStatement();
- int result = st.executeUpdate("delete from STUDENT.STUDENTDB where STUDID=166");
- System.out.println(result + " :records affected");
- conn.close();
- }
- }
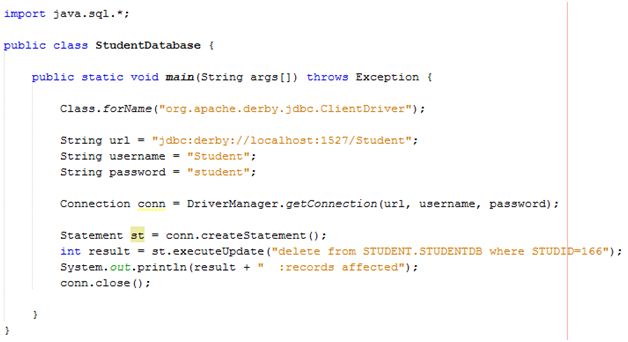
Output


The three examples of Statement interface are mentioned above to insert, update and delete the record.
Summary
Thus, we learned that JDBC Statement interface provides methods to execute queries with the database. This interface is a factory of ResultSet and it provides factory method to get the object of ResultSet and also learn its important methods in Java.