Member Inner Class In Java
Member inner class
Member inner class is created inside the class but outside the method, it is a non-static class in Java.
Creating a member inner class is simple. We need to write a class within a class. The inner class can be private and once we declare an inner class as a private, it cannot be accessed from an object outside the class.
Syntax
class Outer{
class Inner{
}
}
Let’s see an example, given below.
Code
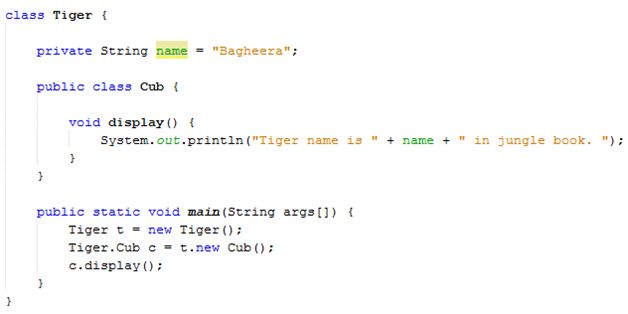
- class Tiger {
- private String name = "Bagheera";
- public class Cub {
- void display() {
- System.out.println("Tiger name is " + name + " in jungle book. ");
- }
- }
- public static void main(String args[]) {
- Tiger t = new Tiger();
- Tiger.Cub c = t.new Cub();
- c.display();
- }
- }
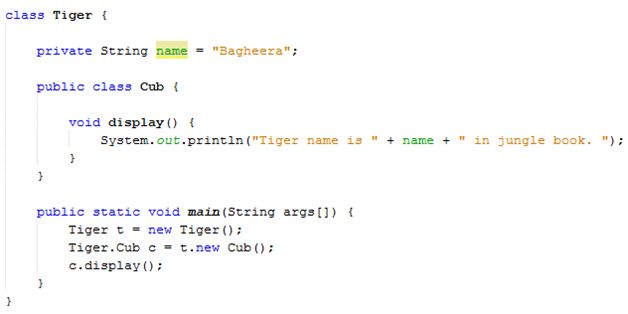
Output


In the example, mentioned above, we create display () method in member inner class, which accesses the private data member of outer class.
Internal working of member inner class
In case of inner class, internally the compiler creates two class files and the file name of inner class is "Outer$Inner". If we want to instantiate the inner class, we should have to create the instance of outer class. In this case, instance of inner class is created inside the instance of outer class.
Summary
Thus, we learnt that a Member inner class is created inside the class but outside the method in Java and also learnt how it works internally.