JComboBox Class In Java
JComboBox Class
In Java, JComboBox class creates the combobox and only one item can be selected from the item list at a time.
Constructors of JComboBox class
- JComboBox()
- JComboBox(Object[] items)
- JComboBox(Vector<?> items)
Methods of JComboBox class
public void addItem(Object anObject)
This method is used to add an item to the item list.
public void removeItem(Object anObject)
This method is used to delete an item from the item list.
public void removeAllItems()
This method is used to remove all the items from the list.
public void setEditable(boolean b)
This method is used to determine whether the JComboBox is editable or not.
public void addActionListener(ActionListener a)
This method is used to add the ActionListener.
public void addItemListener(ItemListener i)
This method is used to add the ItemListener.
Let’s see an example of JcomboBox class, given below.
Code
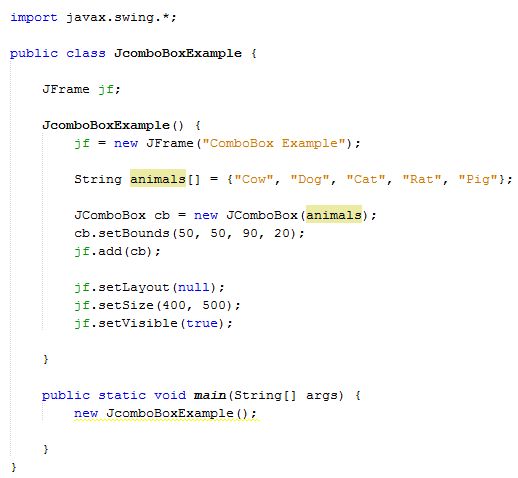
- import javax.swing.*;
- public class JcomboBoxExample {
- JFrame jf;
- JcomboBoxExample() {
- jf = new JFrame("ComboBox Example");
- String animals[] = {"Cow", "Dog", "Cat", "Rat", "Pig"};
- JComboBox cb = new JComboBox(animals);
- cb.setBounds(50, 50, 90, 20);
- jf.add(cb);
- jf.setLayout(null);
- jf.setSize(400, 400);
- jf.setVisible(true);
- }
- public static void main(String[] args) {
- new JcomboBoxExample();
- }
- }
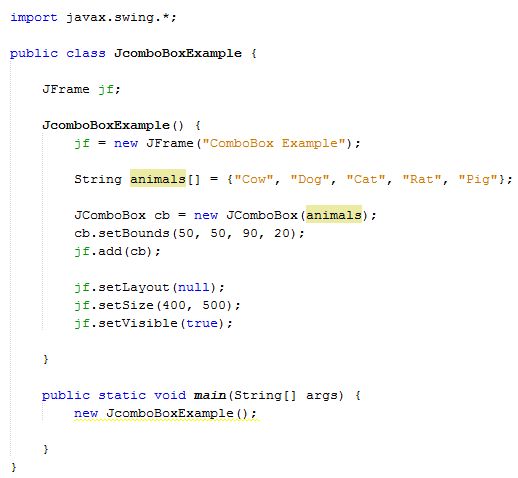
Output
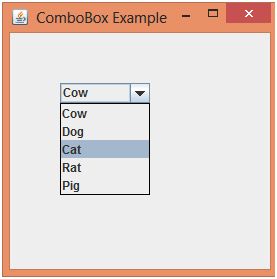
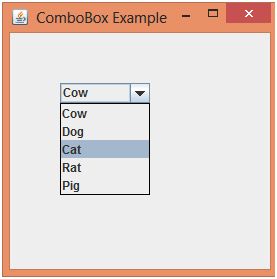
Summary
Thus, we learnt that the JComboBox class creates the combobox and only one item can be selected from the item list at a time only and also learnt how to use it in Java.