StringTokenizer In Java
StringTokenizer
In Java, the StringTokenizer class is used to break a string into small tokens. It is a simple way to break the string.
StringTokenizer class is a legacy class, which is retained for the compatibility reasons, while its use is discouraged in the new code. Its methods do not differentiate between the identifiers, numbers, quoted strings and also do not recognize and skip the comments.
Declaration of StringTokenizer class
The declaration for java.util.StringTokenizer class is given below.
public class StringTokenizer
extends Object
implements Enumeration<Object>
Constructors of StringTokenizer class
Three constructors defined in the StringTokenizer class are.
StringTokenizer(String str)
This constructor creates StringTokenizer with the particular string.
StringTokenizer(String str, String delim)
This constructor creates StringTokenizer with the particular string and delimeter.
StringTokenizer(String str, String delim, boolean returnValue)
This constructor creates StringTokenizer with the particular string, delimeter and returnValue. If return value is true, delimiter characters are considered to be tokens and if it is false, delimiter characters serve to the separate tokens.
Methods of StringTokenizer class
Six methods are very useful of StringTokenizer class are as follows.
boolean hasMoreTokens()
This method checks, if there are more tokens available.
String nextToken()
This method returns the next token from StringTokenizer object.
String nextToken(String delim)
This method returns next token, based on the delimeter.
boolean hasMoreElements()
This method is same as hasMoreTokens() method.
Object nextElement()
This method is same as nextToken(), but its return type is an object.
int countTokens()
This method returns the total number of tokens.
Now, let’s see an example of StringTokenizer class, given below.
Code
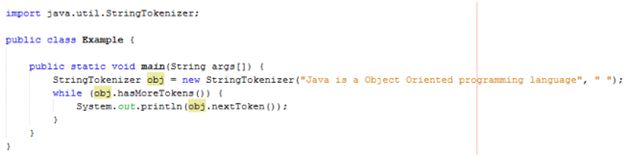
- import java.util.StringTokenizer;
- public class Example {
- public static void main(String args[]) {
- StringTokenizer obj = new StringTokenizer("Java is a Object Oriented programming language", " ");
- while (obj.hasMoreTokens()) {
- System.out.println(obj.nextToken());
- }
- }
- }
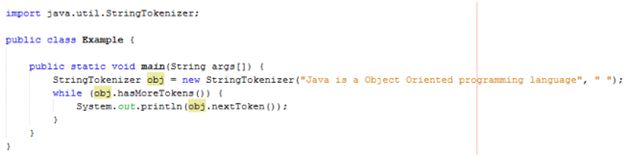
Output
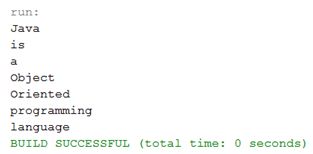
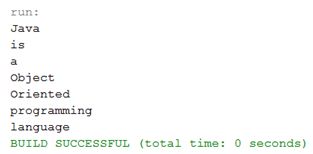
In the example, shown above, we can see that StringTokenizer class tokenizes a string " Java is a Object Oriented programming language " on the basis of whitespace.
Now, let’s see an example of one of the method nextToken(String delim) of StringTokenizer class.
Code
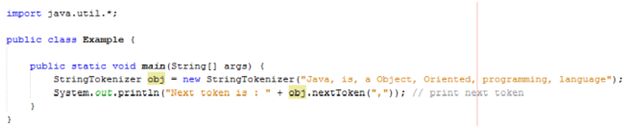
- import java.util.*;
- public class Example {
- public static void main(String[] args) {
- StringTokenizer obj = new StringTokenizer("Java, is, a Object, Oriented, programming, language");
- System.out.println("Next token is : " + obj.nextToken(",")); // print next token
- }
- }
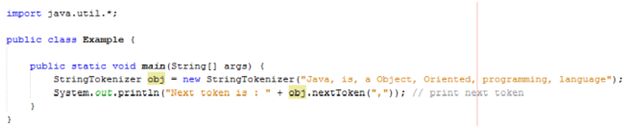
Output


Summary
Thus, we learnt that StringTokenizer class is used to break a string into the tokens in Java and also learnt its important methods.