TreeSet Class In Java
TreeSet Class
In Java, TreeSet class holds only unique elements like HashSet. The TreeSet class implements NavigableSet interface, which extends the SortedSet interface. This class maintains an ascending order.
This class is similar to HashSet, except that it sorts the elements in the ascending order as HashSet doesn’t maintain any order. TreeSet allows null element. This class is also not synchronized.
Constructors of TreeSet Class
TreeSet( )
This constructor is used to construct an empty tree set, which will be sorted in an ascending order according to the natural order of its elements.
TreeSet(Collection c)
This constructor is used to build a tree set, which contains the elements of the collection c.
TreeSet(Comparator comp)
This constructor is used to construct an empty tree set, which will be sorted according to the given comparator.
TreeSet(SortedSet ss)
This constructor is used to build a TreeSet, which contains the elements of the given SortedSet.
Methods of TreeSet Class
void add(Object o)
This method is used to add the particular element to the set, if it is not already present.
boolean addAll(Collection c)
This method is used to add all the elements in the specified collection to the set.
void clear()
This method is used to remove all the elements from the set.
Object clone()
This method is used to return a shallow copy of the TreeSet instance.
Comparator comparator()
This method is used to return the comparator; used to order the sorted set or null, if the tree set uses its elements natural ordering.
boolean contains(Object o)
This method is used to return true, if the set contains the particular element.
Object first()
This method is used to return the first (lowest) element, currently in the sorted set.
SortedSet headSet(Object toElement)
This method is used to return a view of the portion of the set whose elements are strictly less than toElement.
boolean isEmpty()
This method is used to return true, if the set contains no elements.
Iterator iterator()
This method is used to return an iterator over the elements in the set.
Object last()
This method is used to return the last (highest) element, currently in the sorted set.
boolean remove(Object o)
This method is used to remove the particular element from the set, if it is present.
int size()
This method is used to return the number of elements in the set.
SortedSet subSet(Object fromElement, Object toElement)
This method is used to return a view of the portion of the set whose elements range from fromElement, inclusive and to toElement, exclusive.
SortedSet tailSet(Object fromElement)
This method is used to return a view of the portion of the set whose elements are greater than or equal to fromElement.
Let’s see an example, given below.
Code
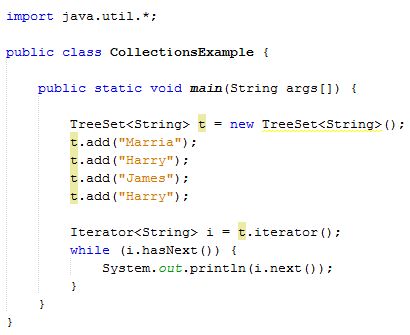
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- TreeSet < String > t = new TreeSet < String > ();
- t.add("Marria");
- t.add("Harry");
- t.add("James");
- t.add("Harry");
- Iterator < String > i = t.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- }
- }
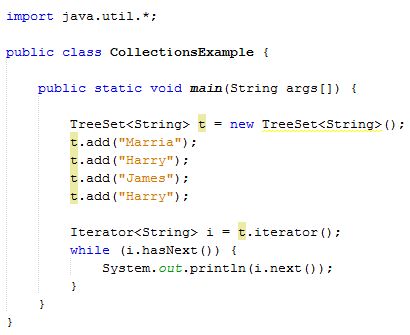
Output
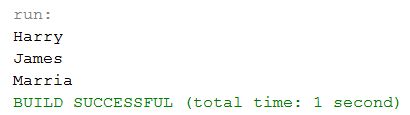
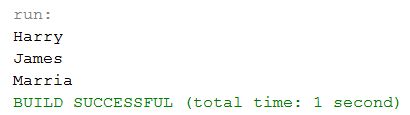
In the example, shown above, we can observe the output- TreeSet maintains an ascending order.
Summary
Thus, we learnt that Java TreeSet class holds only unique elements like HashSet. TreeSet class implements NavigableSet interface, which extends the SortedSet interface. This class maintains an ascending order and also learnt its important methods in Java.