Enum In Java
Enum
In Java, Enum is a data type, which holds the fixed set of constants. It can be used for days of the week like Sunday, Monday etc. and directions like North, South etc. The Enum constants are static and final implicitly. It is available from JDK 1.5.
Enums can be thought of as the classes, which contains a fixed set of constants.
Important points for Java Enum
- It improves the type safety.
- It can be easily used in the switch.
- It can be traversed.
- It can have the fields, constructors and methods.
- It may implement many interfaces but cannot extend any class because it internally extends Enum class.
Let’s see an example, given below.
Code
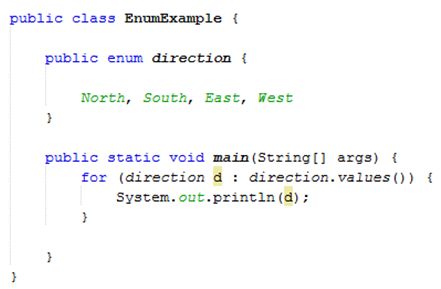
- public class EnumExample {
- public enum direction {
- North, South, East, West
- }
- public static void main(String[] args) {
- for (direction d : direction.values()) {
- System.out.println(d);
- }
- }
- }
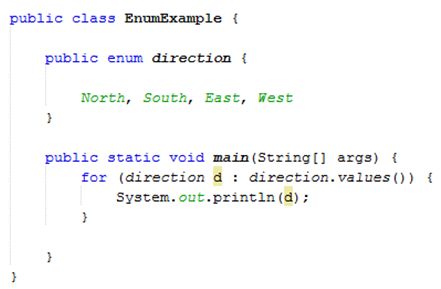
Output
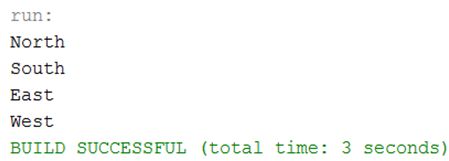
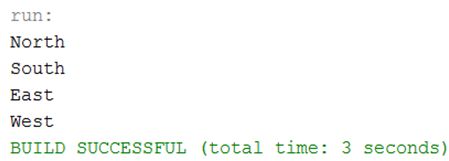
What is the purpose of values() method in Enum?
The compiler internally adds the values() method, when it creates an Enum. The values() method is used to return an array, which contains all the values of the Enum.
The compiler internally creates a static and final class that extends the Enum class.
What Enum can do in Java
Enum can define within or outside the class because it is similar to a class.
Let’s see an example- define outside class, given below.
Code
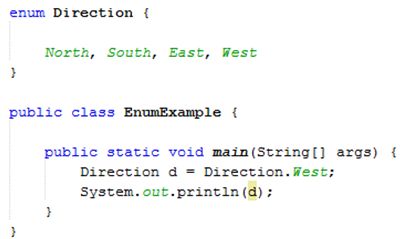
- enum Direction {
- North, South, East, West
- }
- public class EnumExample {
- public static void main(String[] args) {
- Direction d = Direction.West;
- System.out.println(d);
- }
- }
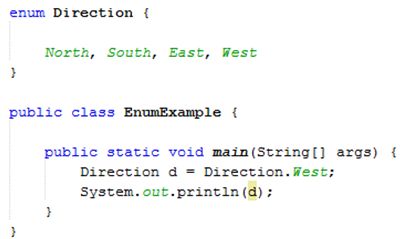
Output


Let’s see an example- define inside class, given below.
Code
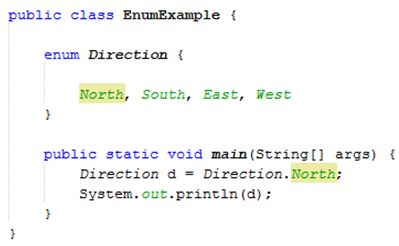
- public class EnumExample {
- enum Direction {
- North, South, East, West
- }
- public static void main(String[] args) {
- Direction d = Direction.North;
- System.out.println(d);
- }
- }
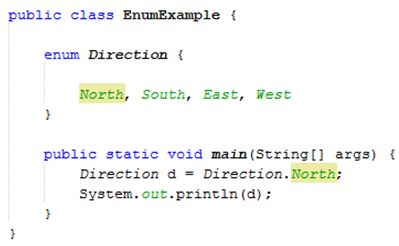
Output


Initializing exact values to the enum constants
In Java, The enum constants have initial value which starts from 0, 1, 2, and so on. But we can initialize the exact value to the enum constants with defining fields and constructors. As specified earlier, Enum can have fields, constructors and methods.
Let’s see an example of specifying the initial value to Enum constants.
Code
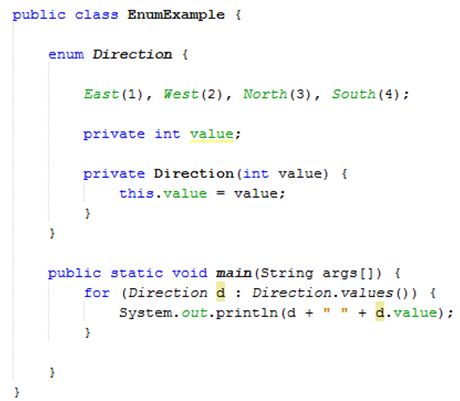
- public class EnumExample {
- enum Direction {
- East(1), West(2), North(3), South(4);
- private int value;
- private Direction(int value) {
- this.value = value;
- }
- }
- public static void main(String args[]) {
- for (Direction d : Direction.values()) {
- System.out.println(d + " " + d.value);
- }
- }
- }
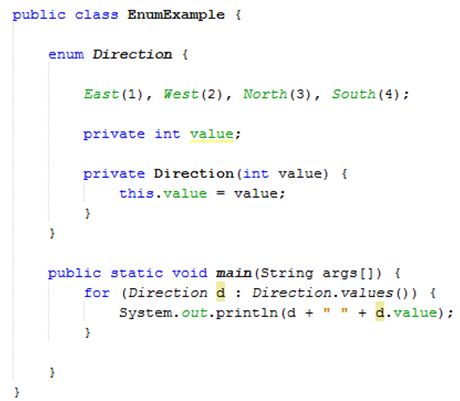
Output
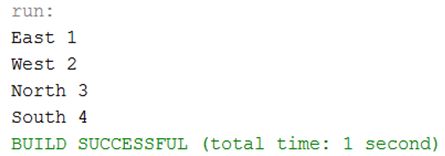
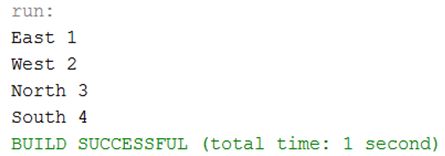
In the example, mentioned above, constructor of Enum type is private. If we don't declare the private compiler internally, it creates the private constructor. We cannot create the object of Enum by new keyword because it contains only private constructors. We can have abstract methods and can give the implementation of these methods.
Enum in switch statement
We can apply Enum on switch statement.
Let’s see an example of Enum on switch statement, given below.
Code
- public class EnumExample {
- enum Direction {
- East, West, North, South
- }
- public static void main(String args[]) {
- Direction d = Direction.South;
- switch (d) {
- case South:
- System.out.println("south");
- break;
- case North:
- System.out.println("north");
- break;
- default:
- System.out.println("other directions");
- }
- }
- }
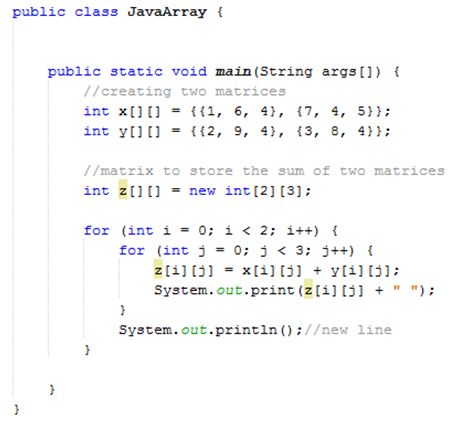
Output


Summary
Thus, we learnt that Java Enum is a data type, which holds the fixed set of the constants. It can be used for the days of the week like Sunday, Monday etc. and directions like North, South etc. and how we can use it in Java.