Introduction Of Synchronization In Java
Synchronization
In Java, Synchronization is the capacity to control the access of the multiple threads to any shared resource. It is a good option, when we want to allow only one thread to access the shared resource.
Why we use synchronization?
It is used to prevent thread interference. It is used to prevent consistency problem.
Types of Synchronization
There are two kinds of synchronization, which are,
- Process Synchronization
- Thread Synchronization
Since Java uses thread synchronization only, so we are going to discuss it only.
Thread Synchronization
There are two kinds of thread synchronization.
- Mutual Exclusive
- Synchronized method.
- Synchronized block.
- Static synchronization.
- Cooperation (Inter-thread communication)
Mutual Exclusive
Mutual Exclusive helps to keep the threads from a interfere with one another as sharing data.
This can be done by three ways, which are,
- By synchronized method
- By synchronized block
- By static synchronization
Lock Concept
Synchronization is built around an internal entity called as the lock or monitor. All objects have a lock associated with it. By rule, a thread that needs consistent access to an object's fields has to get the object's lock before accessing them and release the lock, when it's done with them. Since Java 5, the package java.util.concurrent.locks contains many lock implementations.
Let’s see an example without synchronization.
Code
- class A {
- void print(int n) {
- for (int i = 1; i <= 5; i++) {
- System.out.println(n * i);
- try {
- Thread.sleep(1000);
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
- }
- class MyThread1 extends Thread {
- A a;
- MyThread1(A a) {
- this.a = a;
- }
- public void run() {
- a.print(1);
- }
- }
- class MyThread2 extends Thread {
- A a;
- MyThread2(A a) {
- this.a = a;
- }
- public void run() {
- a.print(100);
- }
- }
- public class SynchronizationExample {
- public static void main(String args[]) {
- A obj = new A();
- MyThread1 t1 = new MyThread1(obj);
- MyThread2 t2 = new MyThread2(obj);
- t1.start();
- t2.start();
- }
- }
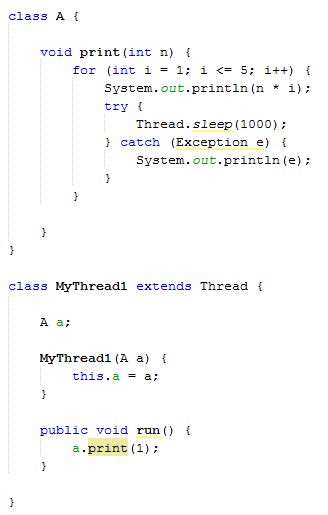
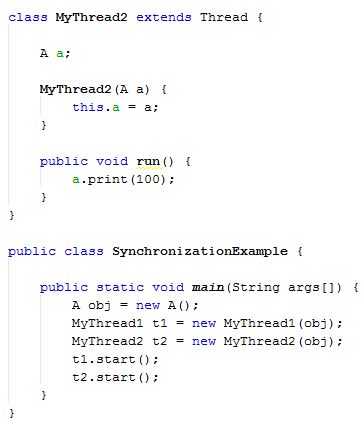
Output
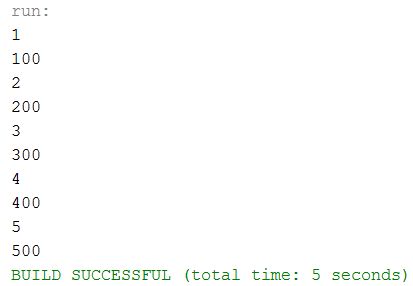
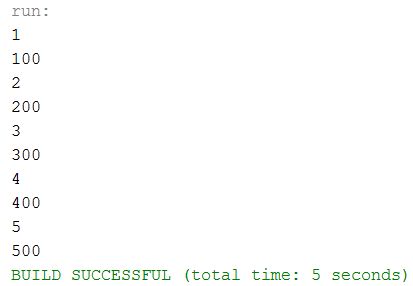
In the example, mentioned above, there is no synchronization. Thus output is inconsistent.
Synchronized method
If we create any method as synchronized, it is called as synchronized method. Synchronized method locks an object for any shared resource.
When a thread calls a synchronized method, it automatically gets the lock for that object and frees it when the thread completes its task.
Let’s see an example of synchronized method, given below.
Code
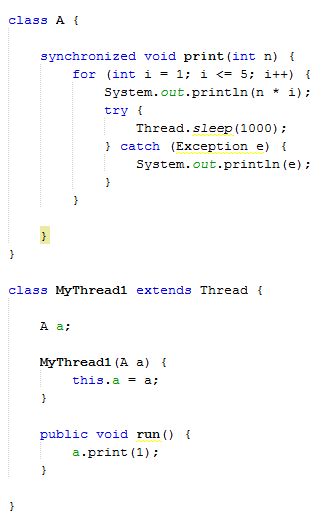
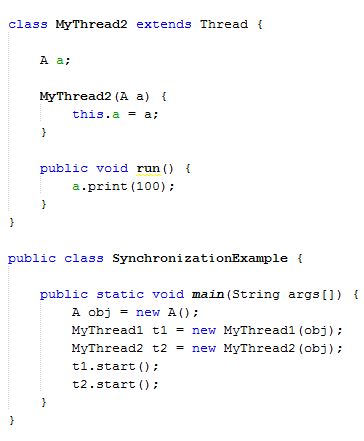
- class A {
- synchronized void print(int n) {
- for (int i = 1; i <= 5; i++) {
- System.out.println(n * i);
- try {
- Thread.sleep(1000);
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
- }
- class MyThread1 extends Thread {
- A a;
- MyThread1(A a) {
- this.a = a;
- }
- public void run() {
- a.print(1);
- }
- }
- class MyThread2 extends Thread {
- A a;
- MyThread2(A a) {
- this.a = a;
- }
- public void run() {
- a.print(100);
- }
- }
- public class SynchronizationExample {
- public static void main(String args[]) {
- A obj = new A();
- MyThread1 t1 = new MyThread1(obj);
- MyThread2 t2 = new MyThread2(obj);
- t1.start();
- t2.start();
- }
- }
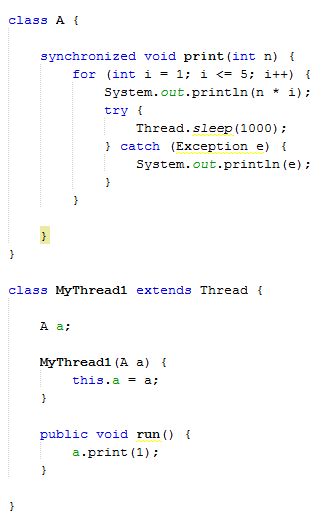
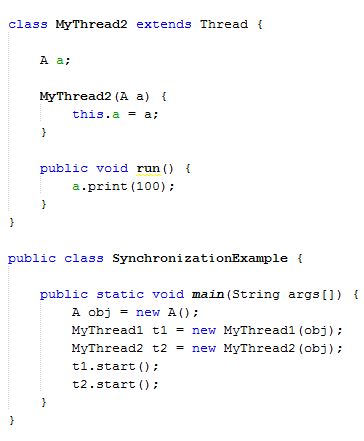
Output
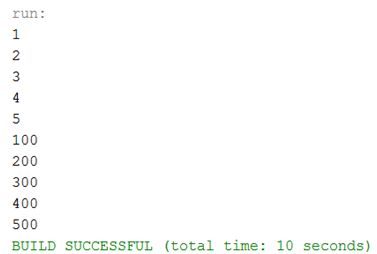
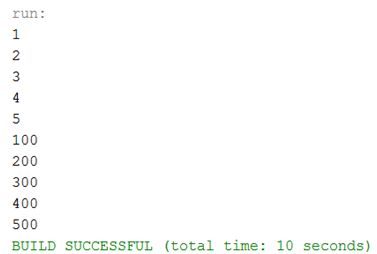
Let’s see an example of synchronized method with annonymous class.
Code
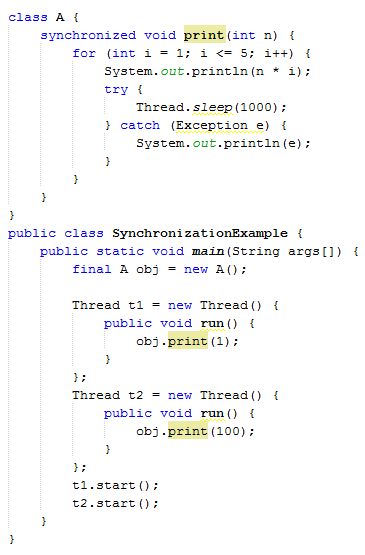
- class A {
- synchronized void print(int n) {
- for (int i = 1; i <= 5; i++) {
- System.out.println(n * i);
- try {
- Thread.sleep(1000);
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
- }
- public class SynchronizationExample {
- public static void main(String args[]) {
- final A obj = new A();
- Thread t1 = new Thread() {
- public void run() {
- obj.print(1);
- }
- };
- Thread t2 = new Thread() {
- public void run() {
- obj.print(100);
- }
- };
- t1.start();
- t2.start();
- }
- }
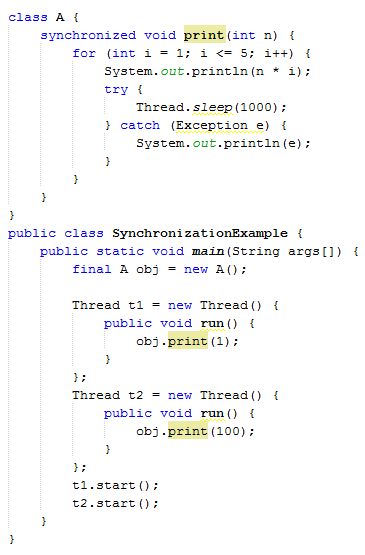
Output
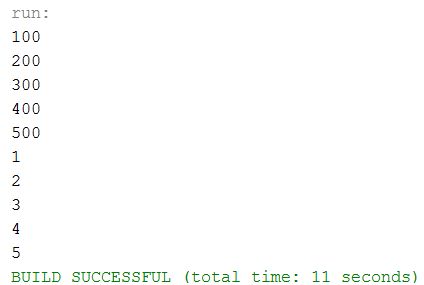
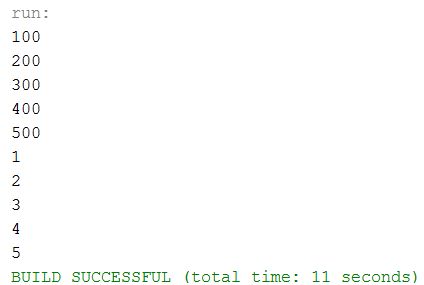
In the program mentioned above, we create two threads with annonymous class. Thus, less coding is required.
Summary
Thus, we learnt, Java synchronization is the capacity to control the access of multiple threads to any shared resource and also learnt why we need to use it in Java.