An Executable Jar File In Java
Executable Jar File
The jar tool of JDK gives the facility to make the executable jar file. An executable jar file calls the main method of the class, if we double click it.
To create the executable jar file, we need to create .mf file. It’s also called as manifest file.
To create the executable jar file, we need to create .mf file. It’s also called as manifest file.
Manifest file
To create manifest file, we need to write Main-Class, colon, space and the class name followed by enter.
For example
myfile.mf
Main-Class: Simple
We can see, the mf file begins with Main-Class colon space class name. Now, class name is simple and the new line is must after the class name is in mf file.
Creating executable jar file using jar tool
The jar tool gives many switches, such as.
- -c it makes new archive file.
- -v it produces verbose output, displays the included and extracted resource on the standard output.
- -m it includes manifest information from the given mf file.
- -f it specifies the archive file name.
- -x it removes the files from the archive file.
Now, let's write the program to produce the executable jar, using mf file.
You need to write jar, swiches, mf_file, jar_file, .classfile like,
jar -cvmf myfile.mf myjar.jar Simple.class
Now, it will create the executable jar file and if we double click on jar file, it will call the main method of the Simple class.
Now, suppose we have created any Window based Application, using AWT or SWING. Let’s see,
Code
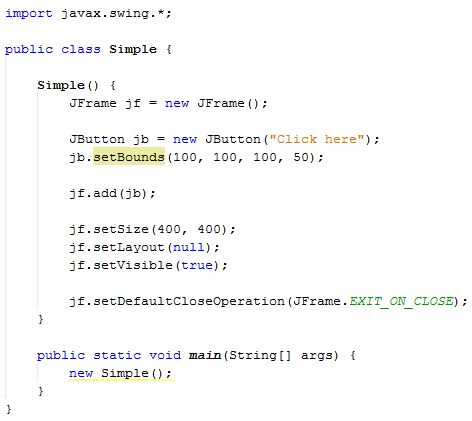
- import javax.swing.*;
- public class Simple {
- Simple() {
- JFrame jf = new JFrame();
- JButton jb = new JButton("Click here");
- jb.setBounds(100, 100, 100, 50);
- jf.add(jb);
- jf.setSize(400, 400);
- jf.setLayout(null);
- jf.setVisible(true);
- jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- }
- public static void main(String[] args) {
- new Simple();
- }
- }
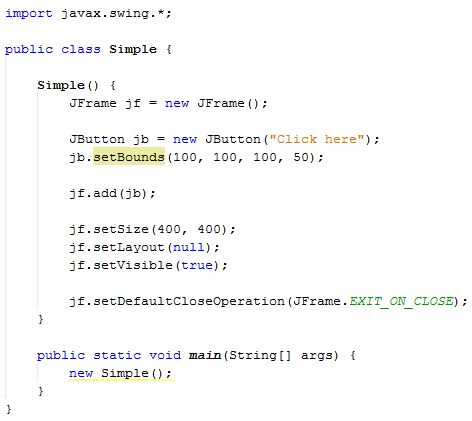
Summary
Thus, we learnt that the jar tool of JDK gives the facility to make the executable jar file and also learnt how to make an executable jar file in Java.