Inheritance In Java
Inheritance
In Java, Inheritance is a process, where one class object acquires all the properties and behaviors of parent class object. It represents IS-A relationship, also called as a parent-child relationship. With the use of an inheritance, we can manage the information easily.
In an inheritance, we can create new classes, which are built upon the existing classes. Main benefit of inheritance is we can reuse the methods and variables of parent class, add new methods and variables also. The class, which acquires the properties of parent class is called as a subclass (derived class, child class) and the class, whose properties are acquired are called as super class (base class, parent class).
Advantages of inheritance are given below.
- Code reusability.
- Method overriding.
Syntax of Inheritance
class Subclass-name extends Superclass-name
{
//variables and methods;
}
In Java, “extends” keyword is used to inherit the properties of a class.
Let’s see an example, given below.
Code
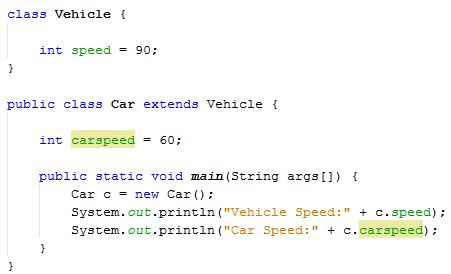
- class Vehicle {
- int speed = 90;
- }
- public class Car extends Vehicle {
- int carspeed = 60;
- public static void main(String args[]) {
- Car c = new Car();
- System.out.println("Vehicle Speed:" + c.speed);
- System.out.println("Car Speed:" + c.carspeed);
- }
- }
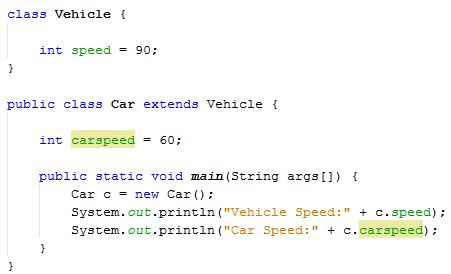
Output
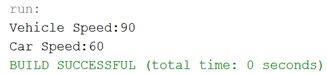
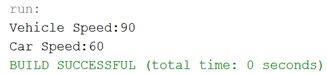
In the example, shown above, car object can access the field of own class as well as of vehicle class and that example shows the reusability.
In Java, there are various types of inheritance, which are,
Single Inheritance
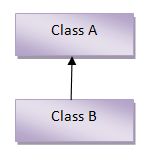
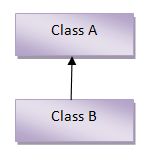
Multilevel Inheritance
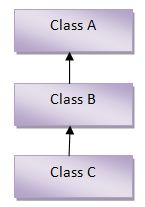
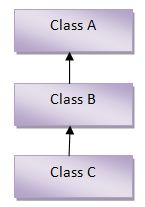
Hierarchical Inheritance
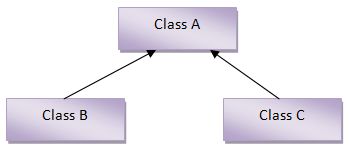
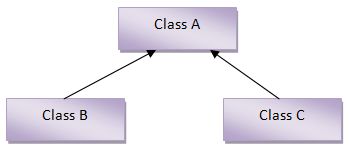
Multiple Inheritance
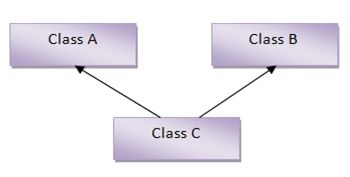
Hybrid Inheritance
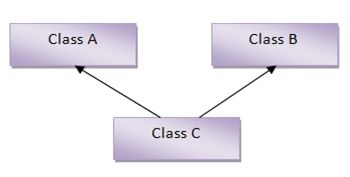
Hybrid Inheritance
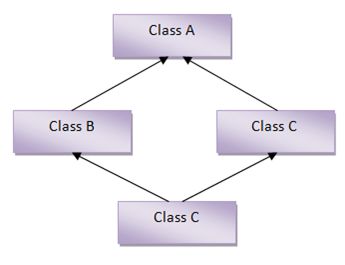
On the basis of class, three types of inheritance in Java exists, which are single, multilevel and hierarchical.
In Java, multiple and hybrid inheritance are not supported through class. Both are supported through interface only.
Why is Multiple inheritance not supported in Java?
Java is very powerful and a simple language. Multiple inheritance increases the complexity of a program. To reduce the difficulty and make language simple, Java does not support the multiple inheritance.
Suppose, we have three classes X, Y and Z. Z is a child class and inherits the properties of class X and class Y. X and Y both are parent classes. If X and Y classes have same method and when we want to call that method from child class object, it gives an error since there will be an ambiguity (confusion) to call method X or Y class. Due to this, Java does not support multiple inheritance through classes.
Summary
Thus, we learnt that inheritance is a process, where one class object acquires all the properties and the behavior of parent class object. We also learnt its types and advantages.