Super Keyword In Java
Super Keyword
In Java, “Super” keyword is a reference variable, which is used to refer current parent class object.
Whenever we create the instance of subclass at the same time, an instance of parent class is also created implicitly by JVM.
Usage of “super” keyword in Java
- “Super” keyword is used to refer the current parent class instance variable.
- Super () keyword is used to call the current parent class constructor.
- Super keyword is used to call the current parent class method.
Super keyword is used to refer current parent class instance variable.
In Java, “super” keyword is used to refer current parent class instance variable.
Let’s see an example, given below.
Code
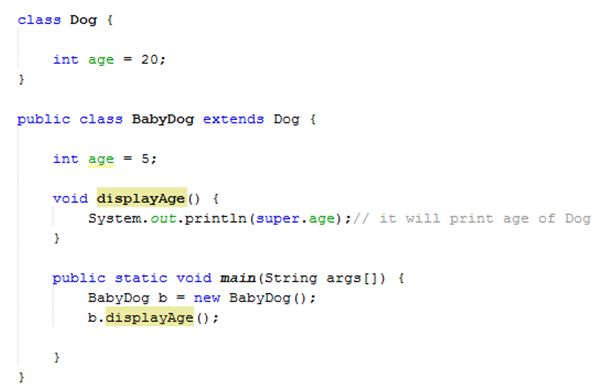
- class Dog {
- int age = 20;
- }
- public class BabyDog extends Dog {
- int age = 5;
- void displayAge() {
- System.out.println(super.age); // it will print age of Dog
- }
- public static void main(String args[]) {
- BabyDog b = new BabyDog();
- b.displayAge();
- }
- }
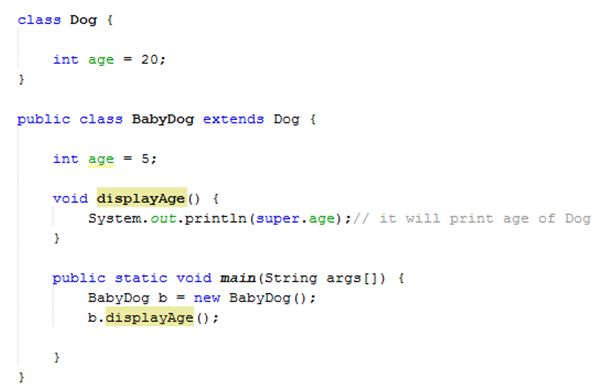
Output


In the example, mentioned above, Dog and BabyDog both class have a common property age. Instance variable of current (child) class is refered bydefault, but we have to refer parent class instance variable due to which we use super keyword to differentiate between parent class instance variable and current class instance variable.
If we don’t use super keyword in program, let’s see an example, given below.
Code
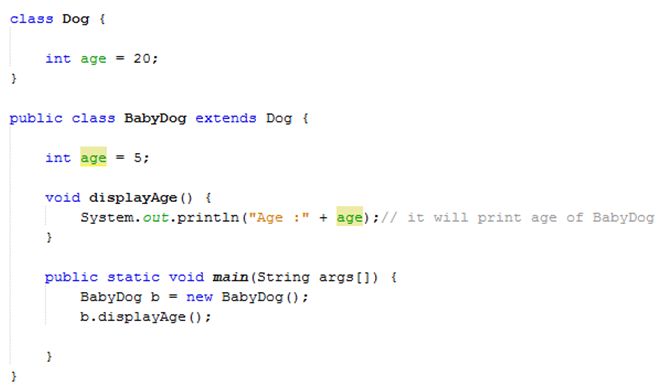
- class Dog {
- int age = 20;
- }
- public class BabyDog extends Dog {
- int age = 5;
- void displayAge() {
- System.out.println("Age :" + age); // it will print age of BabyDog
- }
- public static void main(String args[]) {
- BabyDog b = new BabyDog();
- b.displayAge();
- }
- }
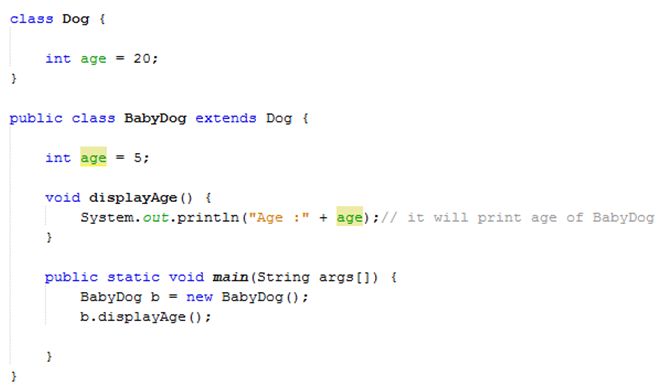
Output


Super () keyword is used to call current parent class constructor.
In Java, super () keyword is also used to call current parent class constructor.
Let’s see an example, given below.
Code
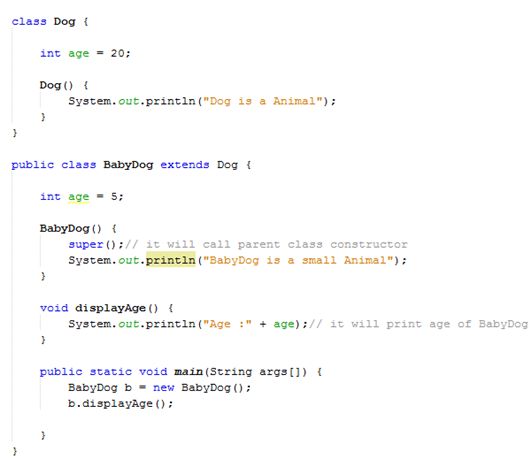
- class Dog {
- int age = 20;
- Dog() {
- System.out.println("Dog is a Animal");
- }
- }
- public class BabyDog extends Dog {
- int age = 5;
- BabyDog() {
- super(); // it will call parent class constructor
- System.out.println("BabyDog is a small Animal");
- }
- void displayAge() {
- System.out.println("Age :" + age); // it will print age of BabyDog
- }
- public static void main(String args[]) {
- BabyDog b = new BabyDog();
- b.displayAge();
- }
- }
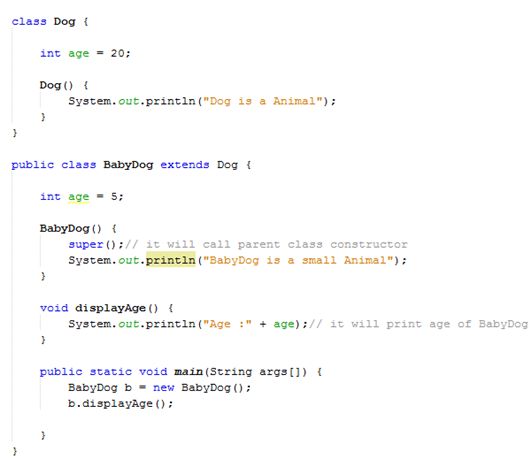
Output
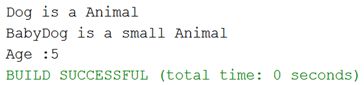
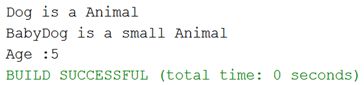
Super() is added in each and every class constructor automatically by compiler.
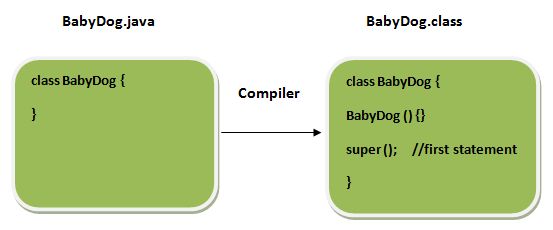
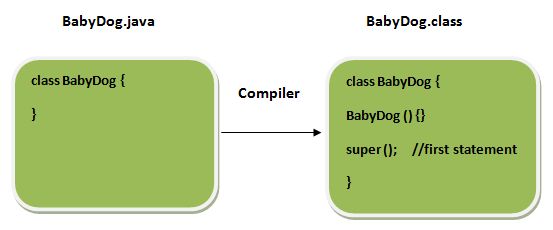
In the example, shown above, we create a constructor and use super keyword as the first statement to call a current parent class constructor. We know that the default constructor is provided by the compiler. We don’t have to need to write super ().
Super keyword is used to call current parent class method.
In Java, super keyword is also used to call parent class method.
Let’s see an example, given below.
Code
- class Dog {
- void eat() {
- System.out.println("Dog eat veg and nonveg both");
- }
- }
- public class BabyDog extends Dog {
- void eat() {
- System.out.println("BabyDog drink milk only");
- }
- void displayMsg() {
- eat(); //it will call current class eat() method
- super.eat(); // it will call parent class eat method
- }
- public static void main(String args[]) {
- BabyDog b = new BabyDog();
- b.displayMsg();
- }
- }
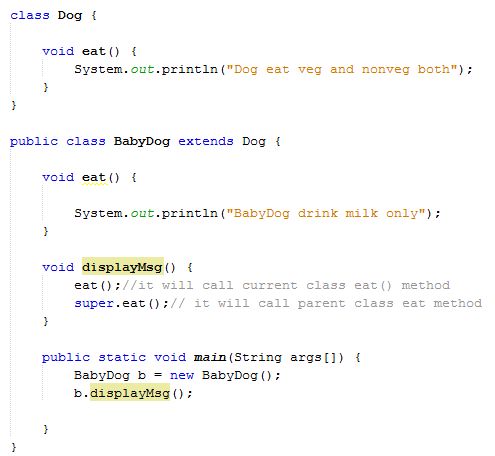
Output
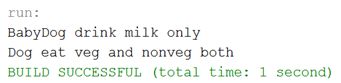
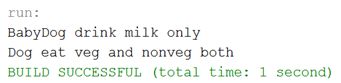
In the example, shown above, Dog and BabyDog are both the classes, which have same method eat() and if we call eat method from BabyDog class, it will call the eat() method of BabyDog class and not of Dog class because it gives priority to local class. In case, there is no method in subclass as parent, there is no need to use super.
Let’s see an example, given below, if there is no method in subclass as parent.
Code
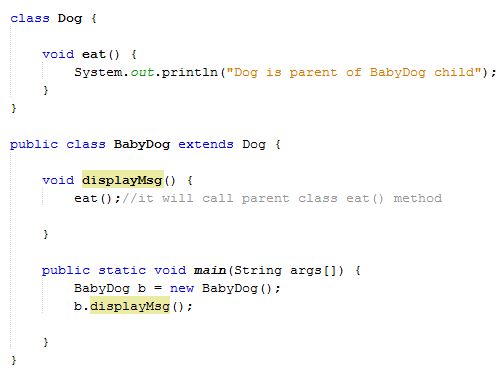
- class Dog {
- void eat() {
- System.out.println("Dog is parent of BabyDog child");
- }
- }
- public class BabyDog extends Dog {
- void displayMsg() {
- eat(); //it will call parent class eat() method
- }
- public static void main(String args[]) {
- BabyDog b = new BabyDog();
- b.displayMsg();
- }
- }
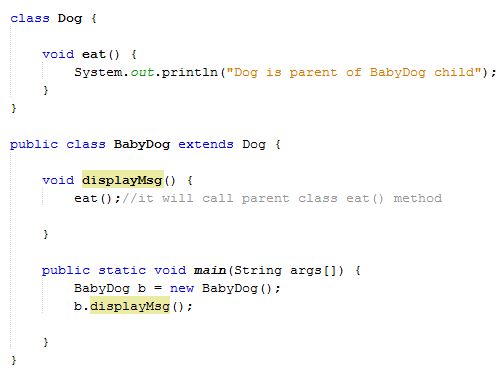
Output


In the example, shown above, eat() method is call from BabyDog class but BabyDog class does not have eat() method, Hence, we can call Dog class eat() method directly.
Summary
Thus, we learnt that “super” keyword is a reference variable, which is used to refer the current parent class object and also learnt how to use it in Java.