PipedInputStream And PipedOutputStream Classes in Java
PipedInputStream and PipedOutputStream classes
PipedInputStream and PipedOutputStream classes have a capability to read and write the data at the same time.
Both streams are connected with each other with the connect() method of the PipedOutputStream class.
Let’s see an example with threads, given below.
Code
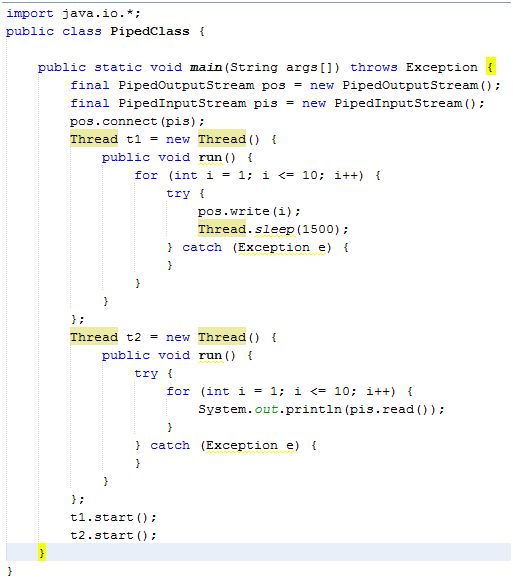
- public class PipedClass {
- public static void main(String args[]) throws Exception {
- final PipedOutputStream pos = new PipedOutputStream();
- final PipedInputStream pis = new PipedInputStream();
- pos.connect(pis);
- Thread t1 = new Thread() {
- public void run() {
- for (int i = 1; i <= 10; i++) {
- try {
- pos.write(i);
- Thread.sleep(1500);
- } catch (Exception e) {}
- }
- }
- };
- Thread t2 = new Thread() {
- public void run() {
- try {
- for (int i = 1; i <= 10; i++) {
- System.out.println(pis.read());
- }
- } catch (Exception e) {}
- }
- };
- t1.start();
- t2.start();
- }
- }
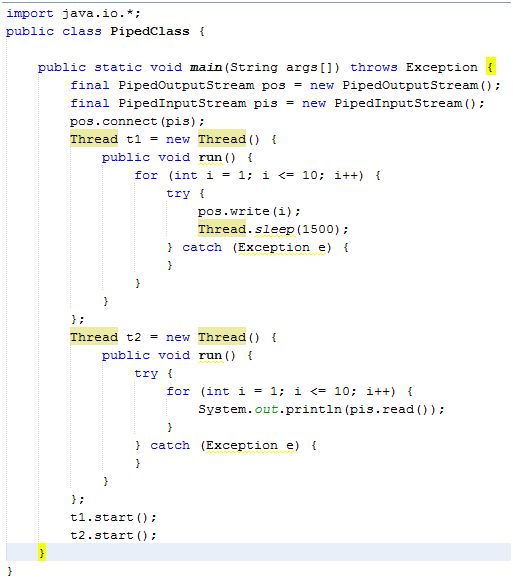
Output
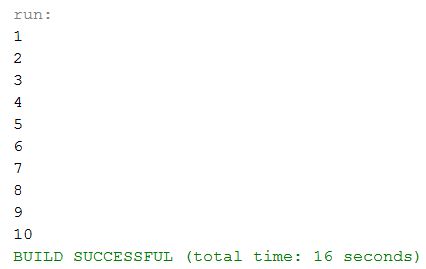
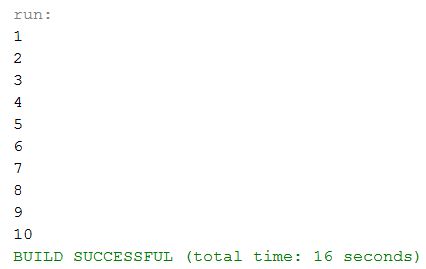
In the example, mentioned above, we create two threads t1 and t2. The thread (t1) writes the data. Use the PipedOutputStream object and the thread (t2) reads the data from that pipe. Both the piped stream objects are connected with each other.
Summary
Thus, we learnt that PipedInputStream and PipedOutputStream classes have a capability to read and write the data together in Java and also learnt how we can create it.