New Date And Time API
A new Date-Time API is introduced to cover the drawbacks of old date-time API like
- java.util.Date is not thread safe. Thus, concurrency issue arises, while using the date but the new date-time API is immutable and does not have setter methods.
- Old API had less direct methods for the date operations but new API provides various utility methods for such operations.
- We need to write a lot of code to deal with timezone issues but the new API has been developed keeping domain-specific design in mind.
A new date-time API is under the package java.time. There are some important classes, introduced in java.time package.
- Local − Simplify date-time API with no complexity of timezone handling.
- Zoned − Specialize date-time API to deal with different timezones.
Local Data-Time API
LocalDate/LocalTime class and LocalDateTime class make the development simple, where the timezones are not required.
Let’s see an example of Local Data-Time, given below.
Code
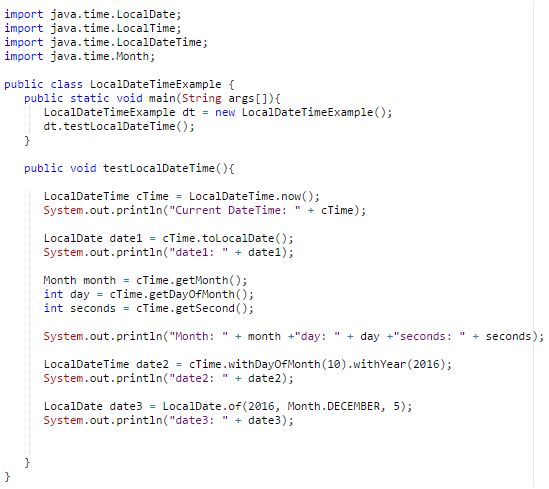
- import java.time.LocalDate;
- import java.time.LocalTime;
- import java.time.LocalDateTime;
- import java.time.Month;
- public class LocalDateTimeExample {
- public static void main(String args[]) {
- LocalDateTimeExample dt = new LocalDateTimeExample();
- dt.testLocalDateTime();
- }
- public void testLocalDateTime() {
- LocalDateTime cTime = LocalDateTime.now();
- System.out.println("Current DateTime: " + cTime);
- LocalDate date1 = cTime.toLocalDate();
- System.out.println("date1: " + date1);
- Month month = cTime.getMonth();
- int day = cTime.getDayOfMonth();
- int seconds = cTime.getSecond();
- System.out.println("Month: " + month + "day: " + day + "seconds: " + seconds);
- LocalDateTime date2 = cTime.withDayOfMonth(10).withYear(2016);
- System.out.println("date2: " + date2);
- LocalDate date3 = LocalDate.of(2016, Month.DECEMBER, 5);
- System.out.println("date3: " + date3);
- }
- }
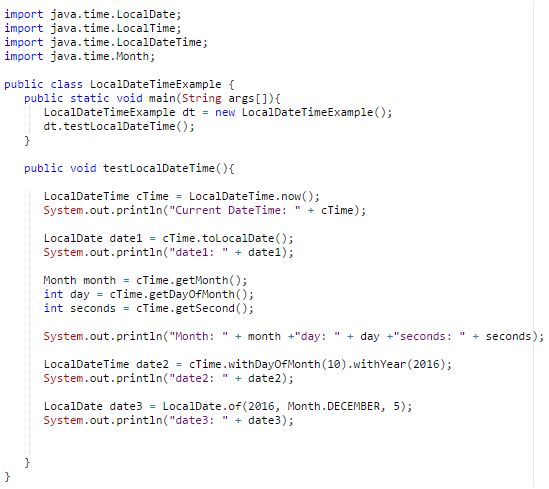
Output
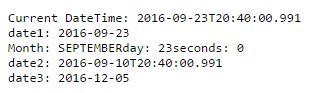
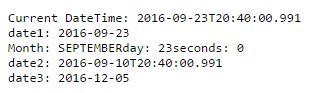
Zoned Date-Time API
Zoned date-time API is used, if time zone is to be considered.
Let’s see an example of Zoned date-time, given below.
Code
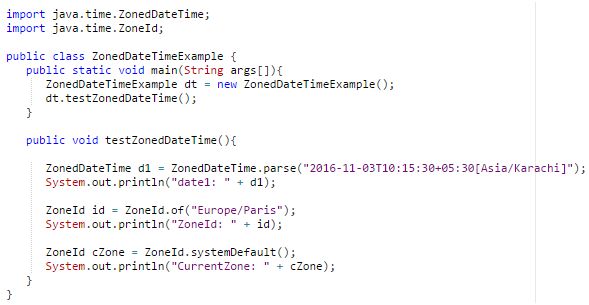
- import java.time.ZonedDateTime;
- import java.time.ZoneId;
- public class ZonedDateTimeExample {
- public static void main(String args[]) {
- ZonedDateTimeExample dt = new ZonedDateTimeExample();
- dt.testZonedDateTime();
- }
- public void testZonedDateTime() {
- ZonedDateTime d1 = ZonedDateTime.parse("2016-11-03T10:15:30+05:30[Asia/Karachi]");
- System.out.println("date1: " + d1);
- ZoneId id = ZoneId.of("Europe/Paris");
- System.out.println("ZoneId: " + id);
- ZoneId cZone = ZoneId.systemDefault();
- System.out.println("CurrentZone: " + cZone);
- }
- }
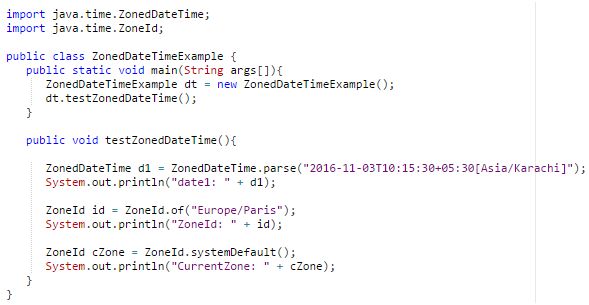
Output


Chrono Units Enum
java.time.temporal.ChronoUnit enum added new to replace the integer values, used in old API to represent day, month, etc.
Let’s see an example of Chrono Units Enum, given below.
Code
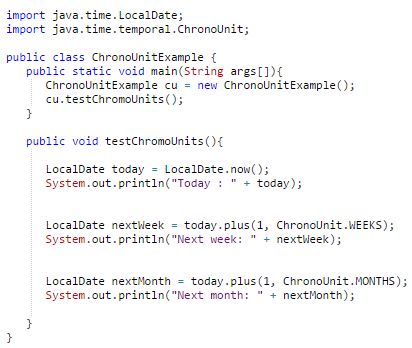
- import java.time.LocalDate;
- import java.time.temporal.ChronoUnit;
- public class ChronoUnitExample {
- public static void main(String args[]) {
- ChronoUnitExample cu = new ChronoUnitExample();
- cu.testChromoUnits();
- }
- public void testChromoUnits() {
- LocalDate today = LocalDate.now();
- System.out.println("Today : " + today);
- LocalDate nextWeek = today.plus(1, ChronoUnit.WEEKS);
- System.out.println("Next week: " + nextWeek);
- LocalDate nextMonth = today.plus(1, ChronoUnit.MONTHS);
- System.out.println("Next month: " + nextMonth);
- }
- }
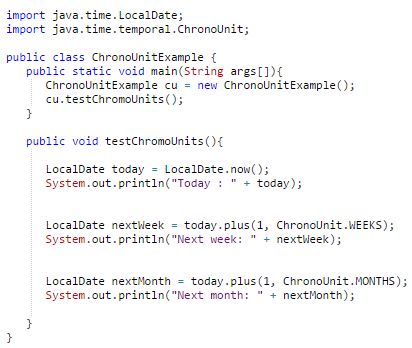
Output
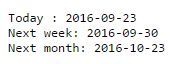
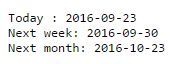
Period & Duration
In Java 8, two classes are dealt with the time differences.
Period – This class deals with date base and time.
Duration − This class deal with time base and amount of time.
Let’s see an example, given below.
Code
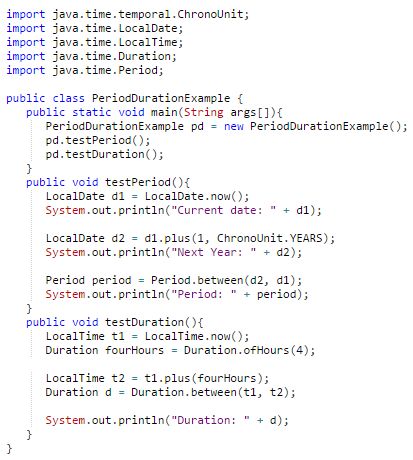
- import java.time.temporal.ChronoUnit;
- import java.time.LocalDate;
- import java.time.LocalTime;
- import java.time.Duration;
- import java.time.Period;
- public class PeriodDurationExample {
- public static void main(String args[]) {
- PeriodDurationExample pd = new PeriodDurationExample();
- pd.testPeriod();
- pd.testDuration();
- }
- public void testPeriod() {
- LocalDate d1 = LocalDate.now();
- System.out.println("Current date: " + d1);
- LocalDate d2 = d1.plus(1, ChronoUnit.YEARS);
- System.out.println("Next Year: " + d2);
- Period period = Period.between(d2, d1);
- System.out.println("Period: " + period);
- }
- public void testDuration() {
- LocalTime t1 = LocalTime.now();
- Duration fourHours = Duration.ofHours(4);
- LocalTime t2 = t1.plus(fourHours);
- Duration d = Duration.between(t1, t2);
- System.out.println("Duration: " + d);
- }
- }
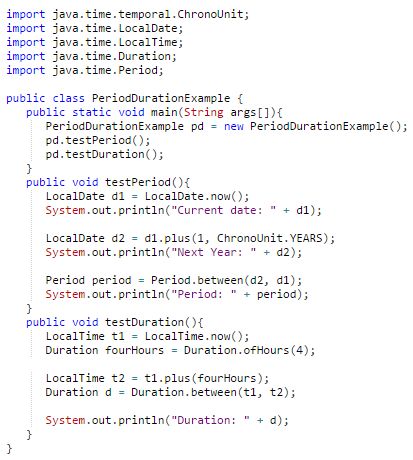
Output
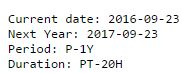
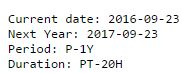
Temporal Adjusters
Temporal Adjuster performs the date mathematics.
For example, get the "Third Monday of the Month" or "Next Friday".
Let’s see an example of Temporal Adjuster, given below.
Code
- import java.time.LocalDate;
- import java.time.temporal.TemporalAdjusters;
- import java.time.DayOfWeek;
- public class TemporalAdjustersExample {
- public static void main(String args[]) {
- TemporalAdjustersExample ta = new TemporalAdjustersExample();
- ta.testAdjusters();
- }
- public void testAdjusters() {
- LocalDate d1 = LocalDate.now();
- System.out.println("Current date: " + d1);
- LocalDate nextFri = d1.with(TemporalAdjusters.next(DayOfWeek.FRIDAY));
- System.out.println("Next Friday on : " + nextFri);
- LocalDate firstInYear = LocalDate.of(d1.getYear(), d1.getMonth(), 1);
- LocalDate secondSun = firstInYear.with(TemporalAdjusters.nextOrSame(DayOfWeek.SUNDAY)).with(TemporalAdjusters.next(DayOfWeek.SUNDAY));
- System.out.println("Second Sunday on : " + secondSun);
- }
- }
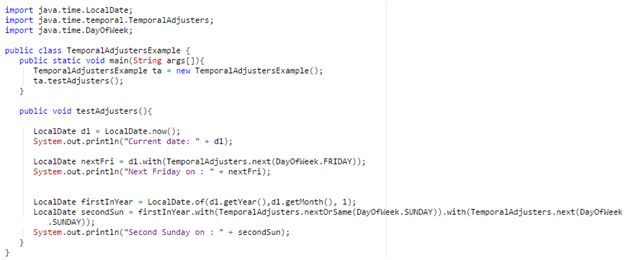
Output
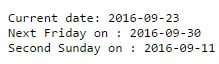
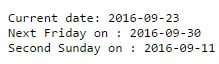
Backward Compatibility
A toInstant() method is used to add to the original date and calendar objects, which can be used to convert them to the new Date-Time API and ofInstant(Insant,ZoneId) method is used to get a LocalDateTime or ZonedDateTime object.
Let’s see an example of backward compatibility, given below.
Code
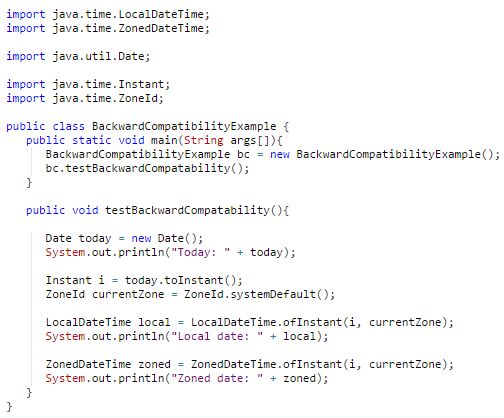
- import java.time.LocalDateTime;
- import java.time.ZonedDateTime;
- import java.util.Date;
- import java.time.Instant;
- import java.time.ZoneId;
- public class BackwardCompatibilityExample {
- public static void main(String args[]) {
- BackwardCompatibilityExample bc = new BackwardCompatibilityExample();
- bc.testBackwardCompatability();
- }
- public void testBackwardCompatability() {
- Date today = new Date();
- System.out.println("Today: " + today);
- Instant i = today.toInstant();
- ZoneId currentZone = ZoneId.systemDefault();
- LocalDateTime local = LocalDateTime.ofInstant(i, currentZone);
- System.out.println("Local date: " + local);
- ZonedDateTime zoned = ZonedDateTime.ofInstant(i, currentZone);
- System.out.println("Zoned date: " + zoned);
- }
- }
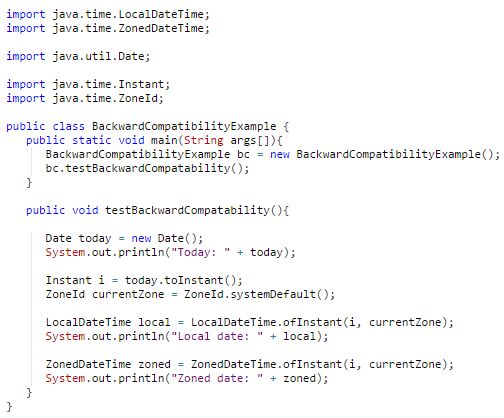
Output


Summary
Thus, we learnt that a new Date-Time API is introduced to cover the drawbacks of old date-time API and also learnt how to use it in Java.