Queue Interface In Java
Queue Interface
In Java, the queue interface basically orders the element in FIFO (First In First Out) manner.
Methods of Queue Interface
- public boolean add(object);
- public boolean offer(object);
- public remove();
- public poll();
- public element();
- public peek();
PriorityQueue class
In Java, PriorityQueue class provides all the facility of using queue. It does not order the elements in FIFO manner.
PriorityQueue class is an unbounded priority queue, based on a priority heap. The priority queue elements are ordered, according to their usual ordering or by a comparator, provided at a queue construction time, depending on which constructor are used. It does not allow null elements and relying on natural ordering also does not allow insertion of non-comparable objects.
Constructor of PriorityQueue Class
E is the type of elements, held in the collection.
PriorityQueue()
This constructor creates a PriorityQueue with the default initial capacity, which orders its elements , according to their natural ordering.
PriorityQueue(Collection<? extends E> c)
This constructor creates a PriorityQueue, which contains the elements in the specified collection.
PriorityQueue(int initialCapacity)
This constructor creates a PriorityQueue with the specified initial capacity, which orders its elements , according to their natural ordering.
PriorityQueue(int initialCapacity, Comparator<? super E> comparator)
This constructor creates a PriorityQueue with the specified initial capacity, which orders its elements , according to the specified comparator.
PriorityQueue(PriorityQueue<? extends E> c)
This constructor creates a PriorityQueue, which contains the elements in the specified priority queue.
PriorityQueue(SortedSet<? extends E> c)
This constructor creates a PriorityQueue, which contains the elements in the specified sorted set.
Methods of PriorityQueue Class
boolean add(E e)
This method is used to insert the specified element into the priority queue.
void clear()
This method is used to remove all of the elements from the priority queue.
Comparator<? super E> comparator()
This method is used to return the comparator used to order the elements in the queue or null, if the queue is sorted, according to the natural ordering of its elements.
boolean contains(Object o)
This method is used to return true, if this queue contains the specified element.
Iterator<E> iterator()
This method is used to return an iterator over the elements in the queue.
boolean offer(E e)
This method is used to insert the specified element into the priority queue.
E peek()
This method is used to retrieve but does not remove the head of this queue or returns null, if the queue is empty.
E poll()
This method is used to retrieve and remove the head of the queue or returns null, if the queue is empty.
boolean remove(Object o)
This method is used to remove a single instance of the specified element from the queue, if it is present.
int size()
This method is used to return the number of elements in the collection.
Object[] toArray()
This method is used to return an array, which contains all the elements in the queue.
<T> T[] toArray(T[] a)
This method is used to return an array, which contains all the elements in the queue; the runtime type of the returned array is that of the specified array.
Let’s see an example, given below.
Code
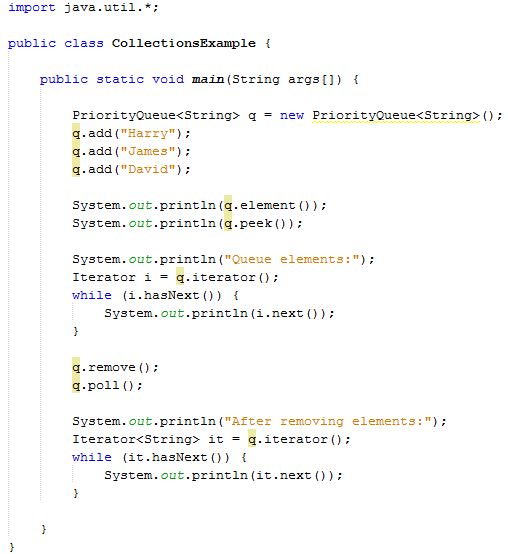
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- PriorityQueue < String > q = new PriorityQueue < String > ();
- q.add("Harry");
- q.add("James");
- q.add("David");
- System.out.println(q.element());
- System.out.println(q.peek());
- System.out.println("Queue elements:");
- Iterator i = q.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- q.remove();
- q.poll();
- System.out.println("After removing elements:");
- Iterator < String > it = q.iterator();
- while (it.hasNext()) {
- System.out.println(it.next());
- }
- }
- }
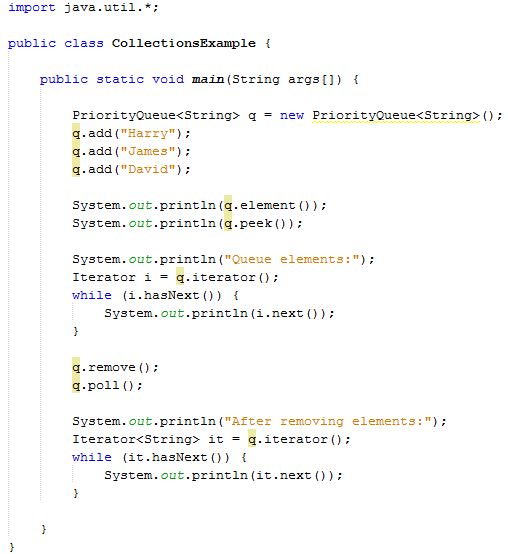
Output
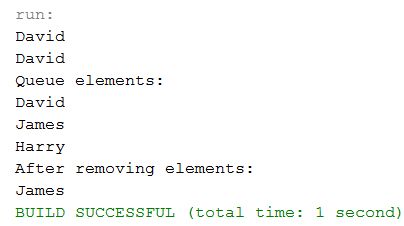
Summary
Thus, we learnt that Java queue interface basically orders the element in FIFO (First In First Out) manner and we also learnt how we can use PriorityQueue class in Java.