Custom Exception In Java
Custom Exception
If we create our own exception, it is called as a custom or user-defined exception in Java.
In other words, sometimes we want to customize the exception, according to our need or required to develop the meaningful exceptions, based on the Application requirements, we can create our own exceptions by extending Exception class.
Let's see an example, given below.
Code
- package exceptionHandling;
- public class MyownException extends Exception {
- public MyownException(String message) {
- super(message);
- }
- }
- package exceptionHandling;
- public class CustomException {
- public static void main(String args[]) throws Exception {
- CustomException obj = new CustomException();
- obj.displayNums();
- }
- public void displayNums() throws MyownException {
- for (int i = 0; i < 15; i++) {
- System.out.println("Number = " + i);
- if (i == 10) {
- throw new MyownException("My own Exception Occurred");
- }
- }
- }
- }
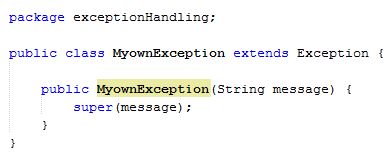
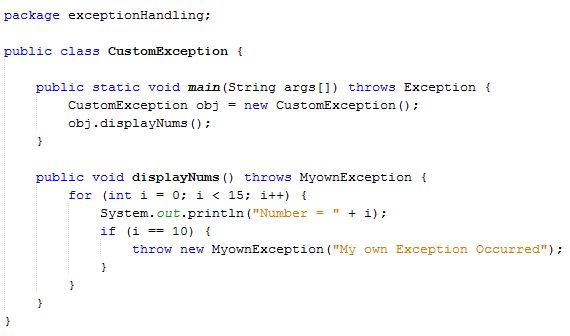
Output
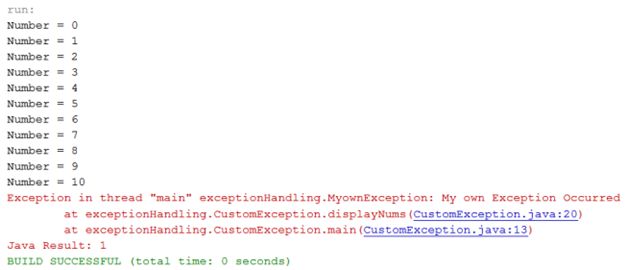
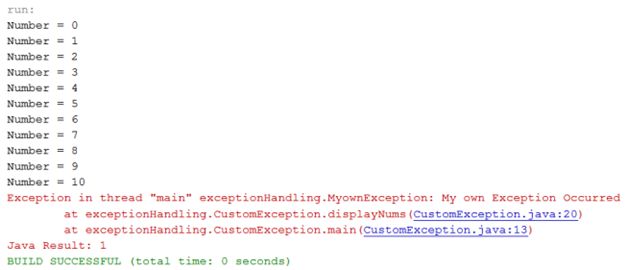
In the example, shown above, first we create a class MyownException . This class extends Exception class, declares a constructor and call parent class constructor.
We create another class CustomException, one method displaynums() and throws MyownException with the method body. This method displayNums may throw "MyownException". The loop in the displayNums method display numbers. If "i" equals to 10, throw "MyownException" with a message "My own Exception Occurred".
Summary
Thus, we learnt that sometimes we want to customize the exception, according to our need due to which we use custom exception in Java and also learnt how to create custom exception.