Difference Between String And StringBuffer In Java
Differentiate Between String And StringBuffer
String is an object which represents sequence of char values. Java platform provides the String class to create and manipulate strings.
In Java, We know that string is immutable. It cannot be changed but a new instance is created. That’s why we use StringBuffer and StringBuilder class for mutable class.
StringBuffer class is used to create for mutable string that means we can modify class through StringBuffer class in Java.
The StringBuffer class is similar as String class except it is mutable. It can be changed easily.
Let’s see the differences between String and StringBuffer are given below.
String | StringBuffer |
String is immutable (if once we created, cannot be modified) object. | StringBuffer is mutable (it can be modified) object. |
String performance is very slow and consumes more memory at the time of concatenate strings because every time it creates new instance. | StringBuffer performance is faster than string and it consumes less memory at the time of concatenate strings. |
String class overrides the equals() method of object class. With the use of it we can compare the contents of two strings. | StringBuffer class doesn’t overrides the equals() method of object class. |
The object created as a string is takes memory or stored in the constant String Pool. | The object created through StringBuffer is stored in the heap memory. |
For example:
String str = “Marria”;
str = “Harry”;
|
For example:
StringBuffer str = new StringBuffer(“Peter”);
str.append(“Jones”);
|
Let’s check the performance of String and StringBuffer.
Code
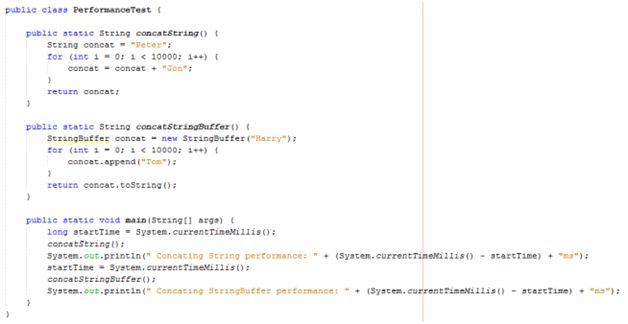
- public class PerformanceTest {
- public static String concatString() {
- String concat = "Peter";
- for (int i = 0; i < 10000; i++) {
- concat = concat + "Jon";
- }
- return concat;
- }
- public static String concatStringBuffer() {
- StringBuffer concat = new StringBuffer("Harry");
- for (int i = 0; i < 10000; i++) {
- concat.append("Tom");
- }
- return concat.toString();
- }
- public static void main(String[] args) {
- long startTime = System.currentTimeMillis();
- concatString();
- System.out.println(" Concating String performance: " + (System.currentTimeMillis() - startTime) + "ms");
- startTime = System.currentTimeMillis();
- concatStringBuffer();
- System.out.println(" Concating StringBuffer performance: " + (System.currentTimeMillis() - startTime) + "ms");
- }
- }
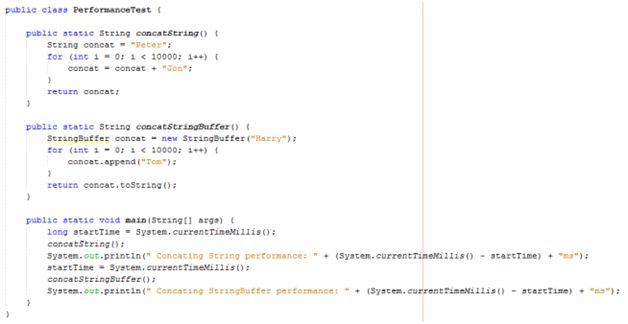
Output
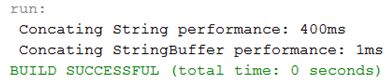
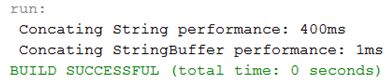
Now, let’s check the String and StringBuffer HashCode Test.
Code
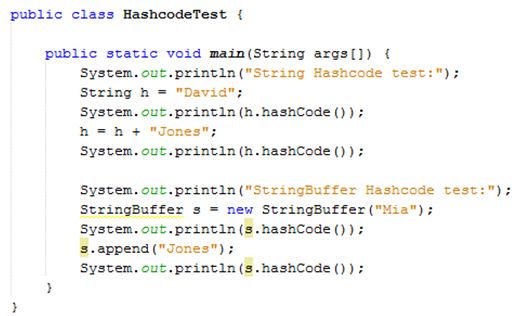
- public class HashcodeTest {
- public static void main(String args[]) {
- System.out.println("String Hashcode test:");
- String h = "David";
- System.out.println(h.hashCode());
- h = h + "Jones";
- System.out.println(h.hashCode());
- System.out.println("StringBuffer Hashcode test:");
- StringBuffer s = new StringBuffer("Mia");
- System.out.println(s.hashCode());
- s.append("Jones");
- System.out.println(s.hashCode());
- }
- }
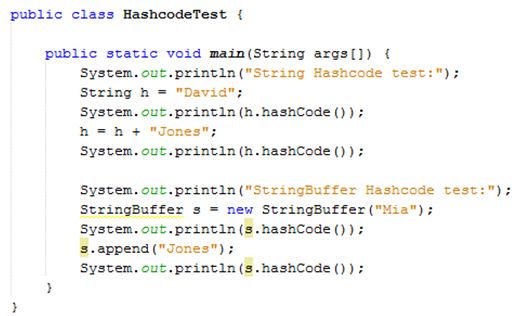
Output
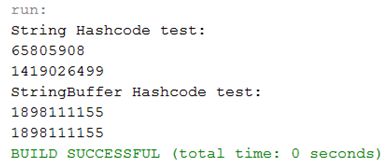
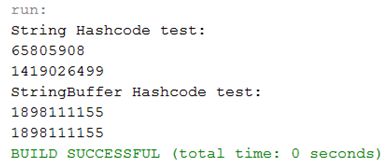
In the program above example, we can see that string returns new hashcode value when we concat string but StringBuffer returns same hashcode value when we concat stringBuffer.
Summary
Thus, we learned that String is immutable. It cannot be changed but a new instance is created and StringBuffer class is same as String class except it is mutable. It can be changed easily and also learn their differences between in Java.