JTable Class In Java
JTable Class
In Java, JTable class displays the data on two dimensional tables of cells.
Constructors of JTable class
JTable()
This constructor creates a table with the empty cells.
JTable(Object[][] rows, Object[] columns)
This constructor creates a table with the specific data.
Let’s see an example of JTable class, given below.
Code
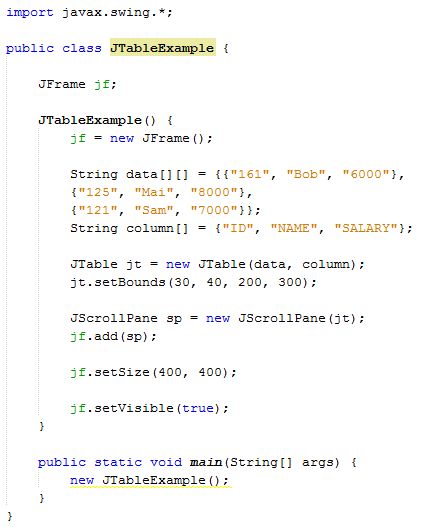
- import javax.swing.*;
- public class JTableExample {
- JFrame jf;
- JTableExample() {
- jf = new JFrame();
- String data[][] = {{"161", "Bob", "6000"},
- {"125", "Mai", "8000"},
- {"121", "Sam", "7000"}};
- String column[] = {"ID", "NAME", "SALARY"};
- JTable jt = new JTable(data, column);
- jt.setBounds(30, 40, 200, 300);
- JScrollPane sp = new JScrollPane(jt);
- jf.add(sp);
- jf.setSize(400, 400);
- jf.setVisible(true);
- }
- public static void main(String[] args) {
- new JTableExample();
- }
- }
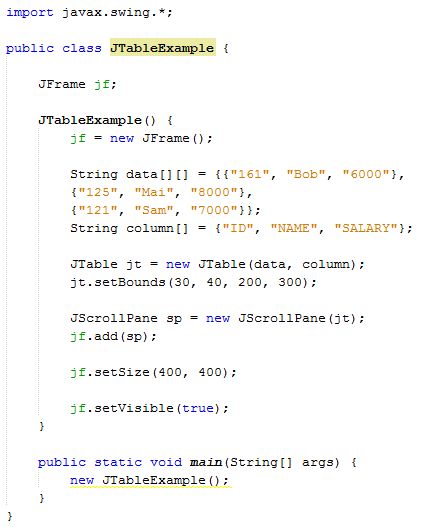
Output
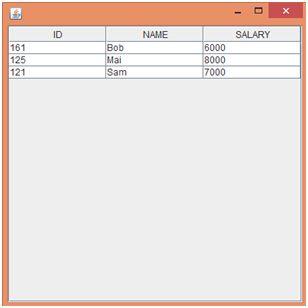
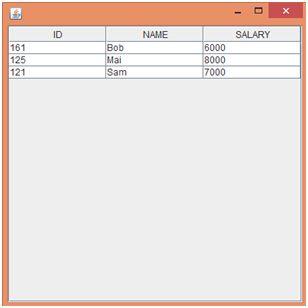
Summary
Thus, we learnt that the JTable class displays the data on the two dimensional tables of the cells and also learnt how to use it in Java.