Nashorn JavaSript In Java
Nashorn JavaSript
Nashorn an improved javascript engine is introduced in Java 8 to change the existing Rhino. It gives better performance, because it directly compiles the code in memory and passes the bytecode to JVM. It uses invokedynamics feature which introduced in Java 7 to improve performance.
jjs
Java 8 introduces a new command line tool for Nashorn engine jjs, to perform JavaScript codes at console.
Interpreting js File
Create and save the file first.js in c:\> JAVA folder.
first.js
display('Java 8');
Then, open console and use the command.
$jjs first.js
Output
Java 8
jjs in Interactive Mode
Open the console and use the command.
$jjs
jjs> display("Java 8")
Java 8
jjs> quit()
>>
Pass Arguments
Open the console and use the command.
$jjs – x y z
jjs> display('letters: ' +arguments.join(", "))
letters: x, y, z
jjs>
Call JavaScript from Java
With the usage of ScriptEngineManager, JavaScript code can be called and interpreted in Java.
Let’s see an example of Nashorn JavaScript.
Code
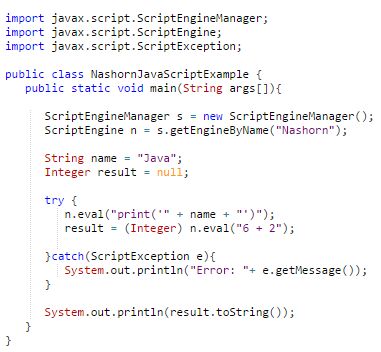
- import javax.script.ScriptEngineManager;
- import javax.script.ScriptEngine;
- import javax.script.ScriptException;
- public class NashornJavaScriptExample {
- public static void main(String args[]) {
- ScriptEngineManager s = new ScriptEngineManager();
- ScriptEngine n = s.getEngineByName("Nashorn");
- String name = "Java";
- Integer result = null;
- try {
- n.eval("print('" + name + "')");
- result = (Integer) n.eval("6 + 2");
- } catch (ScriptException e) {
- System.out.println("Error: " + e.getMessage());
- }
- System.out.println(result.toString());
- }
- }
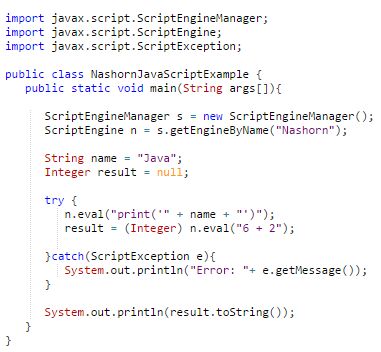
Output


Summary
Thus, we learned that Nashorn an improved javascript engine is introduced in Java 8 to change the existing Rhino. It gives better performance and also learns how to use it in Java.