How To Format Date In Java
Format Date
In Java, there are two classes for formatting the date, which are,
- DateFormat.
- SimpleDateFormat.
DateFormat
In Java, java.text.DateFormat class provides many methods to format and parse the date and time in the language in an independent manner. The DateFormat is an abstract class, java.text.Format is the parent class and java.text.SimpleDateFormat is the child class of java.text.DateFormat class.
We can convert the date into the string, which is called as formatting and vice-versa parsing.
In other words, parsing means the string to the date and formatting means the date to the string in Java.
Fields of java.text.DateFormat
- protected Calendar calendar
- protected NumberFormat numberFormat
- public static final int ERA_FIELD
- public static final int YEAR_FIELD
- public static final int MONTH_FIELD
- public static final int DATE_FIELD
- public static final int HOUR_OF_DAY1_FIELD
- public static final int HOUR_OF_DAY0_FIELD
- public static final int MINUTE_FIELD
- public static final int SECOND_FIELD
- public static final int MILLISECOND_FIELD
- public static final int DAY_OF_WEEK_FIELD
- public static final int DAY_OF_YEAR_FIELD
- public static final int DAY_OF_WEEK_IN_MONTH_FIELD
- public static final int WEEK_OF_YEAR_FIELD
- public static final int WEEK_OF_MONTH_FIELD
- public static final int AM_PM_FIELD
- public static final int HOUR1_FIELD
- public static final int HOUR0_FIELD
- public static final int TIMEZONE_FIELD
- public static final int FULL
- public static final int LONG
- public static final int MEDIUM
- public static final int SHORT
- public static final int DEFAULT
Methods of java.text.DateFormat
Public Method
final String format(Date date)
This method is used to convert the given date object into the string.
Date parse(String source)throws ParseException
This method is used to convert the string into the date object.
static final DateFormat getTimeInstance()
This method is used to return the time formatter with the default formatting style for the default locale.
static final DateFormat getTimeInstance(int style)
This method is used to return the time formatter with the given formatting style for the default locale.
static final DateFormat getTimeInstance(int style, Locale locale)
This method is used to return the time formatter with the particular formatting style for the given locale.
static final DateFormat getDateInstance()
This method is used to return the date formatter with the default formatting style for the default locale.
static final DateFormat getDateInstance(int style)
This method is used to return the date formatter with the given formatting style for the default locale.
static final DateFormat getDateInstance(int style, Locale locale)
This method is used to return date formatter with the given formatting style for the given locale.
static final DateFormat getDateTimeInstance()
This method is used to return the date/time formatter with the default formatting style for the default locale.
static final DateFormat getDateTimeInstance(int dateStyle,int timeStyle)
This method is used to return the date/time formatter with the given date formatting style and time formatting style for the default locale.
static final DateFormat getDateTimeInstance(int dateStyle, int timeStyle, Locale locale)
This method is used to return the date/time formatter with the given date formatting style and time formatting style for the given locale.
static final DateFormat getInstance()
This method is used to return the date/time formatter with short formatting style for date and time.
static Locale[] getAvailableLocales()
This method is used to return an array of the available locales.
Calendar getCalendar()
This method is used to return an instance of the Calendar for this DateFormat instance.
NumberFormat getNumberFormat()
This method is used to return an instance of NumberFormat for this DateFormat instance.
TimeZone getTimeZone()
This method is used to return an instance of the TimeZone for this DateFormat instance.
Let’s see an example: Date to String, given below.
Code
- import java.text.DateFormat;
- import java.util.Date;
- public class DateExample {
- public static void main(String[] args) {
- Date d = new Date();
- System.out.println("Current Date: " + d);
- String s = DateFormat.getInstance().format(d);
- System.out.println("Date Format to String: " + s);
- }
- }
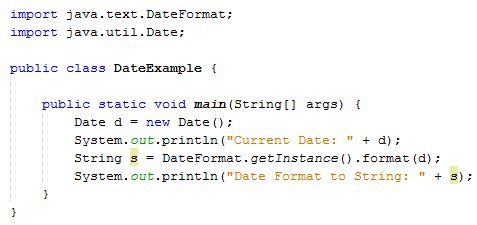
Output
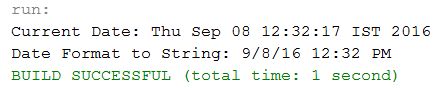
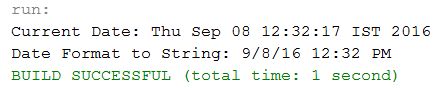
Let’s see another example: Date Format with Time, given below.
Code
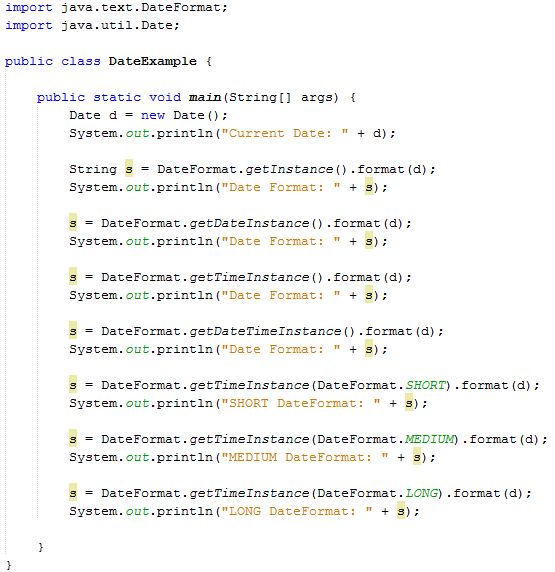
- import java.text.DateFormat;
- import java.util.Date;
- public class DateExample {
- public static void main(String[] args) {
- Date d = new Date();
- System.out.println("Current Date: " + d);
- String s = DateFormat.getInstance().format(d);
- System.out.println("Date Format: " + s);
- s = DateFormat.getDateInstance().format(d);
- System.out.println("Date Format: " + s);
- s = DateFormat.getTimeInstance().format(d);
- System.out.println("Date Format: " + s);
- s = DateFormat.getDateTimeInstance().format(d);
- System.out.println("Date Format: " + s);
- s = DateFormat.getTimeInstance(DateFormat.SHORT).format(d);
- System.out.println("SHORT DateFormat: " + s);
- s = DateFormat.getTimeInstance(DateFormat.MEDIUM).format(d);
- System.out.println("MEDIUM DateFormat: " + s);
- s = DateFormat.getTimeInstance(DateFormat.LONG).format(d);
- System.out.println("LONG DateFormat: " + s);
- }
- }
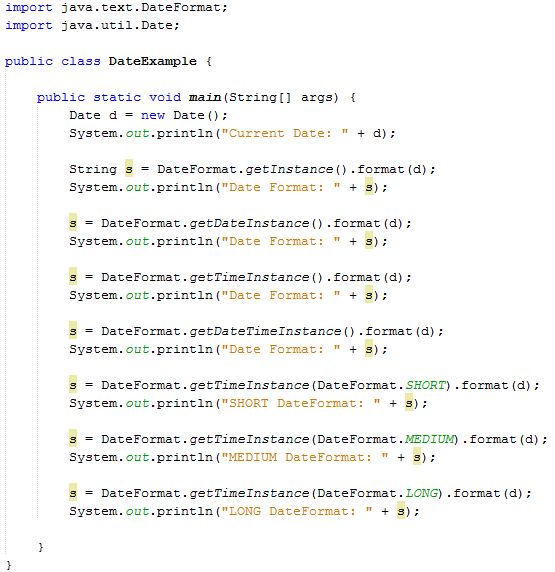
Output
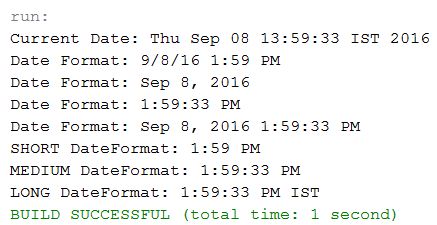
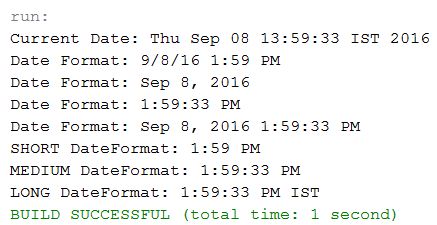
SimpleDateFormat
In Java, java.text.SimpleDateFormat class provides many methods to format and parse the date and time. It is a concrete class to format and parse the date, which extends java.text.DateFormat class.
Let’s see an example: Date to String, given below.
Code
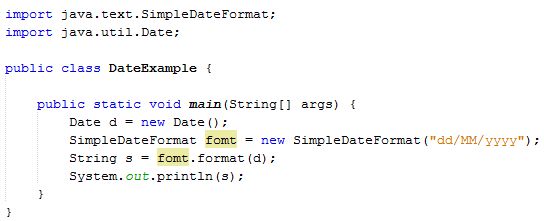
- import java.text.SimpleDateFormat;
- import java.util.Date;
- public class DateExample {
- public static void main(String[] args) {
- Date d = new Date();
- SimpleDateFormat fomt = new SimpleDateFormat("dd/MM/yyyy");
- String s = fomt.format(d);
- System.out.println(s);
- }
- }
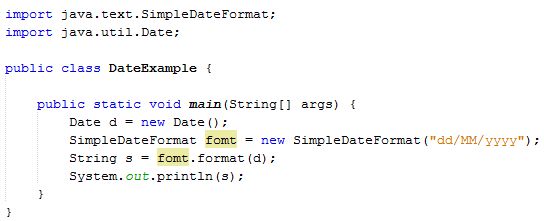
Output


In Java, capital M represents month and small m represents minute.
Let’s see another example: Date Format with Time, given below.
Code
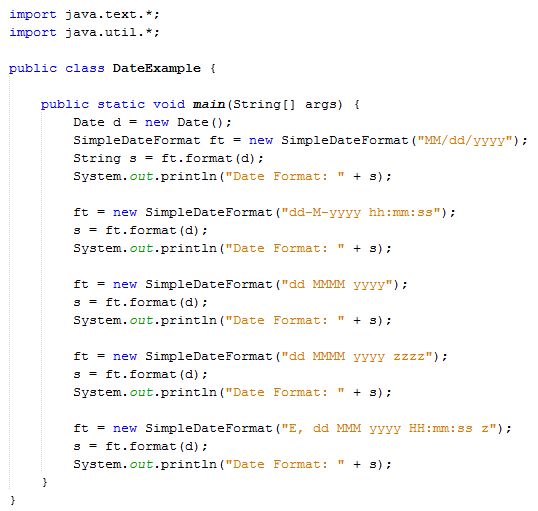
- import java.text.*;
- import java.util.*;
- public class DateExample {
- public static void main(String[] args) {
- Date d = new Date();
- SimpleDateFormat ft = new SimpleDateFormat("MM/dd/yyyy");
- String s = ft.format(d);
- System.out.println("Date Format: " + s);
- ft = new SimpleDateFormat("dd-M-yyyy hh:mm:ss");
- s = ft.format(d);
- System.out.println("Date Format: " + s);
- ft = new SimpleDateFormat("dd MMMM yyyy");
- s = ft.format(d);
- System.out.println("Date Format: " + s);
- ft = new SimpleDateFormat("dd MMMM yyyy zzzz");
- s = ft.format(d);
- System.out.println("Date Format: " + s);
- ft = new SimpleDateFormat("E, dd MMM yyyy HH:mm:ss z");
- s = ft.format(d);
- System.out.println("Date Format: " + s);
- }
- }
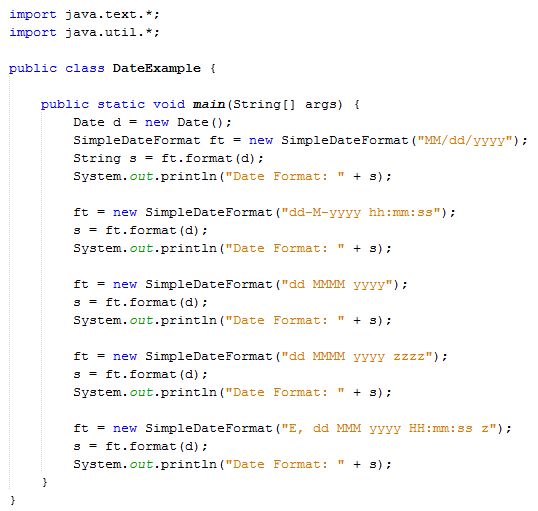
Output
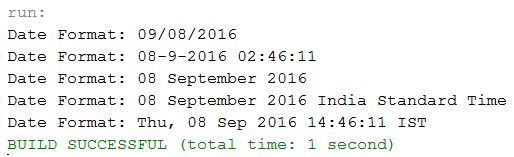
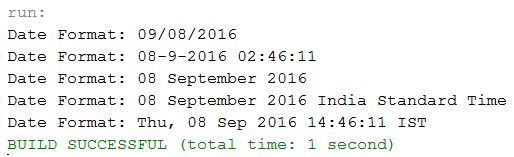
Now, let’s see an example: String to Date, given below.
Code
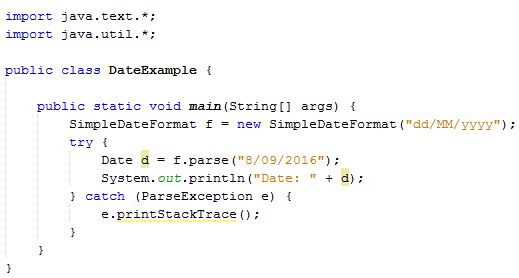
- import java.text.*;
- import java.util.*;
- public class DateExample {
- public static void main(String[] args) {
- SimpleDateFormat f = new SimpleDateFormat("dd/MM/yyyy");
- try {
- Date d = f.parse("8/09/2016");
- System.out.println("Date: " + d);
- } catch (ParseException e) {
- e.printStackTrace();
- }
- }
- }
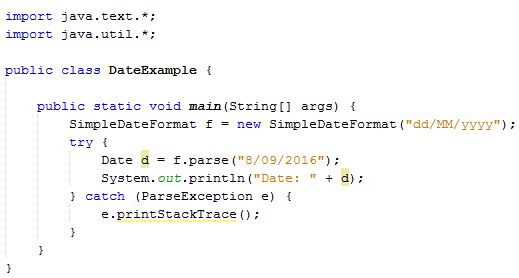
Output


Summary
Thus, we learnt, FormatDate and SimpleFormatDate classes to format the date and also learn, how we can change date format in Java.