Batch Processing In JDBC
Batch Processing
In JDBC, we can execute a batch (group) of queries instead of executing a single query.
The java.sql.Statement interface and java.sql.PreparedStatement interface provides methods for batch processing.
Advantage of Batch Processing
Fast Performance
it is mainly used to make the performance fast.
Methods of Statement interface
The necessary methods for batch processing are,
void addBatch(String query)
This method is used to add a query into a batch.
int[] executeBatch()
This method is used to execute the batch of queries.
Let's see an example of batch processing in JDBC.
Code
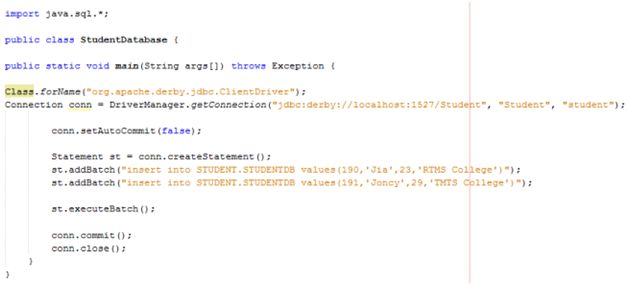
- import java.sql.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- Connection conn = DriverManager.getConnection("jdbc:derby://localhost:1527/Student", "Student", "student");
- conn.setAutoCommit(false);
- Statement st = conn.createStatement();
- st.addBatch("insert into STUDENT.STUDENTDB values(190,'Jia',23,'RTMS College')");
- st.addBatch("insert into STUDENT.STUDENTDB values(191,'Joncy',29,'TMTS College')");
- st.executeBatch();
- conn.commit();
- conn.close();
- }
- }
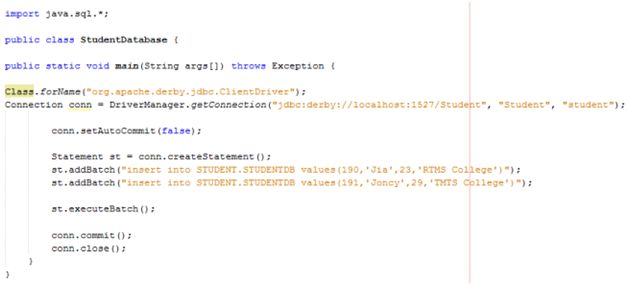
If we see the table student, two records has been added.
Let’s see an example of batch processing using PreparedStatement.
Code
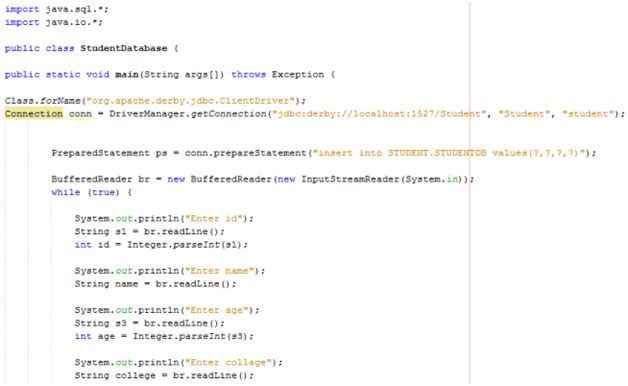
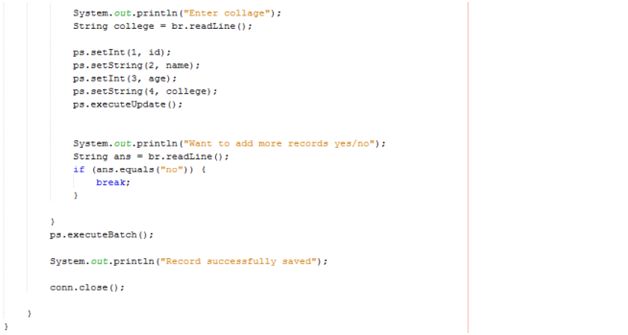
- import java.sql.*;
- import java.io.*;
- public class StudentDatabase {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- Connection conn = DriverManager.getConnection("jdbc:derby://localhost:1527/Student", "Student", "student");
- PreparedStatement ps = conn.prepareStatement("insert into STUDENT.STUDENTDB values(?,?,?,?)");
- BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
- while (true) {
- System.out.println("Enter id");
- String s1 = br.readLine();
- int id = Integer.parseInt(s1);
- System.out.println("Enter name");
- String name = br.readLine();
- System.out.println("Enter age");
- String s3 = br.readLine();
- int age = Integer.parseInt(s3);
- System.out.println("Enter collage");
- String college = br.readLine();
- ps.setInt(1, id);
- ps.setString(2, name);
- ps.setInt(3, age);
- ps.setString(4, college);
- ps.executeUpdate();
- System.out.println("Want to add more records yes/no");
- String ans = br.readLine();
- if (ans.equals("no")) {
- break;
- }
- }
- ps.executeBatch();
- System.out.println("Record successfully saved");
- conn.close();
- }
- }
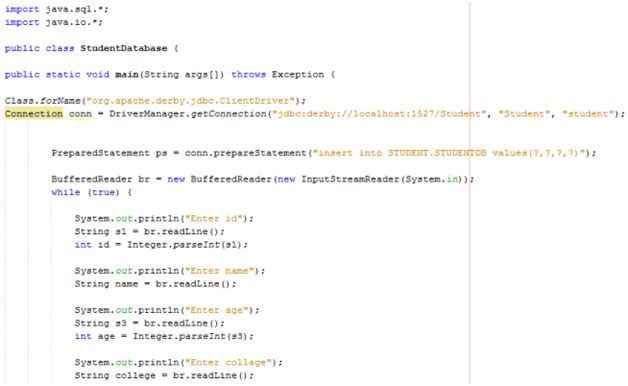
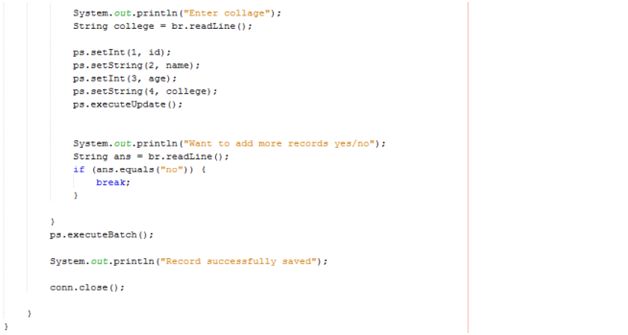
Output
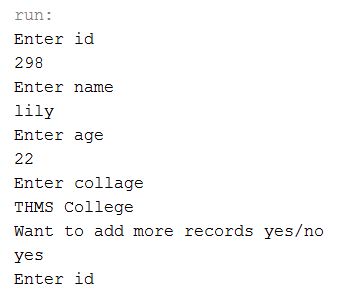
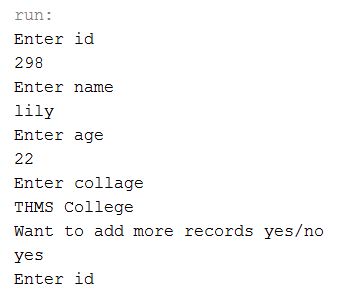
In the example stated above, it will add the queries into the batch until the user presses no. Finally, it executes the batch. Thus, all the added queries will be fired.
Summary
Thus, we learnt, in JDBC, we can execute a batch (group) of queries instead of executing a single query and also learn how we can use it in Java.