Access Modifiers In Java
Access Modifiers
In Java, the access modifiers give accessibility (scope) of a data member, method, constructor or class. In other words, access modifiers helps us to set the level of access for our class, variables and methods. In Java, two types of modifiers are access modifiers and non-access modifiers.
Four types of access modifiers are,
- Public
- Private
- Protected
- Default
Non-access modifiers are static, abstract, synchronized, native, volatile, transient etc.
Public access modifier
The public access modifier is accessible in all the places. It has the widest scope among all other modifiers.
Variables, methods and constructors declared public within a public class are visible to any class in the Java program. Hence, it does not matter whether these classes are in the same package or in another package.
Let’s see an example, given below.
Code
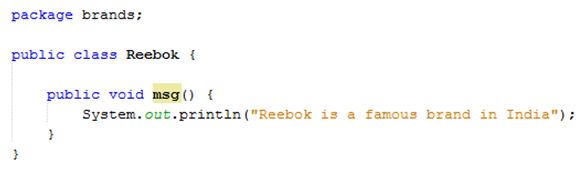
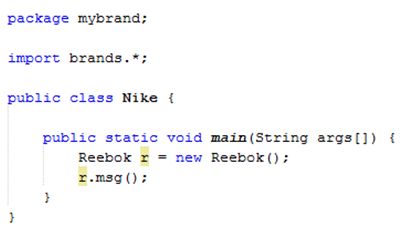
- package brands;
- public class Reebok {
- public void msg() {
- System.out.println("Reebok is a famous brand in India");
- }
- }
- package mybrand;
- import brands.*;
- public class Nike {
- public static void main(String args[]) {
- Reebok r = new Reebok();
- r.msg();
- }
- }
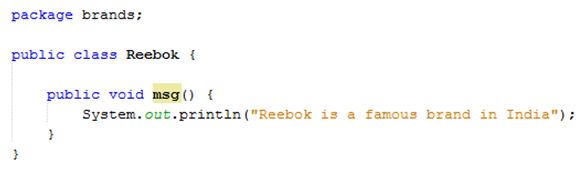
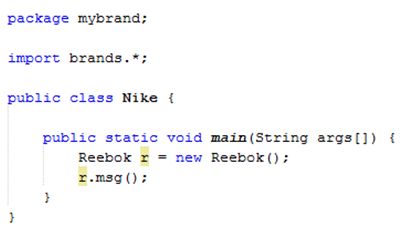
Output


Private access modifier
The private access modifier is accessible within the class only and not outside the class. The private data members or methods cannot be used for the classes and interfaces. Data members, methods or constructors declared private are strictly controlled. It means private access modifier cannot be accessed by anyplace outside the class.
A class cannot be private or protected except the nested class.
Let’s see an example, given below.
Code
- class Scooter {
- private int speed = 30;
- private Scooter() {}
- private void run() {
- System.out.println("Scooter is running");
- }
- }
- public class Car {
- public static void main(String args[]) {
- Scooter s = new Scooter();
- System.out.println(s.speed);
- s.run();
- }
- }
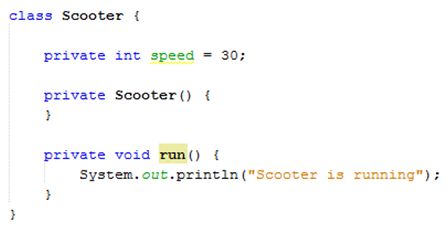
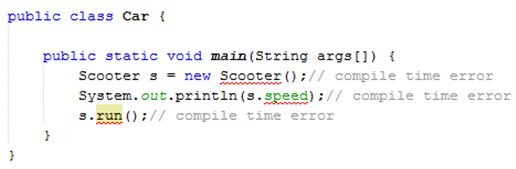
In this example, we create two classes Scooter and car. Scooter class contains private variable, constructor and method. If we want to access these private members from outside the class, it gives the compile time error.
Protected access modifier
The protected access modifier is accessible within the package and outside the package also but through inheritance only.
The protected access modifier can apply on the variables, methods and constructors but it can’t apply on the classes. Variables, methods and constructors declared are protected in a parent class can be accessed only by child classes in other packages. Classes in the same package can also access protected variables, methods and constructors as well, even if they are not a child class of the protected member’s class.
Let’s see an example, given below.
Code
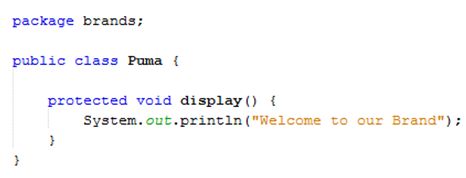
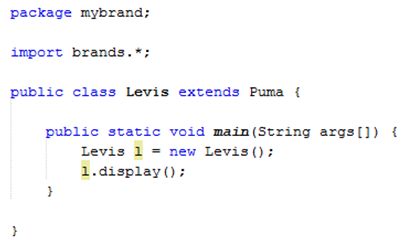
- package brands;
- public class Puma {
- protected void display() {
- System.out.println("Welcome to our Brand");
- }
- }
- package mybrand;
- import brands.*;
- public class Levis extends Puma {
- public static void main(String args[]) {
- Levis l = new Levis();
- l.display();
- }
- }
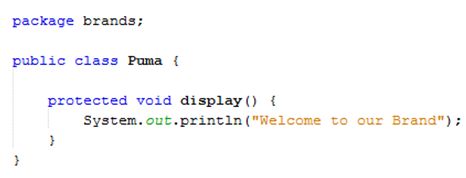
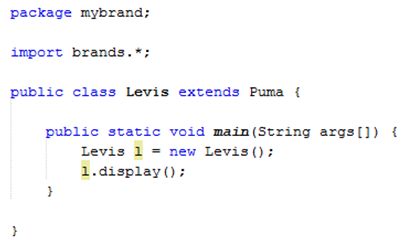
Output


In the example, mentioned above, we create the two packages brands and mybrand. The puma class of brands package is public. Hence, it can be accessed from outside the package but display method of this package is declared as protected. Hence, it can be accessed from outside the class but through an inheritance only.
Default access modifier
When we don't use any modifier, it is treated as a default access modifier in Java. The default modifier is accessible only within the package.
In other words, Java provides a default modifier, which is used when no access modifier is present. Any class, variable, method or constructor that has default access modifier is accessible only by the classes in the same package. The default access modifier is not used for the variables and methods within an interface.
Let’s see an example, given below.
Code
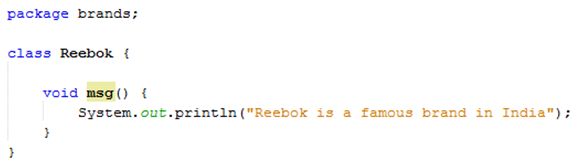
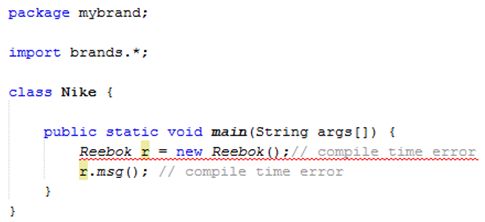
- package brands;
- class Reebok {
- void msg() {
- System.out.println("Reebok is a famous brand in India");
- }
- }
- package mybrand;
- import brands.*;
- class Nike {
- public static void main(String args[]) {
- Reebok r = new Reebok(); // compile time error
- r.msg(); // compile time error
- }
- }
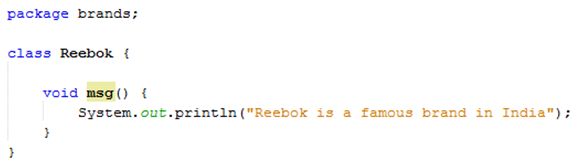
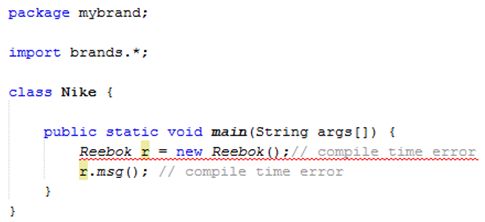
In the example, shown above, we create two packages brands and mybrand. We are accessing the reebok class from outside its package and reebok class is not public. Hence, it cannot be accessed from outside the package.
Let’s understand with the table, given below.
Access Modifiers | Within class | Within package | Outside package by subclass only | Outside package |
Private | Yes | No | No | No |
Default | Yes | Yes | No | No |
Protected | Yes | Yes | Yes | No |
Public | Yes | Yes | Yes | Yes |
The overridden method must not be more restrictive.
Summary
Thus, we learnt that access modifiers helps us to set the level of access for our class, variables, methods and also learnt their types.