How To Create A Program That Works As Javap Tool
Methods of java.lang.Class class
Some methods of java.lang.Class class are used to display the metadata of a class, which are,
public Field[] getDeclaredFields()throws SecurityException
This method is used to return an array of field objects, reflecting all the fields, created by the class and an interface represented by the class object.
public Constructor[] getDeclaredConstructors()throws SecurityException
This method is used to return an array of constructor objects, reflecting all the constructors, created by the class represented by the class object.
public Method[] getDeclaredMethods()throws SecurityException
This method is used to return an array of method objects, reflecting all the methods, created by the class and an interface, represented by the class object.
Let's create a program that works as javap tool, given below.
Code
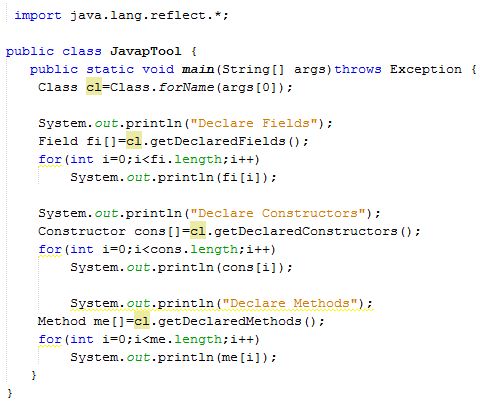
- import java.lang.reflect.*;
- public class JavapTool {
- public static void main(String[] args) throws Exception {
- Class cl = Class.forName(args[0]);
- System.out.println("Declare Fields");
- Field fi[] = cl.getDeclaredFields();
- for (int i = 0; i < fi.length; i++)
- System.out.println(fi[i]);
- System.out.println("Declare Constructors");
- Constructor cons[] = cl.getDeclaredConstructors();
- for (int i = 0; i < cons.length; i++)
- System.out.println(cons[i]);
- System.out.println("Declare Methods");
- Method me[] = cl.getDeclaredMethods();
- for (int i = 0; i < me.length; i++)
- System.out.println(me[i]);
- }
- }
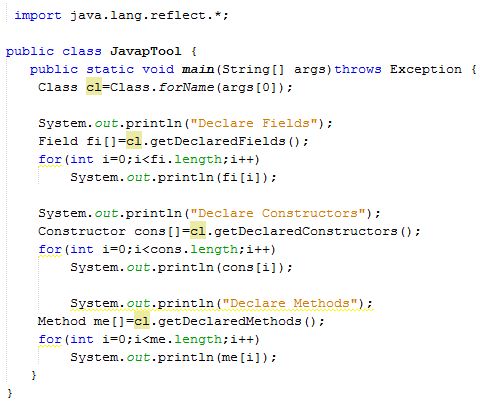
In the example, shown above, we can get the details of any class and it may be user-defined or pre-defined class at the runtime.
Summary
Thus, we learnt how we can create a program that works as javap tool in Java and also learnt that some important methods of java.lang.Class class in Java.